Animation#
You can use animation
class of matplotlib
to show animations.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import animation
After downloading the code, please uncomment the line %matplotlib notebook
to see animations.
%matplotlib inline
#%matplotlib notebook
FuncAnimation#
It is often helpful to visualize the data while they are computed, rather than after all computations are done.
For that, you can define a function to draw each frame, and pass the function to FuncAnimation
.
We will see defining your own function in Chapter 4, but here is an example.
def pendulum(i, clear=True, dt=0.05):
"""swinging pendulum. i:frame number"""
# clear previous plot
if clear:
plt.cla()
# swinging pendulum
l = 1 # length
th = np.sin(np.pi*i*dt) # angle, 2 sec period
x = np.sin(th)
y = -np.cos(th)
# draw a new plot
pl = plt.plot([0, x], [0, y], 'b')
plt.axis('square')
plt.xlim(-1.2*l, 1.2*l)
plt.ylim(-1.2*l, 1.2*l)
return pl # for saving
fig = plt.figure()
anim = animation.FuncAnimation(fig, pendulum, frames=40, interval=50)
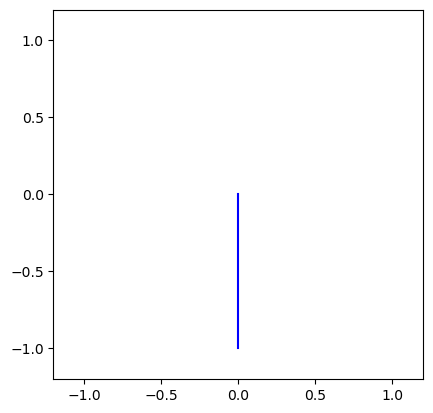
ArtistAnimation#
You can alternatively save all frames in an array and animate at once.
fig = plt.figure()
frames = [] # prepare frames
for i in range(40):
art = pendulum(i, False)
# append to the frame
frames.append(art)
anim = animation.ArtistAnimation(fig, frames, interval=50)
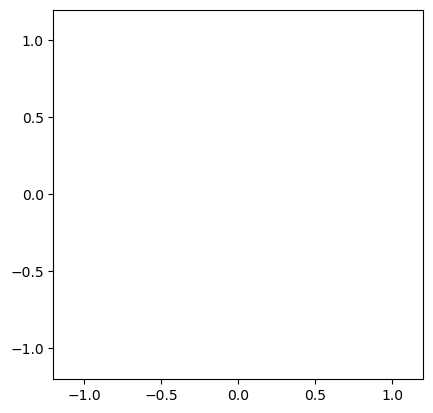
You can save the movie in a motion gif file.
anim.save("pend.gif", writer='pillow')
Here is the saved file pend.gif
: