9. Stochastic Methods#
Some computations involve random numbers, such as simulating stochastic processes or searching in a high dimension space.
References:
Christopher M. Bishop: Pattern Recognition and Machine Learning, Chapter 11: Sampling methods. Springer, 2006.
Jun S. Liu: Monte Carlo Strategies in Scientific Computing. Springer, 2004.
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
Random Numbers#
How can we generate random numbers from deterministic digital computers? They are, of course, pseudo random numbers.
The classic way of generating pseudo random numbers is Linear Congruent Method using a sequential dynimcs:
$\( x_{i+1}=ax_i+b \mod m. \)$
Below is what was long used in the Unix system.
def lcm(n=1, seed=-1726662223):
"""Random integers <2**31 by linear congruent method"""
a = 1103515245
b = 12345
c = 0x7fffffff # 2**31 - 1
x = np.zeros(n+1, dtype=int)
x[0] = seed
for i in range(n):
x[i+1] = (a*x[i] + b) & c # bit-wise and
return(x[1:])
x = lcm(1000)
plt.subplot(1, 3, (1,2))
plt.plot(x, '.')
plt.ylabel('x')
plt.subplot(1, 3, 3)
plt.hist(x, orientation='horizontal');
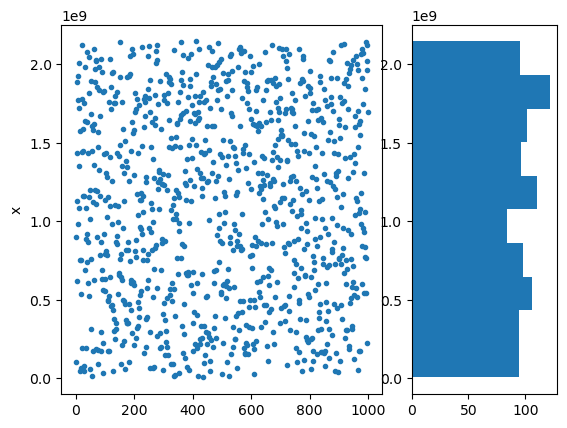
A common problem with LCM is that lower digits can fall in simple cycles.
x[:100]%4
array([2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3,
0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1,
2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3,
0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1,
2, 3, 0, 1, 2, 3, 0, 1, 2, 3, 0, 1])
Also the samples can form crystal-like structure when consecutive numbers are taken as a vector.
numpy.random
uses an advanced method called Mersenne Twister which overcomes these problems.
x = np.random.randint(2**31, size=1000)
plt.subplot(1, 3, (1,2))
plt.plot(x, '.')
plt.ylabel('x')
plt.subplot(1, 3, 3)
plt.hist(x, orientation='horizontal');
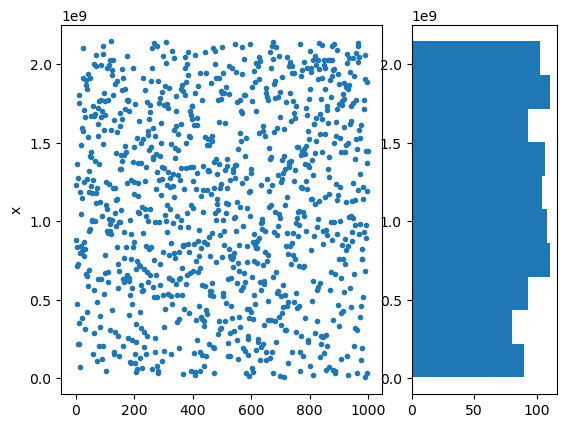
x[:100]%4
array([1, 0, 0, 2, 2, 1, 3, 1, 3, 2, 2, 1, 2, 1, 2, 0, 1, 3, 0, 0, 3, 0,
1, 1, 3, 1, 2, 1, 0, 2, 0, 3, 3, 3, 3, 2, 2, 2, 3, 3, 0, 3, 2, 1,
3, 3, 0, 0, 1, 1, 3, 0, 1, 1, 2, 0, 1, 1, 2, 0, 1, 0, 1, 3, 2, 0,
3, 3, 1, 3, 0, 3, 1, 0, 1, 2, 2, 3, 3, 2, 3, 0, 0, 0, 2, 0, 3, 2,
2, 2, 3, 3, 2, 3, 0, 2, 2, 2, 3, 3])
Integrating area/volume#
Uniform random numbers can be used to approximate integration $\( \int_V f(x)dx \simeq \frac{|V|}{n}\sum_{i=1}^n f(x_i) \)\( by uniform samples \)x_i\( in a volume \)V$
For example, let us evaluate a volume of a sphere.
# Sphere in 3D
def sphere(x):
"""height of a half sphere"""
h2 = 1 - x[0]**2 - x[1]**2
return np.sqrt((h2>0)*h2)
m = 10000
x = np.random.random((2, m)) # one quadrant
v = np.sum(sphere(x))/m
print(8*v)
print(4/3*np.pi)
4.206959704313234
4.1887902047863905
Let us evaluate the volume of n-dimension sphere, which is supposed to be \( \frac{\pi^{n/2}}{\Gamma(n/2-1)} \)
from scipy.special import gamma
# n-dimensional sphere
def nsphere(x):
"""height of a half sphere in n-dim"""
h2 = 1 - np.sum(x**2, axis=0)
return np.sqrt((h2>0)*h2)
m = 10000
for n in range(2,20):
x = np.random.random((n-1, m)) # one quadrant
v = np.sum(nsphere(x))/m
print(n, 2**n*v, np.pi**(n/2)/gamma(n/2+1))
2 3.1526499173370155 3.141592653589793
3 4.203831762692867 4.188790204786391
4 4.83270741655944 4.934802200544679
5 5.150269773222107 5.263789013914324
6 5.070027814534007 5.167712780049969
7 4.72773563599012 4.724765970331401
8 3.836871468206199 4.058712126416768
9 3.2305764107604706 3.2985089027387064
10 2.0934617588475652 2.550164039877345
11 2.8162671917424706 1.8841038793898999
12 0.8587133920913308 1.3352627688545893
13 1.5590615605111273 0.9106287547832829
14 1.486328320231397 0.5992645293207919
15 0.0 0.38144328082330436
16 0.0 0.23533063035889312
17 0.0 0.140981106917139
18 0.0 0.08214588661112819
19 0.0 0.04662160103008853
Non-uniform Distributions#
How can we generate samples following a non-uniform distribution \(p(x)\)?
If the cumulative density function \(f(x) = \int_{-\infty}^{x} p(u) du\) is known, we can map uniformly distributed samples \(y_i\in[0,1]\) to \(x_i = f^{-1}(y_i)\).
Exponential distribution#
# Exponential distribution in [0,infinity)
def p_exp(x, mu=1):
"""density function of exponential distribution"""
return np.exp(-x/mu)/mu
def f_exp(x, mu=1):
"""cumulative density function of exponential distribution"""
return 1 - np.exp(-x/mu)
def finv_exp(y, mu=1):
"""inverse of cumulative density function of exponential distribution"""
return -mu*np.log(1 - y)
x = np.linspace(0, 10, 100)
plt.plot(x, p_exp(x, 2))
plt.plot(x, f_exp(x, 2))
y = np.arange(0, 1, 0.01)
plt.plot(finv_exp(y, 2), y, ':')
plt.xlabel('x'); plt.ylabel('p, f');
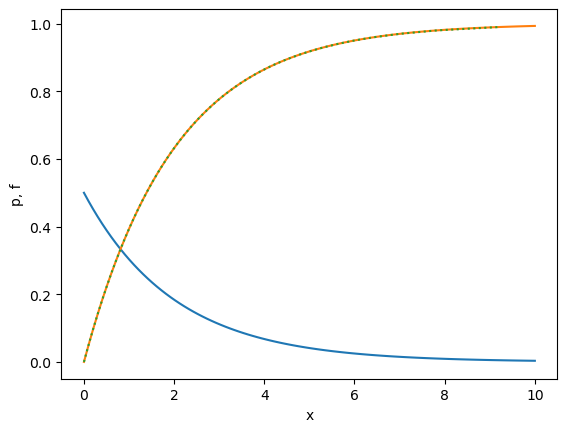
def x_exp(n=1, mu=1):
"""sample from exponential distribution"""
ys = np.random.random(n) # uniform in [0,1]
return finv_exp(ys, mu)
x_exp(10, 2)
array([0.20095515, 0.94894238, 9.55496086, 1.40499287, 0.58635524,
3.18393456, 1.07225255, 1.65301845, 1.02949799, 0.15646067])
xs = x_exp(1000, 4)
plt.hist(xs, bins=20, density=True)
plt.plot(x, p_exp(x, 4))
plt.xlabel('x'); plt.ylabel('p');
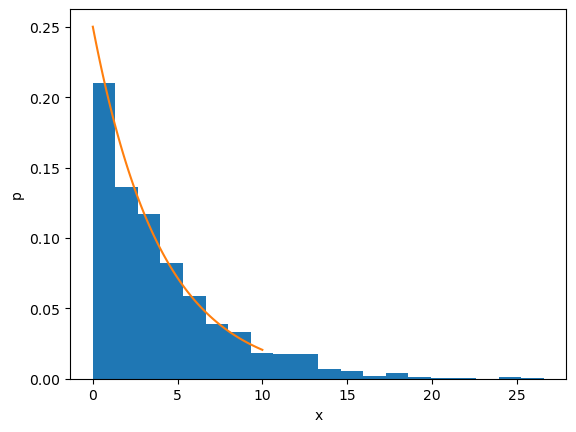
When a stochastic variable \(x\) following the distribution \(p(x)\) is transformed by \(y=g(x)\), the distribution of \(y\) is given by $\(p(y) = \left|\frac{\partial x}{\partial y}\right|p(x)\)\( where \)|,|$ means the absolute value for a scalar derivative and the determinant for a Jacobian matrix.
Normal distribution#
A common way of generating a normal (Gaussian) distribution $\( p(x) = \frac{1}{\sqrt{2\pi}}e^{-\frac{1}{2}x^2} \)\( is to consider a 2D normal distribution \)\( p(x_1,x_2) = p(x_1)p(x_2) = \frac{1}{2\pi}e^{-\frac{1}{2}(x_1^2+x_2^2)}. \)\( For a polar coordinate tranformation \)\( x_1 = r\cos\theta \)\( \)\( x_2 = r\sin\theta, \)\( the Jacobian is \)\( \frac{\partial (x_1,x_2)}{\partial (r,\theta)} = \left(\begin{array}{cc} \cos\theta & -r\sin\theta\\ \sin\theta & r\cos\theta\end{array}\right)\)\( and its determinant is \)\( \det\frac{\partial (x_1,x_2)}{\partial (r,\theta)} = r\cos^2\theta + r\sin^2\theta = r. \)\( Thus we have the relationship \)\( p(r,\theta) = r p(x_1,x_2) = \frac{r}{2\pi} e^{−\frac{r^2}{2}}. \)\( By further transforming \)u=r^2\(, from \)\frac{du}{dr}= 2r\(, we have \)\( p(u,\theta) = \frac{1}{2r}p(r,\theta) = \frac{1}{4\pi} e^{−\frac{u}{2}}.\)$
Thus we can sample \(u\) by exponential distribution \(p(u)=\frac{1}{2} e^{−\frac{u}{2}}\) and \(\theta\) by uniform distribution in \([0,2\pi)\), and then transform them to \(x_1\) and \(x_2\) to generate two samples following normal distribution.
This is known as Box-Muller method.
def box_muller(n=1):
"""Generate 2n gaussian samples"""
u = x_exp(n, 2)
r = np.sqrt(u)
theta = 2*np.pi*np.random.random(n)
x1 = r*np.cos(theta)
x2 = r*np.sin(theta)
return np.hstack((x1,x2))
box_muller(1)
array([ 0.95079908, -0.39543194])
xs = box_muller(1000)
plt.hist(xs, bins=20, density=True)
# check how the histogram fits the pdf
x = np.linspace(-5, 5, 100)
px = np.exp(-x**2/2)/np.sqrt(2*np.pi)
plt.plot(x, px)
plt.xlabel('x'); plt.ylabel('p');
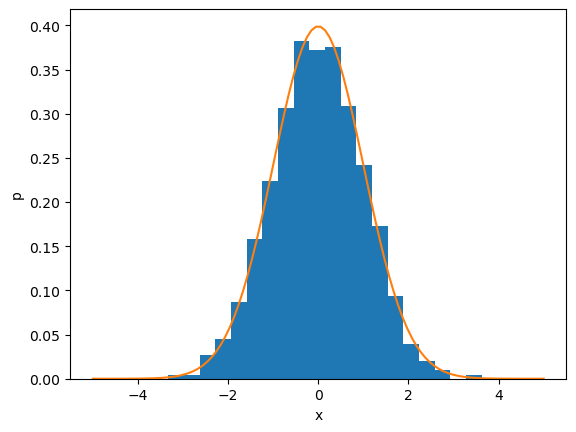
In practice, you can sample by the whole variety of distributions in numpy.random package, but it’s good to know the principle.
help(np.random)
Help on package numpy.random in numpy:
NAME
numpy.random
DESCRIPTION
========================
Random Number Generation
========================
Use ``default_rng()`` to create a `Generator` and call its methods.
=============== =========================================================
Generator
--------------- ---------------------------------------------------------
Generator Class implementing all of the random number distributions
default_rng Default constructor for ``Generator``
=============== =========================================================
============================================= ===
BitGenerator Streams that work with Generator
--------------------------------------------- ---
MT19937
PCG64
PCG64DXSM
Philox
SFC64
============================================= ===
============================================= ===
Getting entropy to initialize a BitGenerator
--------------------------------------------- ---
SeedSequence
============================================= ===
Legacy
------
For backwards compatibility with previous versions of numpy before 1.17, the
various aliases to the global `RandomState` methods are left alone and do not
use the new `Generator` API.
==================== =========================================================
Utility functions
-------------------- ---------------------------------------------------------
random Uniformly distributed floats over ``[0, 1)``
bytes Uniformly distributed random bytes.
permutation Randomly permute a sequence / generate a random sequence.
shuffle Randomly permute a sequence in place.
choice Random sample from 1-D array.
==================== =========================================================
==================== =========================================================
Compatibility
functions - removed
in the new API
-------------------- ---------------------------------------------------------
rand Uniformly distributed values.
randn Normally distributed values.
ranf Uniformly distributed floating point numbers.
random_integers Uniformly distributed integers in a given range.
(deprecated, use ``integers(..., closed=True)`` instead)
random_sample Alias for `random_sample`
randint Uniformly distributed integers in a given range
seed Seed the legacy random number generator.
==================== =========================================================
==================== =========================================================
Univariate
distributions
-------------------- ---------------------------------------------------------
beta Beta distribution over ``[0, 1]``.
binomial Binomial distribution.
chisquare :math:`\chi^2` distribution.
exponential Exponential distribution.
f F (Fisher-Snedecor) distribution.
gamma Gamma distribution.
geometric Geometric distribution.
gumbel Gumbel distribution.
hypergeometric Hypergeometric distribution.
laplace Laplace distribution.
logistic Logistic distribution.
lognormal Log-normal distribution.
logseries Logarithmic series distribution.
negative_binomial Negative binomial distribution.
noncentral_chisquare Non-central chi-square distribution.
noncentral_f Non-central F distribution.
normal Normal / Gaussian distribution.
pareto Pareto distribution.
poisson Poisson distribution.
power Power distribution.
rayleigh Rayleigh distribution.
triangular Triangular distribution.
uniform Uniform distribution.
vonmises Von Mises circular distribution.
wald Wald (inverse Gaussian) distribution.
weibull Weibull distribution.
zipf Zipf's distribution over ranked data.
==================== =========================================================
==================== ==========================================================
Multivariate
distributions
-------------------- ----------------------------------------------------------
dirichlet Multivariate generalization of Beta distribution.
multinomial Multivariate generalization of the binomial distribution.
multivariate_normal Multivariate generalization of the normal distribution.
==================== ==========================================================
==================== =========================================================
Standard
distributions
-------------------- ---------------------------------------------------------
standard_cauchy Standard Cauchy-Lorentz distribution.
standard_exponential Standard exponential distribution.
standard_gamma Standard Gamma distribution.
standard_normal Standard normal distribution.
standard_t Standard Student's t-distribution.
==================== =========================================================
==================== =========================================================
Internal functions
-------------------- ---------------------------------------------------------
get_state Get tuple representing internal state of generator.
set_state Set state of generator.
==================== =========================================================
PACKAGE CONTENTS
_bounded_integers
_common
_generator
_mt19937
_pcg64
_philox
_pickle
_sfc64
bit_generator
mtrand
tests (package)
CLASSES
builtins.object
numpy.random._generator.Generator
numpy.random.bit_generator.BitGenerator
numpy.random._mt19937.MT19937
numpy.random._pcg64.PCG64
numpy.random._pcg64.PCG64DXSM
numpy.random._philox.Philox
numpy.random._sfc64.SFC64
numpy.random.bit_generator.SeedSequence
numpy.random.mtrand.RandomState
class BitGenerator(builtins.object)
| BitGenerator(seed=None)
|
| Base Class for generic BitGenerators, which provide a stream
| of random bits based on different algorithms. Must be overridden.
|
| Parameters
| ----------
| seed : {None, int, array_like[ints], SeedSequence}, optional
| A seed to initialize the `BitGenerator`. If None, then fresh,
| unpredictable entropy will be pulled from the OS. If an ``int`` or
| ``array_like[ints]`` is passed, then it will be passed to
| `~numpy.random.SeedSequence` to derive the initial `BitGenerator` state.
| One may also pass in a `SeedSequence` instance.
| All integer values must be non-negative.
|
| Attributes
| ----------
| lock : threading.Lock
| Lock instance that is shared so that the same BitGenerator can
| be used in multiple Generators without corrupting the state. Code that
| generates values from a bit generator should hold the bit generator's
| lock.
|
| See Also
| --------
| SeedSequence
|
| Methods defined here:
|
| __getstate__(...)
| Helper for pickle.
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce__(...)
| Helper for pickle.
|
| __setstate__(...)
|
| random_raw(...)
| random_raw(self, size=None)
|
| Return randoms as generated by the underlying BitGenerator
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| output : bool, optional
| Output values. Used for performance testing since the generated
| values are not returned.
|
| Returns
| -------
| out : uint or ndarray
| Drawn samples.
|
| Notes
| -----
| This method directly exposes the raw underlying pseudo-random
| number generator. All values are returned as unsigned 64-bit
| values irrespective of the number of bits produced by the PRNG.
|
| See the class docstring for the number of bits returned.
|
| spawn(...)
| spawn(n_children)
|
| Create new independent child bit generators.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children. Some bit generators also implement ``jumped``
| as a different approach for creating independent streams.
|
| .. versionadded:: 1.25.0
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| child_bit_generators : list of BitGenerators
|
| Raises
| ------
| TypeError
| When the underlying SeedSequence does not implement spawning.
|
| See Also
| --------
| random.Generator.spawn, random.SeedSequence.spawn :
| Equivalent method on the generator and seed sequence.
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| capsule
|
| cffi
| CFFI interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing CFFI wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| ctypes
| ctypes interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing ctypes wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| lock
|
| seed_seq
| Get the seed sequence used to initialize the bit generator.
|
| .. versionadded:: 1.25.0
|
| Returns
| -------
| seed_seq : ISeedSequence
| The SeedSequence object used to initialize the BitGenerator.
| This is normally a `np.random.SeedSequence` instance.
|
| state
| Get or set the PRNG state
|
| The base BitGenerator.state must be overridden by a subclass
|
| Returns
| -------
| state : dict
| Dictionary containing the information required to describe the
| state of the PRNG
class Generator(builtins.object)
| Generator(bit_generator)
|
| Container for the BitGenerators.
|
| ``Generator`` exposes a number of methods for generating random
| numbers drawn from a variety of probability distributions. In addition to
| the distribution-specific arguments, each method takes a keyword argument
| `size` that defaults to ``None``. If `size` is ``None``, then a single
| value is generated and returned. If `size` is an integer, then a 1-D
| array filled with generated values is returned. If `size` is a tuple,
| then an array with that shape is filled and returned.
|
| The function :func:`numpy.random.default_rng` will instantiate
| a `Generator` with numpy's default `BitGenerator`.
|
| **No Compatibility Guarantee**
|
| ``Generator`` does not provide a version compatibility guarantee. In
| particular, as better algorithms evolve the bit stream may change.
|
| Parameters
| ----------
| bit_generator : BitGenerator
| BitGenerator to use as the core generator.
|
| Notes
| -----
| The Python stdlib module `random` contains pseudo-random number generator
| with a number of methods that are similar to the ones available in
| ``Generator``. It uses Mersenne Twister, and this bit generator can
| be accessed using ``MT19937``. ``Generator``, besides being
| NumPy-aware, has the advantage that it provides a much larger number
| of probability distributions to choose from.
|
| Examples
| --------
| >>> from numpy.random import Generator, PCG64
| >>> rng = Generator(PCG64())
| >>> rng.standard_normal()
| -0.203 # random
|
| See Also
| --------
| default_rng : Recommended constructor for `Generator`.
|
| Methods defined here:
|
| __getstate__(...)
| Helper for pickle.
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce__(...)
| Helper for pickle.
|
| __repr__(...)
| Return repr(self).
|
| __setstate__(...)
|
| __str__(self, /)
| Return str(self).
|
| beta(...)
| beta(a, b, size=None)
|
| Draw samples from a Beta distribution.
|
| The Beta distribution is a special case of the Dirichlet distribution,
| and is related to the Gamma distribution. It has the probability
| distribution function
|
| .. math:: f(x; a,b) = \frac{1}{B(\alpha, \beta)} x^{\alpha - 1}
| (1 - x)^{\beta - 1},
|
| where the normalization, B, is the beta function,
|
| .. math:: B(\alpha, \beta) = \int_0^1 t^{\alpha - 1}
| (1 - t)^{\beta - 1} dt.
|
| It is often seen in Bayesian inference and order statistics.
|
| Parameters
| ----------
| a : float or array_like of floats
| Alpha, positive (>0).
| b : float or array_like of floats
| Beta, positive (>0).
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` and ``b`` are both scalars.
| Otherwise, ``np.broadcast(a, b).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized beta distribution.
|
| binomial(...)
| binomial(n, p, size=None)
|
| Draw samples from a binomial distribution.
|
| Samples are drawn from a binomial distribution with specified
| parameters, n trials and p probability of success where
| n an integer >= 0 and p is in the interval [0,1]. (n may be
| input as a float, but it is truncated to an integer in use)
|
| Parameters
| ----------
| n : int or array_like of ints
| Parameter of the distribution, >= 0. Floats are also accepted,
| but they will be truncated to integers.
| p : float or array_like of floats
| Parameter of the distribution, >= 0 and <=1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``n`` and ``p`` are both scalars.
| Otherwise, ``np.broadcast(n, p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized binomial distribution, where
| each sample is equal to the number of successes over the n trials.
|
| See Also
| --------
| scipy.stats.binom : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the binomial distribution is
|
| .. math:: P(N) = \binom{n}{N}p^N(1-p)^{n-N},
|
| where :math:`n` is the number of trials, :math:`p` is the probability
| of success, and :math:`N` is the number of successes.
|
| When estimating the standard error of a proportion in a population by
| using a random sample, the normal distribution works well unless the
| product p*n <=5, where p = population proportion estimate, and n =
| number of samples, in which case the binomial distribution is used
| instead. For example, a sample of 15 people shows 4 who are left
| handed, and 11 who are right handed. Then p = 4/15 = 27%. 0.27*15 = 4,
| so the binomial distribution should be used in this case.
|
| References
| ----------
| .. [1] Dalgaard, Peter, "Introductory Statistics with R",
| Springer-Verlag, 2002.
| .. [2] Glantz, Stanton A. "Primer of Biostatistics.", McGraw-Hill,
| Fifth Edition, 2002.
| .. [3] Lentner, Marvin, "Elementary Applied Statistics", Bogden
| and Quigley, 1972.
| .. [4] Weisstein, Eric W. "Binomial Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/BinomialDistribution.html
| .. [5] Wikipedia, "Binomial distribution",
| https://en.wikipedia.org/wiki/Binomial_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> rng = np.random.default_rng()
| >>> n, p = 10, .5 # number of trials, probability of each trial
| >>> s = rng.binomial(n, p, 1000)
| # result of flipping a coin 10 times, tested 1000 times.
|
| A real world example. A company drills 9 wild-cat oil exploration
| wells, each with an estimated probability of success of 0.1. All nine
| wells fail. What is the probability of that happening?
|
| Let's do 20,000 trials of the model, and count the number that
| generate zero positive results.
|
| >>> sum(rng.binomial(9, 0.1, 20000) == 0)/20000.
| # answer = 0.38885, or 39%.
|
| bytes(...)
| bytes(length)
|
| Return random bytes.
|
| Parameters
| ----------
| length : int
| Number of random bytes.
|
| Returns
| -------
| out : bytes
| String of length `length`.
|
| Examples
| --------
| >>> np.random.default_rng().bytes(10)
| b'\xfeC\x9b\x86\x17\xf2\xa1\xafcp' # random
|
| chisquare(...)
| chisquare(df, size=None)
|
| Draw samples from a chi-square distribution.
|
| When `df` independent random variables, each with standard normal
| distributions (mean 0, variance 1), are squared and summed, the
| resulting distribution is chi-square (see Notes). This distribution
| is often used in hypothesis testing.
|
| Parameters
| ----------
| df : float or array_like of floats
| Number of degrees of freedom, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``df`` is a scalar. Otherwise,
| ``np.array(df).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized chi-square distribution.
|
| Raises
| ------
| ValueError
| When `df` <= 0 or when an inappropriate `size` (e.g. ``size=-1``)
| is given.
|
| Notes
| -----
| The variable obtained by summing the squares of `df` independent,
| standard normally distributed random variables:
|
| .. math:: Q = \sum_{i=0}^{\mathtt{df}} X^2_i
|
| is chi-square distributed, denoted
|
| .. math:: Q \sim \chi^2_k.
|
| The probability density function of the chi-squared distribution is
|
| .. math:: p(x) = \frac{(1/2)^{k/2}}{\Gamma(k/2)}
| x^{k/2 - 1} e^{-x/2},
|
| where :math:`\Gamma` is the gamma function,
|
| .. math:: \Gamma(x) = \int_0^{-\infty} t^{x - 1} e^{-t} dt.
|
| References
| ----------
| .. [1] NIST "Engineering Statistics Handbook"
| https://www.itl.nist.gov/div898/handbook/eda/section3/eda3666.htm
|
| Examples
| --------
| >>> np.random.default_rng().chisquare(2,4)
| array([ 1.89920014, 9.00867716, 3.13710533, 5.62318272]) # random
|
| choice(...)
| choice(a, size=None, replace=True, p=None, axis=0, shuffle=True)
|
| Generates a random sample from a given array
|
| Parameters
| ----------
| a : {array_like, int}
| If an ndarray, a random sample is generated from its elements.
| If an int, the random sample is generated from np.arange(a).
| size : {int, tuple[int]}, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn from the 1-d `a`. If `a` has more
| than one dimension, the `size` shape will be inserted into the
| `axis` dimension, so the output ``ndim`` will be ``a.ndim - 1 +
| len(size)``. Default is None, in which case a single value is
| returned.
| replace : bool, optional
| Whether the sample is with or without replacement. Default is True,
| meaning that a value of ``a`` can be selected multiple times.
| p : 1-D array_like, optional
| The probabilities associated with each entry in a.
| If not given, the sample assumes a uniform distribution over all
| entries in ``a``.
| axis : int, optional
| The axis along which the selection is performed. The default, 0,
| selects by row.
| shuffle : bool, optional
| Whether the sample is shuffled when sampling without replacement.
| Default is True, False provides a speedup.
|
| Returns
| -------
| samples : single item or ndarray
| The generated random samples
|
| Raises
| ------
| ValueError
| If a is an int and less than zero, if p is not 1-dimensional, if
| a is array-like with a size 0, if p is not a vector of
| probabilities, if a and p have different lengths, or if
| replace=False and the sample size is greater than the population
| size.
|
| See Also
| --------
| integers, shuffle, permutation
|
| Notes
| -----
| Setting user-specified probabilities through ``p`` uses a more general but less
| efficient sampler than the default. The general sampler produces a different sample
| than the optimized sampler even if each element of ``p`` is 1 / len(a).
|
| Examples
| --------
| Generate a uniform random sample from np.arange(5) of size 3:
|
| >>> rng = np.random.default_rng()
| >>> rng.choice(5, 3)
| array([0, 3, 4]) # random
| >>> #This is equivalent to rng.integers(0,5,3)
|
| Generate a non-uniform random sample from np.arange(5) of size 3:
|
| >>> rng.choice(5, 3, p=[0.1, 0, 0.3, 0.6, 0])
| array([3, 3, 0]) # random
|
| Generate a uniform random sample from np.arange(5) of size 3 without
| replacement:
|
| >>> rng.choice(5, 3, replace=False)
| array([3,1,0]) # random
| >>> #This is equivalent to rng.permutation(np.arange(5))[:3]
|
| Generate a uniform random sample from a 2-D array along the first
| axis (the default), without replacement:
|
| >>> rng.choice([[0, 1, 2], [3, 4, 5], [6, 7, 8]], 2, replace=False)
| array([[3, 4, 5], # random
| [0, 1, 2]])
|
| Generate a non-uniform random sample from np.arange(5) of size
| 3 without replacement:
|
| >>> rng.choice(5, 3, replace=False, p=[0.1, 0, 0.3, 0.6, 0])
| array([2, 3, 0]) # random
|
| Any of the above can be repeated with an arbitrary array-like
| instead of just integers. For instance:
|
| >>> aa_milne_arr = ['pooh', 'rabbit', 'piglet', 'Christopher']
| >>> rng.choice(aa_milne_arr, 5, p=[0.5, 0.1, 0.1, 0.3])
| array(['pooh', 'pooh', 'pooh', 'Christopher', 'piglet'], # random
| dtype='<U11')
|
| dirichlet(...)
| dirichlet(alpha, size=None)
|
| Draw samples from the Dirichlet distribution.
|
| Draw `size` samples of dimension k from a Dirichlet distribution. A
| Dirichlet-distributed random variable can be seen as a multivariate
| generalization of a Beta distribution. The Dirichlet distribution
| is a conjugate prior of a multinomial distribution in Bayesian
| inference.
|
| Parameters
| ----------
| alpha : sequence of floats, length k
| Parameter of the distribution (length ``k`` for sample of
| length ``k``).
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| vector of length ``k`` is returned.
|
| Returns
| -------
| samples : ndarray,
| The drawn samples, of shape ``(size, k)``.
|
| Raises
| ------
| ValueError
| If any value in ``alpha`` is less than zero
|
| Notes
| -----
| The Dirichlet distribution is a distribution over vectors
| :math:`x` that fulfil the conditions :math:`x_i>0` and
| :math:`\sum_{i=1}^k x_i = 1`.
|
| The probability density function :math:`p` of a
| Dirichlet-distributed random vector :math:`X` is
| proportional to
|
| .. math:: p(x) \propto \prod_{i=1}^{k}{x^{\alpha_i-1}_i},
|
| where :math:`\alpha` is a vector containing the positive
| concentration parameters.
|
| The method uses the following property for computation: let :math:`Y`
| be a random vector which has components that follow a standard gamma
| distribution, then :math:`X = \frac{1}{\sum_{i=1}^k{Y_i}} Y`
| is Dirichlet-distributed
|
| References
| ----------
| .. [1] David McKay, "Information Theory, Inference and Learning
| Algorithms," chapter 23,
| http://www.inference.org.uk/mackay/itila/
| .. [2] Wikipedia, "Dirichlet distribution",
| https://en.wikipedia.org/wiki/Dirichlet_distribution
|
| Examples
| --------
| Taking an example cited in Wikipedia, this distribution can be used if
| one wanted to cut strings (each of initial length 1.0) into K pieces
| with different lengths, where each piece had, on average, a designated
| average length, but allowing some variation in the relative sizes of
| the pieces.
|
| >>> s = np.random.default_rng().dirichlet((10, 5, 3), 20).transpose()
|
| >>> import matplotlib.pyplot as plt
| >>> plt.barh(range(20), s[0])
| >>> plt.barh(range(20), s[1], left=s[0], color='g')
| >>> plt.barh(range(20), s[2], left=s[0]+s[1], color='r')
| >>> plt.title("Lengths of Strings")
|
| exponential(...)
| exponential(scale=1.0, size=None)
|
| Draw samples from an exponential distribution.
|
| Its probability density function is
|
| .. math:: f(x; \frac{1}{\beta}) = \frac{1}{\beta} \exp(-\frac{x}{\beta}),
|
| for ``x > 0`` and 0 elsewhere. :math:`\beta` is the scale parameter,
| which is the inverse of the rate parameter :math:`\lambda = 1/\beta`.
| The rate parameter is an alternative, widely used parameterization
| of the exponential distribution [3]_.
|
| The exponential distribution is a continuous analogue of the
| geometric distribution. It describes many common situations, such as
| the size of raindrops measured over many rainstorms [1]_, or the time
| between page requests to Wikipedia [2]_.
|
| Parameters
| ----------
| scale : float or array_like of floats
| The scale parameter, :math:`\beta = 1/\lambda`. Must be
| non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``scale`` is a scalar. Otherwise,
| ``np.array(scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized exponential distribution.
|
| Examples
| --------
| A real world example: Assume a company has 10000 customer support
| agents and the average time between customer calls is 4 minutes.
|
| >>> n = 10000
| >>> time_between_calls = np.random.default_rng().exponential(scale=4, size=n)
|
| What is the probability that a customer will call in the next
| 4 to 5 minutes?
|
| >>> x = ((time_between_calls < 5).sum())/n
| >>> y = ((time_between_calls < 4).sum())/n
| >>> x-y
| 0.08 # may vary
|
| References
| ----------
| .. [1] Peyton Z. Peebles Jr., "Probability, Random Variables and
| Random Signal Principles", 4th ed, 2001, p. 57.
| .. [2] Wikipedia, "Poisson process",
| https://en.wikipedia.org/wiki/Poisson_process
| .. [3] Wikipedia, "Exponential distribution",
| https://en.wikipedia.org/wiki/Exponential_distribution
|
| f(...)
| f(dfnum, dfden, size=None)
|
| Draw samples from an F distribution.
|
| Samples are drawn from an F distribution with specified parameters,
| `dfnum` (degrees of freedom in numerator) and `dfden` (degrees of
| freedom in denominator), where both parameters must be greater than
| zero.
|
| The random variate of the F distribution (also known as the
| Fisher distribution) is a continuous probability distribution
| that arises in ANOVA tests, and is the ratio of two chi-square
| variates.
|
| Parameters
| ----------
| dfnum : float or array_like of floats
| Degrees of freedom in numerator, must be > 0.
| dfden : float or array_like of float
| Degrees of freedom in denominator, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``dfnum`` and ``dfden`` are both scalars.
| Otherwise, ``np.broadcast(dfnum, dfden).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Fisher distribution.
|
| See Also
| --------
| scipy.stats.f : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The F statistic is used to compare in-group variances to between-group
| variances. Calculating the distribution depends on the sampling, and
| so it is a function of the respective degrees of freedom in the
| problem. The variable `dfnum` is the number of samples minus one, the
| between-groups degrees of freedom, while `dfden` is the within-groups
| degrees of freedom, the sum of the number of samples in each group
| minus the number of groups.
|
| References
| ----------
| .. [1] Glantz, Stanton A. "Primer of Biostatistics.", McGraw-Hill,
| Fifth Edition, 2002.
| .. [2] Wikipedia, "F-distribution",
| https://en.wikipedia.org/wiki/F-distribution
|
| Examples
| --------
| An example from Glantz[1], pp 47-40:
|
| Two groups, children of diabetics (25 people) and children from people
| without diabetes (25 controls). Fasting blood glucose was measured,
| case group had a mean value of 86.1, controls had a mean value of
| 82.2. Standard deviations were 2.09 and 2.49 respectively. Are these
| data consistent with the null hypothesis that the parents diabetic
| status does not affect their children's blood glucose levels?
| Calculating the F statistic from the data gives a value of 36.01.
|
| Draw samples from the distribution:
|
| >>> dfnum = 1. # between group degrees of freedom
| >>> dfden = 48. # within groups degrees of freedom
| >>> s = np.random.default_rng().f(dfnum, dfden, 1000)
|
| The lower bound for the top 1% of the samples is :
|
| >>> np.sort(s)[-10]
| 7.61988120985 # random
|
| So there is about a 1% chance that the F statistic will exceed 7.62,
| the measured value is 36, so the null hypothesis is rejected at the 1%
| level.
|
| gamma(...)
| gamma(shape, scale=1.0, size=None)
|
| Draw samples from a Gamma distribution.
|
| Samples are drawn from a Gamma distribution with specified parameters,
| `shape` (sometimes designated "k") and `scale` (sometimes designated
| "theta"), where both parameters are > 0.
|
| Parameters
| ----------
| shape : float or array_like of floats
| The shape of the gamma distribution. Must be non-negative.
| scale : float or array_like of floats, optional
| The scale of the gamma distribution. Must be non-negative.
| Default is equal to 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``shape`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(shape, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized gamma distribution.
|
| See Also
| --------
| scipy.stats.gamma : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the Gamma distribution is
|
| .. math:: p(x) = x^{k-1}\frac{e^{-x/\theta}}{\theta^k\Gamma(k)},
|
| where :math:`k` is the shape and :math:`\theta` the scale,
| and :math:`\Gamma` is the Gamma function.
|
| The Gamma distribution is often used to model the times to failure of
| electronic components, and arises naturally in processes for which the
| waiting times between Poisson distributed events are relevant.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Gamma Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/GammaDistribution.html
| .. [2] Wikipedia, "Gamma distribution",
| https://en.wikipedia.org/wiki/Gamma_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> shape, scale = 2., 2. # mean=4, std=2*sqrt(2)
| >>> s = np.random.default_rng().gamma(shape, scale, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> import scipy.special as sps # doctest: +SKIP
| >>> count, bins, ignored = plt.hist(s, 50, density=True)
| >>> y = bins**(shape-1)*(np.exp(-bins/scale) / # doctest: +SKIP
| ... (sps.gamma(shape)*scale**shape))
| >>> plt.plot(bins, y, linewidth=2, color='r') # doctest: +SKIP
| >>> plt.show()
|
| geometric(...)
| geometric(p, size=None)
|
| Draw samples from the geometric distribution.
|
| Bernoulli trials are experiments with one of two outcomes:
| success or failure (an example of such an experiment is flipping
| a coin). The geometric distribution models the number of trials
| that must be run in order to achieve success. It is therefore
| supported on the positive integers, ``k = 1, 2, ...``.
|
| The probability mass function of the geometric distribution is
|
| .. math:: f(k) = (1 - p)^{k - 1} p
|
| where `p` is the probability of success of an individual trial.
|
| Parameters
| ----------
| p : float or array_like of floats
| The probability of success of an individual trial.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``p`` is a scalar. Otherwise,
| ``np.array(p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized geometric distribution.
|
| Examples
| --------
| Draw ten thousand values from the geometric distribution,
| with the probability of an individual success equal to 0.35:
|
| >>> z = np.random.default_rng().geometric(p=0.35, size=10000)
|
| How many trials succeeded after a single run?
|
| >>> (z == 1).sum() / 10000.
| 0.34889999999999999 # random
|
| gumbel(...)
| gumbel(loc=0.0, scale=1.0, size=None)
|
| Draw samples from a Gumbel distribution.
|
| Draw samples from a Gumbel distribution with specified location and
| scale. For more information on the Gumbel distribution, see
| Notes and References below.
|
| Parameters
| ----------
| loc : float or array_like of floats, optional
| The location of the mode of the distribution. Default is 0.
| scale : float or array_like of floats, optional
| The scale parameter of the distribution. Default is 1. Must be non-
| negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Gumbel distribution.
|
| See Also
| --------
| scipy.stats.gumbel_l
| scipy.stats.gumbel_r
| scipy.stats.genextreme
| weibull
|
| Notes
| -----
| The Gumbel (or Smallest Extreme Value (SEV) or the Smallest Extreme
| Value Type I) distribution is one of a class of Generalized Extreme
| Value (GEV) distributions used in modeling extreme value problems.
| The Gumbel is a special case of the Extreme Value Type I distribution
| for maximums from distributions with "exponential-like" tails.
|
| The probability density for the Gumbel distribution is
|
| .. math:: p(x) = \frac{e^{-(x - \mu)/ \beta}}{\beta} e^{ -e^{-(x - \mu)/
| \beta}},
|
| where :math:`\mu` is the mode, a location parameter, and
| :math:`\beta` is the scale parameter.
|
| The Gumbel (named for German mathematician Emil Julius Gumbel) was used
| very early in the hydrology literature, for modeling the occurrence of
| flood events. It is also used for modeling maximum wind speed and
| rainfall rates. It is a "fat-tailed" distribution - the probability of
| an event in the tail of the distribution is larger than if one used a
| Gaussian, hence the surprisingly frequent occurrence of 100-year
| floods. Floods were initially modeled as a Gaussian process, which
| underestimated the frequency of extreme events.
|
| It is one of a class of extreme value distributions, the Generalized
| Extreme Value (GEV) distributions, which also includes the Weibull and
| Frechet.
|
| The function has a mean of :math:`\mu + 0.57721\beta` and a variance
| of :math:`\frac{\pi^2}{6}\beta^2`.
|
| References
| ----------
| .. [1] Gumbel, E. J., "Statistics of Extremes,"
| New York: Columbia University Press, 1958.
| .. [2] Reiss, R.-D. and Thomas, M., "Statistical Analysis of Extreme
| Values from Insurance, Finance, Hydrology and Other Fields,"
| Basel: Birkhauser Verlag, 2001.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> rng = np.random.default_rng()
| >>> mu, beta = 0, 0.1 # location and scale
| >>> s = rng.gumbel(mu, beta, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 30, density=True)
| >>> plt.plot(bins, (1/beta)*np.exp(-(bins - mu)/beta)
| ... * np.exp( -np.exp( -(bins - mu) /beta) ),
| ... linewidth=2, color='r')
| >>> plt.show()
|
| Show how an extreme value distribution can arise from a Gaussian process
| and compare to a Gaussian:
|
| >>> means = []
| >>> maxima = []
| >>> for i in range(0,1000) :
| ... a = rng.normal(mu, beta, 1000)
| ... means.append(a.mean())
| ... maxima.append(a.max())
| >>> count, bins, ignored = plt.hist(maxima, 30, density=True)
| >>> beta = np.std(maxima) * np.sqrt(6) / np.pi
| >>> mu = np.mean(maxima) - 0.57721*beta
| >>> plt.plot(bins, (1/beta)*np.exp(-(bins - mu)/beta)
| ... * np.exp(-np.exp(-(bins - mu)/beta)),
| ... linewidth=2, color='r')
| >>> plt.plot(bins, 1/(beta * np.sqrt(2 * np.pi))
| ... * np.exp(-(bins - mu)**2 / (2 * beta**2)),
| ... linewidth=2, color='g')
| >>> plt.show()
|
| hypergeometric(...)
| hypergeometric(ngood, nbad, nsample, size=None)
|
| Draw samples from a Hypergeometric distribution.
|
| Samples are drawn from a hypergeometric distribution with specified
| parameters, `ngood` (ways to make a good selection), `nbad` (ways to make
| a bad selection), and `nsample` (number of items sampled, which is less
| than or equal to the sum ``ngood + nbad``).
|
| Parameters
| ----------
| ngood : int or array_like of ints
| Number of ways to make a good selection. Must be nonnegative and
| less than 10**9.
| nbad : int or array_like of ints
| Number of ways to make a bad selection. Must be nonnegative and
| less than 10**9.
| nsample : int or array_like of ints
| Number of items sampled. Must be nonnegative and less than
| ``ngood + nbad``.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if `ngood`, `nbad`, and `nsample`
| are all scalars. Otherwise, ``np.broadcast(ngood, nbad, nsample).size``
| samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized hypergeometric distribution. Each
| sample is the number of good items within a randomly selected subset of
| size `nsample` taken from a set of `ngood` good items and `nbad` bad items.
|
| See Also
| --------
| multivariate_hypergeometric : Draw samples from the multivariate
| hypergeometric distribution.
| scipy.stats.hypergeom : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the Hypergeometric distribution is
|
| .. math:: P(x) = \frac{\binom{g}{x}\binom{b}{n-x}}{\binom{g+b}{n}},
|
| where :math:`0 \le x \le n` and :math:`n-b \le x \le g`
|
| for P(x) the probability of ``x`` good results in the drawn sample,
| g = `ngood`, b = `nbad`, and n = `nsample`.
|
| Consider an urn with black and white marbles in it, `ngood` of them
| are black and `nbad` are white. If you draw `nsample` balls without
| replacement, then the hypergeometric distribution describes the
| distribution of black balls in the drawn sample.
|
| Note that this distribution is very similar to the binomial
| distribution, except that in this case, samples are drawn without
| replacement, whereas in the Binomial case samples are drawn with
| replacement (or the sample space is infinite). As the sample space
| becomes large, this distribution approaches the binomial.
|
| The arguments `ngood` and `nbad` each must be less than `10**9`. For
| extremely large arguments, the algorithm that is used to compute the
| samples [4]_ breaks down because of loss of precision in floating point
| calculations. For such large values, if `nsample` is not also large,
| the distribution can be approximated with the binomial distribution,
| `binomial(n=nsample, p=ngood/(ngood + nbad))`.
|
| References
| ----------
| .. [1] Lentner, Marvin, "Elementary Applied Statistics", Bogden
| and Quigley, 1972.
| .. [2] Weisstein, Eric W. "Hypergeometric Distribution." From
| MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/HypergeometricDistribution.html
| .. [3] Wikipedia, "Hypergeometric distribution",
| https://en.wikipedia.org/wiki/Hypergeometric_distribution
| .. [4] Stadlober, Ernst, "The ratio of uniforms approach for generating
| discrete random variates", Journal of Computational and Applied
| Mathematics, 31, pp. 181-189 (1990).
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> rng = np.random.default_rng()
| >>> ngood, nbad, nsamp = 100, 2, 10
| # number of good, number of bad, and number of samples
| >>> s = rng.hypergeometric(ngood, nbad, nsamp, 1000)
| >>> from matplotlib.pyplot import hist
| >>> hist(s)
| # note that it is very unlikely to grab both bad items
|
| Suppose you have an urn with 15 white and 15 black marbles.
| If you pull 15 marbles at random, how likely is it that
| 12 or more of them are one color?
|
| >>> s = rng.hypergeometric(15, 15, 15, 100000)
| >>> sum(s>=12)/100000. + sum(s<=3)/100000.
| # answer = 0.003 ... pretty unlikely!
|
| integers(...)
| integers(low, high=None, size=None, dtype=np.int64, endpoint=False)
|
| Return random integers from `low` (inclusive) to `high` (exclusive), or
| if endpoint=True, `low` (inclusive) to `high` (inclusive). Replaces
| `RandomState.randint` (with endpoint=False) and
| `RandomState.random_integers` (with endpoint=True)
|
| Return random integers from the "discrete uniform" distribution of
| the specified dtype. If `high` is None (the default), then results are
| from 0 to `low`.
|
| Parameters
| ----------
| low : int or array-like of ints
| Lowest (signed) integers to be drawn from the distribution (unless
| ``high=None``, in which case this parameter is 0 and this value is
| used for `high`).
| high : int or array-like of ints, optional
| If provided, one above the largest (signed) integer to be drawn
| from the distribution (see above for behavior if ``high=None``).
| If array-like, must contain integer values
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| dtype : dtype, optional
| Desired dtype of the result. Byteorder must be native.
| The default value is np.int64.
| endpoint : bool, optional
| If true, sample from the interval [low, high] instead of the
| default [low, high)
| Defaults to False
|
| Returns
| -------
| out : int or ndarray of ints
| `size`-shaped array of random integers from the appropriate
| distribution, or a single such random int if `size` not provided.
|
| Notes
| -----
| When using broadcasting with uint64 dtypes, the maximum value (2**64)
| cannot be represented as a standard integer type. The high array (or
| low if high is None) must have object dtype, e.g., array([2**64]).
|
| Examples
| --------
| >>> rng = np.random.default_rng()
| >>> rng.integers(2, size=10)
| array([1, 0, 0, 0, 1, 1, 0, 0, 1, 0]) # random
| >>> rng.integers(1, size=10)
| array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0])
|
| Generate a 2 x 4 array of ints between 0 and 4, inclusive:
|
| >>> rng.integers(5, size=(2, 4))
| array([[4, 0, 2, 1],
| [3, 2, 2, 0]]) # random
|
| Generate a 1 x 3 array with 3 different upper bounds
|
| >>> rng.integers(1, [3, 5, 10])
| array([2, 2, 9]) # random
|
| Generate a 1 by 3 array with 3 different lower bounds
|
| >>> rng.integers([1, 5, 7], 10)
| array([9, 8, 7]) # random
|
| Generate a 2 by 4 array using broadcasting with dtype of uint8
|
| >>> rng.integers([1, 3, 5, 7], [[10], [20]], dtype=np.uint8)
| array([[ 8, 6, 9, 7],
| [ 1, 16, 9, 12]], dtype=uint8) # random
|
| References
| ----------
| .. [1] Daniel Lemire., "Fast Random Integer Generation in an Interval",
| ACM Transactions on Modeling and Computer Simulation 29 (1), 2019,
| http://arxiv.org/abs/1805.10941.
|
| laplace(...)
| laplace(loc=0.0, scale=1.0, size=None)
|
| Draw samples from the Laplace or double exponential distribution with
| specified location (or mean) and scale (decay).
|
| The Laplace distribution is similar to the Gaussian/normal distribution,
| but is sharper at the peak and has fatter tails. It represents the
| difference between two independent, identically distributed exponential
| random variables.
|
| Parameters
| ----------
| loc : float or array_like of floats, optional
| The position, :math:`\mu`, of the distribution peak. Default is 0.
| scale : float or array_like of floats, optional
| :math:`\lambda`, the exponential decay. Default is 1. Must be non-
| negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Laplace distribution.
|
| Notes
| -----
| It has the probability density function
|
| .. math:: f(x; \mu, \lambda) = \frac{1}{2\lambda}
| \exp\left(-\frac{|x - \mu|}{\lambda}\right).
|
| The first law of Laplace, from 1774, states that the frequency
| of an error can be expressed as an exponential function of the
| absolute magnitude of the error, which leads to the Laplace
| distribution. For many problems in economics and health
| sciences, this distribution seems to model the data better
| than the standard Gaussian distribution.
|
| References
| ----------
| .. [1] Abramowitz, M. and Stegun, I. A. (Eds.). "Handbook of
| Mathematical Functions with Formulas, Graphs, and Mathematical
| Tables, 9th printing," New York: Dover, 1972.
| .. [2] Kotz, Samuel, et. al. "The Laplace Distribution and
| Generalizations, " Birkhauser, 2001.
| .. [3] Weisstein, Eric W. "Laplace Distribution."
| From MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/LaplaceDistribution.html
| .. [4] Wikipedia, "Laplace distribution",
| https://en.wikipedia.org/wiki/Laplace_distribution
|
| Examples
| --------
| Draw samples from the distribution
|
| >>> loc, scale = 0., 1.
| >>> s = np.random.default_rng().laplace(loc, scale, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 30, density=True)
| >>> x = np.arange(-8., 8., .01)
| >>> pdf = np.exp(-abs(x-loc)/scale)/(2.*scale)
| >>> plt.plot(x, pdf)
|
| Plot Gaussian for comparison:
|
| >>> g = (1/(scale * np.sqrt(2 * np.pi)) *
| ... np.exp(-(x - loc)**2 / (2 * scale**2)))
| >>> plt.plot(x,g)
|
| logistic(...)
| logistic(loc=0.0, scale=1.0, size=None)
|
| Draw samples from a logistic distribution.
|
| Samples are drawn from a logistic distribution with specified
| parameters, loc (location or mean, also median), and scale (>0).
|
| Parameters
| ----------
| loc : float or array_like of floats, optional
| Parameter of the distribution. Default is 0.
| scale : float or array_like of floats, optional
| Parameter of the distribution. Must be non-negative.
| Default is 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized logistic distribution.
|
| See Also
| --------
| scipy.stats.logistic : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the Logistic distribution is
|
| .. math:: P(x) = P(x) = \frac{e^{-(x-\mu)/s}}{s(1+e^{-(x-\mu)/s})^2},
|
| where :math:`\mu` = location and :math:`s` = scale.
|
| The Logistic distribution is used in Extreme Value problems where it
| can act as a mixture of Gumbel distributions, in Epidemiology, and by
| the World Chess Federation (FIDE) where it is used in the Elo ranking
| system, assuming the performance of each player is a logistically
| distributed random variable.
|
| References
| ----------
| .. [1] Reiss, R.-D. and Thomas M. (2001), "Statistical Analysis of
| Extreme Values, from Insurance, Finance, Hydrology and Other
| Fields," Birkhauser Verlag, Basel, pp 132-133.
| .. [2] Weisstein, Eric W. "Logistic Distribution." From
| MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/LogisticDistribution.html
| .. [3] Wikipedia, "Logistic-distribution",
| https://en.wikipedia.org/wiki/Logistic_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> loc, scale = 10, 1
| >>> s = np.random.default_rng().logistic(loc, scale, 10000)
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, bins=50)
|
| # plot against distribution
|
| >>> def logist(x, loc, scale):
| ... return np.exp((loc-x)/scale)/(scale*(1+np.exp((loc-x)/scale))**2)
| >>> lgst_val = logist(bins, loc, scale)
| >>> plt.plot(bins, lgst_val * count.max() / lgst_val.max())
| >>> plt.show()
|
| lognormal(...)
| lognormal(mean=0.0, sigma=1.0, size=None)
|
| Draw samples from a log-normal distribution.
|
| Draw samples from a log-normal distribution with specified mean,
| standard deviation, and array shape. Note that the mean and standard
| deviation are not the values for the distribution itself, but of the
| underlying normal distribution it is derived from.
|
| Parameters
| ----------
| mean : float or array_like of floats, optional
| Mean value of the underlying normal distribution. Default is 0.
| sigma : float or array_like of floats, optional
| Standard deviation of the underlying normal distribution. Must be
| non-negative. Default is 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``mean`` and ``sigma`` are both scalars.
| Otherwise, ``np.broadcast(mean, sigma).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized log-normal distribution.
|
| See Also
| --------
| scipy.stats.lognorm : probability density function, distribution,
| cumulative density function, etc.
|
| Notes
| -----
| A variable `x` has a log-normal distribution if `log(x)` is normally
| distributed. The probability density function for the log-normal
| distribution is:
|
| .. math:: p(x) = \frac{1}{\sigma x \sqrt{2\pi}}
| e^{(-\frac{(ln(x)-\mu)^2}{2\sigma^2})}
|
| where :math:`\mu` is the mean and :math:`\sigma` is the standard
| deviation of the normally distributed logarithm of the variable.
| A log-normal distribution results if a random variable is the *product*
| of a large number of independent, identically-distributed variables in
| the same way that a normal distribution results if the variable is the
| *sum* of a large number of independent, identically-distributed
| variables.
|
| References
| ----------
| .. [1] Limpert, E., Stahel, W. A., and Abbt, M., "Log-normal
| Distributions across the Sciences: Keys and Clues,"
| BioScience, Vol. 51, No. 5, May, 2001.
| https://stat.ethz.ch/~stahel/lognormal/bioscience.pdf
| .. [2] Reiss, R.D. and Thomas, M., "Statistical Analysis of Extreme
| Values," Basel: Birkhauser Verlag, 2001, pp. 31-32.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> rng = np.random.default_rng()
| >>> mu, sigma = 3., 1. # mean and standard deviation
| >>> s = rng.lognormal(mu, sigma, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 100, density=True, align='mid')
|
| >>> x = np.linspace(min(bins), max(bins), 10000)
| >>> pdf = (np.exp(-(np.log(x) - mu)**2 / (2 * sigma**2))
| ... / (x * sigma * np.sqrt(2 * np.pi)))
|
| >>> plt.plot(x, pdf, linewidth=2, color='r')
| >>> plt.axis('tight')
| >>> plt.show()
|
| Demonstrate that taking the products of random samples from a uniform
| distribution can be fit well by a log-normal probability density
| function.
|
| >>> # Generate a thousand samples: each is the product of 100 random
| >>> # values, drawn from a normal distribution.
| >>> rng = rng
| >>> b = []
| >>> for i in range(1000):
| ... a = 10. + rng.standard_normal(100)
| ... b.append(np.prod(a))
|
| >>> b = np.array(b) / np.min(b) # scale values to be positive
| >>> count, bins, ignored = plt.hist(b, 100, density=True, align='mid')
| >>> sigma = np.std(np.log(b))
| >>> mu = np.mean(np.log(b))
|
| >>> x = np.linspace(min(bins), max(bins), 10000)
| >>> pdf = (np.exp(-(np.log(x) - mu)**2 / (2 * sigma**2))
| ... / (x * sigma * np.sqrt(2 * np.pi)))
|
| >>> plt.plot(x, pdf, color='r', linewidth=2)
| >>> plt.show()
|
| logseries(...)
| logseries(p, size=None)
|
| Draw samples from a logarithmic series distribution.
|
| Samples are drawn from a log series distribution with specified
| shape parameter, 0 <= ``p`` < 1.
|
| Parameters
| ----------
| p : float or array_like of floats
| Shape parameter for the distribution. Must be in the range [0, 1).
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``p`` is a scalar. Otherwise,
| ``np.array(p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized logarithmic series distribution.
|
| See Also
| --------
| scipy.stats.logser : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability mass function for the Log Series distribution is
|
| .. math:: P(k) = \frac{-p^k}{k \ln(1-p)},
|
| where p = probability.
|
| The log series distribution is frequently used to represent species
| richness and occurrence, first proposed by Fisher, Corbet, and
| Williams in 1943 [2]. It may also be used to model the numbers of
| occupants seen in cars [3].
|
| References
| ----------
| .. [1] Buzas, Martin A.; Culver, Stephen J., Understanding regional
| species diversity through the log series distribution of
| occurrences: BIODIVERSITY RESEARCH Diversity & Distributions,
| Volume 5, Number 5, September 1999 , pp. 187-195(9).
| .. [2] Fisher, R.A,, A.S. Corbet, and C.B. Williams. 1943. The
| relation between the number of species and the number of
| individuals in a random sample of an animal population.
| Journal of Animal Ecology, 12:42-58.
| .. [3] D. J. Hand, F. Daly, D. Lunn, E. Ostrowski, A Handbook of Small
| Data Sets, CRC Press, 1994.
| .. [4] Wikipedia, "Logarithmic distribution",
| https://en.wikipedia.org/wiki/Logarithmic_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a = .6
| >>> s = np.random.default_rng().logseries(a, 10000)
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s)
|
| # plot against distribution
|
| >>> def logseries(k, p):
| ... return -p**k/(k*np.log(1-p))
| >>> plt.plot(bins, logseries(bins, a) * count.max()/
| ... logseries(bins, a).max(), 'r')
| >>> plt.show()
|
| multinomial(...)
| multinomial(n, pvals, size=None)
|
| Draw samples from a multinomial distribution.
|
| The multinomial distribution is a multivariate generalization of the
| binomial distribution. Take an experiment with one of ``p``
| possible outcomes. An example of such an experiment is throwing a dice,
| where the outcome can be 1 through 6. Each sample drawn from the
| distribution represents `n` such experiments. Its values,
| ``X_i = [X_0, X_1, ..., X_p]``, represent the number of times the
| outcome was ``i``.
|
| Parameters
| ----------
| n : int or array-like of ints
| Number of experiments.
| pvals : array-like of floats
| Probabilities of each of the ``p`` different outcomes with shape
| ``(k0, k1, ..., kn, p)``. Each element ``pvals[i,j,...,:]`` must
| sum to 1 (however, the last element is always assumed to account
| for the remaining probability, as long as
| ``sum(pvals[..., :-1], axis=-1) <= 1.0``. Must have at least 1
| dimension where pvals.shape[-1] > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn each with ``p`` elements. Default
| is None where the output size is determined by the broadcast shape
| of ``n`` and all by the final dimension of ``pvals``, which is
| denoted as ``b=(b0, b1, ..., bq)``. If size is not None, then it
| must be compatible with the broadcast shape ``b``. Specifically,
| size must have ``q`` or more elements and size[-(q-j):] must equal
| ``bj``.
|
| Returns
| -------
| out : ndarray
| The drawn samples, of shape size, if provided. When size is
| provided, the output shape is size + (p,) If not specified,
| the shape is determined by the broadcast shape of ``n`` and
| ``pvals``, ``(b0, b1, ..., bq)`` augmented with the dimension of
| the multinomial, ``p``, so that that output shape is
| ``(b0, b1, ..., bq, p)``.
|
| Each entry ``out[i,j,...,:]`` is a ``p``-dimensional value drawn
| from the distribution.
|
| .. versionchanged:: 1.22.0
| Added support for broadcasting `pvals` against `n`
|
| Examples
| --------
| Throw a dice 20 times:
|
| >>> rng = np.random.default_rng()
| >>> rng.multinomial(20, [1/6.]*6, size=1)
| array([[4, 1, 7, 5, 2, 1]]) # random
|
| It landed 4 times on 1, once on 2, etc.
|
| Now, throw the dice 20 times, and 20 times again:
|
| >>> rng.multinomial(20, [1/6.]*6, size=2)
| array([[3, 4, 3, 3, 4, 3],
| [2, 4, 3, 4, 0, 7]]) # random
|
| For the first run, we threw 3 times 1, 4 times 2, etc. For the second,
| we threw 2 times 1, 4 times 2, etc.
|
| Now, do one experiment throwing the dice 10 time, and 10 times again,
| and another throwing the dice 20 times, and 20 times again:
|
| >>> rng.multinomial([[10], [20]], [1/6.]*6, size=(2, 2))
| array([[[2, 4, 0, 1, 2, 1],
| [1, 3, 0, 3, 1, 2]],
| [[1, 4, 4, 4, 4, 3],
| [3, 3, 2, 5, 5, 2]]]) # random
|
| The first array shows the outcomes of throwing the dice 10 times, and
| the second shows the outcomes from throwing the dice 20 times.
|
| A loaded die is more likely to land on number 6:
|
| >>> rng.multinomial(100, [1/7.]*5 + [2/7.])
| array([11, 16, 14, 17, 16, 26]) # random
|
| Simulate 10 throws of a 4-sided die and 20 throws of a 6-sided die
|
| >>> rng.multinomial([10, 20],[[1/4]*4 + [0]*2, [1/6]*6])
| array([[2, 1, 4, 3, 0, 0],
| [3, 3, 3, 6, 1, 4]], dtype=int64) # random
|
| Generate categorical random variates from two categories where the
| first has 3 outcomes and the second has 2.
|
| >>> rng.multinomial(1, [[.1, .5, .4 ], [.3, .7, .0]])
| array([[0, 0, 1],
| [0, 1, 0]], dtype=int64) # random
|
| ``argmax(axis=-1)`` is then used to return the categories.
|
| >>> pvals = [[.1, .5, .4 ], [.3, .7, .0]]
| >>> rvs = rng.multinomial(1, pvals, size=(4,2))
| >>> rvs.argmax(axis=-1)
| array([[0, 1],
| [2, 0],
| [2, 1],
| [2, 0]], dtype=int64) # random
|
| The same output dimension can be produced using broadcasting.
|
| >>> rvs = rng.multinomial([[1]] * 4, pvals)
| >>> rvs.argmax(axis=-1)
| array([[0, 1],
| [2, 0],
| [2, 1],
| [2, 0]], dtype=int64) # random
|
| The probability inputs should be normalized. As an implementation
| detail, the value of the last entry is ignored and assumed to take
| up any leftover probability mass, but this should not be relied on.
| A biased coin which has twice as much weight on one side as on the
| other should be sampled like so:
|
| >>> rng.multinomial(100, [1.0 / 3, 2.0 / 3]) # RIGHT
| array([38, 62]) # random
|
| not like:
|
| >>> rng.multinomial(100, [1.0, 2.0]) # WRONG
| Traceback (most recent call last):
| ValueError: pvals < 0, pvals > 1 or pvals contains NaNs
|
| multivariate_hypergeometric(...)
| multivariate_hypergeometric(colors, nsample, size=None,
| method='marginals')
|
| Generate variates from a multivariate hypergeometric distribution.
|
| The multivariate hypergeometric distribution is a generalization
| of the hypergeometric distribution.
|
| Choose ``nsample`` items at random without replacement from a
| collection with ``N`` distinct types. ``N`` is the length of
| ``colors``, and the values in ``colors`` are the number of occurrences
| of that type in the collection. The total number of items in the
| collection is ``sum(colors)``. Each random variate generated by this
| function is a vector of length ``N`` holding the counts of the
| different types that occurred in the ``nsample`` items.
|
| The name ``colors`` comes from a common description of the
| distribution: it is the probability distribution of the number of
| marbles of each color selected without replacement from an urn
| containing marbles of different colors; ``colors[i]`` is the number
| of marbles in the urn with color ``i``.
|
| Parameters
| ----------
| colors : sequence of integers
| The number of each type of item in the collection from which
| a sample is drawn. The values in ``colors`` must be nonnegative.
| To avoid loss of precision in the algorithm, ``sum(colors)``
| must be less than ``10**9`` when `method` is "marginals".
| nsample : int
| The number of items selected. ``nsample`` must not be greater
| than ``sum(colors)``.
| size : int or tuple of ints, optional
| The number of variates to generate, either an integer or a tuple
| holding the shape of the array of variates. If the given size is,
| e.g., ``(k, m)``, then ``k * m`` variates are drawn, where one
| variate is a vector of length ``len(colors)``, and the return value
| has shape ``(k, m, len(colors))``. If `size` is an integer, the
| output has shape ``(size, len(colors))``. Default is None, in
| which case a single variate is returned as an array with shape
| ``(len(colors),)``.
| method : string, optional
| Specify the algorithm that is used to generate the variates.
| Must be 'count' or 'marginals' (the default). See the Notes
| for a description of the methods.
|
| Returns
| -------
| variates : ndarray
| Array of variates drawn from the multivariate hypergeometric
| distribution.
|
| See Also
| --------
| hypergeometric : Draw samples from the (univariate) hypergeometric
| distribution.
|
| Notes
| -----
| The two methods do not return the same sequence of variates.
|
| The "count" algorithm is roughly equivalent to the following numpy
| code::
|
| choices = np.repeat(np.arange(len(colors)), colors)
| selection = np.random.choice(choices, nsample, replace=False)
| variate = np.bincount(selection, minlength=len(colors))
|
| The "count" algorithm uses a temporary array of integers with length
| ``sum(colors)``.
|
| The "marginals" algorithm generates a variate by using repeated
| calls to the univariate hypergeometric sampler. It is roughly
| equivalent to::
|
| variate = np.zeros(len(colors), dtype=np.int64)
| # `remaining` is the cumulative sum of `colors` from the last
| # element to the first; e.g. if `colors` is [3, 1, 5], then
| # `remaining` is [9, 6, 5].
| remaining = np.cumsum(colors[::-1])[::-1]
| for i in range(len(colors)-1):
| if nsample < 1:
| break
| variate[i] = hypergeometric(colors[i], remaining[i+1],
| nsample)
| nsample -= variate[i]
| variate[-1] = nsample
|
| The default method is "marginals". For some cases (e.g. when
| `colors` contains relatively small integers), the "count" method
| can be significantly faster than the "marginals" method. If
| performance of the algorithm is important, test the two methods
| with typical inputs to decide which works best.
|
| .. versionadded:: 1.18.0
|
| Examples
| --------
| >>> colors = [16, 8, 4]
| >>> seed = 4861946401452
| >>> gen = np.random.Generator(np.random.PCG64(seed))
| >>> gen.multivariate_hypergeometric(colors, 6)
| array([5, 0, 1])
| >>> gen.multivariate_hypergeometric(colors, 6, size=3)
| array([[5, 0, 1],
| [2, 2, 2],
| [3, 3, 0]])
| >>> gen.multivariate_hypergeometric(colors, 6, size=(2, 2))
| array([[[3, 2, 1],
| [3, 2, 1]],
| [[4, 1, 1],
| [3, 2, 1]]])
|
| multivariate_normal(...)
| multivariate_normal(mean, cov, size=None, check_valid='warn',
| tol=1e-8, *, method='svd')
|
| Draw random samples from a multivariate normal distribution.
|
| The multivariate normal, multinormal or Gaussian distribution is a
| generalization of the one-dimensional normal distribution to higher
| dimensions. Such a distribution is specified by its mean and
| covariance matrix. These parameters are analogous to the mean
| (average or "center") and variance (the squared standard deviation,
| or "width") of the one-dimensional normal distribution.
|
| Parameters
| ----------
| mean : 1-D array_like, of length N
| Mean of the N-dimensional distribution.
| cov : 2-D array_like, of shape (N, N)
| Covariance matrix of the distribution. It must be symmetric and
| positive-semidefinite for proper sampling.
| size : int or tuple of ints, optional
| Given a shape of, for example, ``(m,n,k)``, ``m*n*k`` samples are
| generated, and packed in an `m`-by-`n`-by-`k` arrangement. Because
| each sample is `N`-dimensional, the output shape is ``(m,n,k,N)``.
| If no shape is specified, a single (`N`-D) sample is returned.
| check_valid : { 'warn', 'raise', 'ignore' }, optional
| Behavior when the covariance matrix is not positive semidefinite.
| tol : float, optional
| Tolerance when checking the singular values in covariance matrix.
| cov is cast to double before the check.
| method : { 'svd', 'eigh', 'cholesky'}, optional
| The cov input is used to compute a factor matrix A such that
| ``A @ A.T = cov``. This argument is used to select the method
| used to compute the factor matrix A. The default method 'svd' is
| the slowest, while 'cholesky' is the fastest but less robust than
| the slowest method. The method `eigh` uses eigen decomposition to
| compute A and is faster than svd but slower than cholesky.
|
| .. versionadded:: 1.18.0
|
| Returns
| -------
| out : ndarray
| The drawn samples, of shape *size*, if that was provided. If not,
| the shape is ``(N,)``.
|
| In other words, each entry ``out[i,j,...,:]`` is an N-dimensional
| value drawn from the distribution.
|
| Notes
| -----
| The mean is a coordinate in N-dimensional space, which represents the
| location where samples are most likely to be generated. This is
| analogous to the peak of the bell curve for the one-dimensional or
| univariate normal distribution.
|
| Covariance indicates the level to which two variables vary together.
| From the multivariate normal distribution, we draw N-dimensional
| samples, :math:`X = [x_1, x_2, ... x_N]`. The covariance matrix
| element :math:`C_{ij}` is the covariance of :math:`x_i` and :math:`x_j`.
| The element :math:`C_{ii}` is the variance of :math:`x_i` (i.e. its
| "spread").
|
| Instead of specifying the full covariance matrix, popular
| approximations include:
|
| - Spherical covariance (`cov` is a multiple of the identity matrix)
| - Diagonal covariance (`cov` has non-negative elements, and only on
| the diagonal)
|
| This geometrical property can be seen in two dimensions by plotting
| generated data-points:
|
| >>> mean = [0, 0]
| >>> cov = [[1, 0], [0, 100]] # diagonal covariance
|
| Diagonal covariance means that points are oriented along x or y-axis:
|
| >>> import matplotlib.pyplot as plt
| >>> x, y = np.random.default_rng().multivariate_normal(mean, cov, 5000).T
| >>> plt.plot(x, y, 'x')
| >>> plt.axis('equal')
| >>> plt.show()
|
| Note that the covariance matrix must be positive semidefinite (a.k.a.
| nonnegative-definite). Otherwise, the behavior of this method is
| undefined and backwards compatibility is not guaranteed.
|
| This function internally uses linear algebra routines, and thus results
| may not be identical (even up to precision) across architectures, OSes,
| or even builds. For example, this is likely if ``cov`` has multiple equal
| singular values and ``method`` is ``'svd'`` (default). In this case,
| ``method='cholesky'`` may be more robust.
|
| References
| ----------
| .. [1] Papoulis, A., "Probability, Random Variables, and Stochastic
| Processes," 3rd ed., New York: McGraw-Hill, 1991.
| .. [2] Duda, R. O., Hart, P. E., and Stork, D. G., "Pattern
| Classification," 2nd ed., New York: Wiley, 2001.
|
| Examples
| --------
| >>> mean = (1, 2)
| >>> cov = [[1, 0], [0, 1]]
| >>> rng = np.random.default_rng()
| >>> x = rng.multivariate_normal(mean, cov, (3, 3))
| >>> x.shape
| (3, 3, 2)
|
| We can use a different method other than the default to factorize cov:
|
| >>> y = rng.multivariate_normal(mean, cov, (3, 3), method='cholesky')
| >>> y.shape
| (3, 3, 2)
|
| Here we generate 800 samples from the bivariate normal distribution
| with mean [0, 0] and covariance matrix [[6, -3], [-3, 3.5]]. The
| expected variances of the first and second components of the sample
| are 6 and 3.5, respectively, and the expected correlation
| coefficient is -3/sqrt(6*3.5) ≈ -0.65465.
|
| >>> cov = np.array([[6, -3], [-3, 3.5]])
| >>> pts = rng.multivariate_normal([0, 0], cov, size=800)
|
| Check that the mean, covariance, and correlation coefficient of the
| sample are close to the expected values:
|
| >>> pts.mean(axis=0)
| array([ 0.0326911 , -0.01280782]) # may vary
| >>> np.cov(pts.T)
| array([[ 5.96202397, -2.85602287],
| [-2.85602287, 3.47613949]]) # may vary
| >>> np.corrcoef(pts.T)[0, 1]
| -0.6273591314603949 # may vary
|
| We can visualize this data with a scatter plot. The orientation
| of the point cloud illustrates the negative correlation of the
| components of this sample.
|
| >>> import matplotlib.pyplot as plt
| >>> plt.plot(pts[:, 0], pts[:, 1], '.', alpha=0.5)
| >>> plt.axis('equal')
| >>> plt.grid()
| >>> plt.show()
|
| negative_binomial(...)
| negative_binomial(n, p, size=None)
|
| Draw samples from a negative binomial distribution.
|
| Samples are drawn from a negative binomial distribution with specified
| parameters, `n` successes and `p` probability of success where `n`
| is > 0 and `p` is in the interval (0, 1].
|
| Parameters
| ----------
| n : float or array_like of floats
| Parameter of the distribution, > 0.
| p : float or array_like of floats
| Parameter of the distribution. Must satisfy 0 < p <= 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``n`` and ``p`` are both scalars.
| Otherwise, ``np.broadcast(n, p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized negative binomial distribution,
| where each sample is equal to N, the number of failures that
| occurred before a total of n successes was reached.
|
| Notes
| -----
| The probability mass function of the negative binomial distribution is
|
| .. math:: P(N;n,p) = \frac{\Gamma(N+n)}{N!\Gamma(n)}p^{n}(1-p)^{N},
|
| where :math:`n` is the number of successes, :math:`p` is the
| probability of success, :math:`N+n` is the number of trials, and
| :math:`\Gamma` is the gamma function. When :math:`n` is an integer,
| :math:`\frac{\Gamma(N+n)}{N!\Gamma(n)} = \binom{N+n-1}{N}`, which is
| the more common form of this term in the pmf. The negative
| binomial distribution gives the probability of N failures given n
| successes, with a success on the last trial.
|
| If one throws a die repeatedly until the third time a "1" appears,
| then the probability distribution of the number of non-"1"s that
| appear before the third "1" is a negative binomial distribution.
|
| Because this method internally calls ``Generator.poisson`` with an
| intermediate random value, a ValueError is raised when the choice of
| :math:`n` and :math:`p` would result in the mean + 10 sigma of the sampled
| intermediate distribution exceeding the max acceptable value of the
| ``Generator.poisson`` method. This happens when :math:`p` is too low
| (a lot of failures happen for every success) and :math:`n` is too big (
| a lot of successes are allowed).
| Therefore, the :math:`n` and :math:`p` values must satisfy the constraint:
|
| .. math:: n\frac{1-p}{p}+10n\sqrt{n}\frac{1-p}{p}<2^{63}-1-10\sqrt{2^{63}-1},
|
| Where the left side of the equation is the derived mean + 10 sigma of
| a sample from the gamma distribution internally used as the :math:`lam`
| parameter of a poisson sample, and the right side of the equation is
| the constraint for maximum value of :math:`lam` in ``Generator.poisson``.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Negative Binomial Distribution." From
| MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/NegativeBinomialDistribution.html
| .. [2] Wikipedia, "Negative binomial distribution",
| https://en.wikipedia.org/wiki/Negative_binomial_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| A real world example. A company drills wild-cat oil
| exploration wells, each with an estimated probability of
| success of 0.1. What is the probability of having one success
| for each successive well, that is what is the probability of a
| single success after drilling 5 wells, after 6 wells, etc.?
|
| >>> s = np.random.default_rng().negative_binomial(1, 0.1, 100000)
| >>> for i in range(1, 11): # doctest: +SKIP
| ... probability = sum(s<i) / 100000.
| ... print(i, "wells drilled, probability of one success =", probability)
|
| noncentral_chisquare(...)
| noncentral_chisquare(df, nonc, size=None)
|
| Draw samples from a noncentral chi-square distribution.
|
| The noncentral :math:`\chi^2` distribution is a generalization of
| the :math:`\chi^2` distribution.
|
| Parameters
| ----------
| df : float or array_like of floats
| Degrees of freedom, must be > 0.
|
| .. versionchanged:: 1.10.0
| Earlier NumPy versions required dfnum > 1.
| nonc : float or array_like of floats
| Non-centrality, must be non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``df`` and ``nonc`` are both scalars.
| Otherwise, ``np.broadcast(df, nonc).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized noncentral chi-square distribution.
|
| Notes
| -----
| The probability density function for the noncentral Chi-square
| distribution is
|
| .. math:: P(x;df,nonc) = \sum^{\infty}_{i=0}
| \frac{e^{-nonc/2}(nonc/2)^{i}}{i!}
| P_{Y_{df+2i}}(x),
|
| where :math:`Y_{q}` is the Chi-square with q degrees of freedom.
|
| References
| ----------
| .. [1] Wikipedia, "Noncentral chi-squared distribution"
| https://en.wikipedia.org/wiki/Noncentral_chi-squared_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram
|
| >>> rng = np.random.default_rng()
| >>> import matplotlib.pyplot as plt
| >>> values = plt.hist(rng.noncentral_chisquare(3, 20, 100000),
| ... bins=200, density=True)
| >>> plt.show()
|
| Draw values from a noncentral chisquare with very small noncentrality,
| and compare to a chisquare.
|
| >>> plt.figure()
| >>> values = plt.hist(rng.noncentral_chisquare(3, .0000001, 100000),
| ... bins=np.arange(0., 25, .1), density=True)
| >>> values2 = plt.hist(rng.chisquare(3, 100000),
| ... bins=np.arange(0., 25, .1), density=True)
| >>> plt.plot(values[1][0:-1], values[0]-values2[0], 'ob')
| >>> plt.show()
|
| Demonstrate how large values of non-centrality lead to a more symmetric
| distribution.
|
| >>> plt.figure()
| >>> values = plt.hist(rng.noncentral_chisquare(3, 20, 100000),
| ... bins=200, density=True)
| >>> plt.show()
|
| noncentral_f(...)
| noncentral_f(dfnum, dfden, nonc, size=None)
|
| Draw samples from the noncentral F distribution.
|
| Samples are drawn from an F distribution with specified parameters,
| `dfnum` (degrees of freedom in numerator) and `dfden` (degrees of
| freedom in denominator), where both parameters > 1.
| `nonc` is the non-centrality parameter.
|
| Parameters
| ----------
| dfnum : float or array_like of floats
| Numerator degrees of freedom, must be > 0.
|
| .. versionchanged:: 1.14.0
| Earlier NumPy versions required dfnum > 1.
| dfden : float or array_like of floats
| Denominator degrees of freedom, must be > 0.
| nonc : float or array_like of floats
| Non-centrality parameter, the sum of the squares of the numerator
| means, must be >= 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``dfnum``, ``dfden``, and ``nonc``
| are all scalars. Otherwise, ``np.broadcast(dfnum, dfden, nonc).size``
| samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized noncentral Fisher distribution.
|
| Notes
| -----
| When calculating the power of an experiment (power = probability of
| rejecting the null hypothesis when a specific alternative is true) the
| non-central F statistic becomes important. When the null hypothesis is
| true, the F statistic follows a central F distribution. When the null
| hypothesis is not true, then it follows a non-central F statistic.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Noncentral F-Distribution."
| From MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/NoncentralF-Distribution.html
| .. [2] Wikipedia, "Noncentral F-distribution",
| https://en.wikipedia.org/wiki/Noncentral_F-distribution
|
| Examples
| --------
| In a study, testing for a specific alternative to the null hypothesis
| requires use of the Noncentral F distribution. We need to calculate the
| area in the tail of the distribution that exceeds the value of the F
| distribution for the null hypothesis. We'll plot the two probability
| distributions for comparison.
|
| >>> rng = np.random.default_rng()
| >>> dfnum = 3 # between group deg of freedom
| >>> dfden = 20 # within groups degrees of freedom
| >>> nonc = 3.0
| >>> nc_vals = rng.noncentral_f(dfnum, dfden, nonc, 1000000)
| >>> NF = np.histogram(nc_vals, bins=50, density=True)
| >>> c_vals = rng.f(dfnum, dfden, 1000000)
| >>> F = np.histogram(c_vals, bins=50, density=True)
| >>> import matplotlib.pyplot as plt
| >>> plt.plot(F[1][1:], F[0])
| >>> plt.plot(NF[1][1:], NF[0])
| >>> plt.show()
|
| normal(...)
| normal(loc=0.0, scale=1.0, size=None)
|
| Draw random samples from a normal (Gaussian) distribution.
|
| The probability density function of the normal distribution, first
| derived by De Moivre and 200 years later by both Gauss and Laplace
| independently [2]_, is often called the bell curve because of
| its characteristic shape (see the example below).
|
| The normal distributions occurs often in nature. For example, it
| describes the commonly occurring distribution of samples influenced
| by a large number of tiny, random disturbances, each with its own
| unique distribution [2]_.
|
| Parameters
| ----------
| loc : float or array_like of floats
| Mean ("centre") of the distribution.
| scale : float or array_like of floats
| Standard deviation (spread or "width") of the distribution. Must be
| non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized normal distribution.
|
| See Also
| --------
| scipy.stats.norm : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the Gaussian distribution is
|
| .. math:: p(x) = \frac{1}{\sqrt{ 2 \pi \sigma^2 }}
| e^{ - \frac{ (x - \mu)^2 } {2 \sigma^2} },
|
| where :math:`\mu` is the mean and :math:`\sigma` the standard
| deviation. The square of the standard deviation, :math:`\sigma^2`,
| is called the variance.
|
| The function has its peak at the mean, and its "spread" increases with
| the standard deviation (the function reaches 0.607 times its maximum at
| :math:`x + \sigma` and :math:`x - \sigma` [2]_). This implies that
| :meth:`normal` is more likely to return samples lying close to the
| mean, rather than those far away.
|
| References
| ----------
| .. [1] Wikipedia, "Normal distribution",
| https://en.wikipedia.org/wiki/Normal_distribution
| .. [2] P. R. Peebles Jr., "Central Limit Theorem" in "Probability,
| Random Variables and Random Signal Principles", 4th ed., 2001,
| pp. 51, 51, 125.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> mu, sigma = 0, 0.1 # mean and standard deviation
| >>> s = np.random.default_rng().normal(mu, sigma, 1000)
|
| Verify the mean and the variance:
|
| >>> abs(mu - np.mean(s))
| 0.0 # may vary
|
| >>> abs(sigma - np.std(s, ddof=1))
| 0.0 # may vary
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 30, density=True)
| >>> plt.plot(bins, 1/(sigma * np.sqrt(2 * np.pi)) *
| ... np.exp( - (bins - mu)**2 / (2 * sigma**2) ),
| ... linewidth=2, color='r')
| >>> plt.show()
|
| Two-by-four array of samples from the normal distribution with
| mean 3 and standard deviation 2.5:
|
| >>> np.random.default_rng().normal(3, 2.5, size=(2, 4))
| array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
| [ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
|
| pareto(...)
| pareto(a, size=None)
|
| Draw samples from a Pareto II or Lomax distribution with
| specified shape.
|
| The Lomax or Pareto II distribution is a shifted Pareto
| distribution. The classical Pareto distribution can be
| obtained from the Lomax distribution by adding 1 and
| multiplying by the scale parameter ``m`` (see Notes). The
| smallest value of the Lomax distribution is zero while for the
| classical Pareto distribution it is ``mu``, where the standard
| Pareto distribution has location ``mu = 1``. Lomax can also
| be considered as a simplified version of the Generalized
| Pareto distribution (available in SciPy), with the scale set
| to one and the location set to zero.
|
| The Pareto distribution must be greater than zero, and is
| unbounded above. It is also known as the "80-20 rule". In
| this distribution, 80 percent of the weights are in the lowest
| 20 percent of the range, while the other 20 percent fill the
| remaining 80 percent of the range.
|
| Parameters
| ----------
| a : float or array_like of floats
| Shape of the distribution. Must be positive.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Pareto distribution.
|
| See Also
| --------
| scipy.stats.lomax : probability density function, distribution or
| cumulative density function, etc.
| scipy.stats.genpareto : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the Pareto distribution is
|
| .. math:: p(x) = \frac{am^a}{x^{a+1}}
|
| where :math:`a` is the shape and :math:`m` the scale.
|
| The Pareto distribution, named after the Italian economist
| Vilfredo Pareto, is a power law probability distribution
| useful in many real world problems. Outside the field of
| economics it is generally referred to as the Bradford
| distribution. Pareto developed the distribution to describe
| the distribution of wealth in an economy. It has also found
| use in insurance, web page access statistics, oil field sizes,
| and many other problems, including the download frequency for
| projects in Sourceforge [1]_. It is one of the so-called
| "fat-tailed" distributions.
|
|
| References
| ----------
| .. [1] Francis Hunt and Paul Johnson, On the Pareto Distribution of
| Sourceforge projects.
| .. [2] Pareto, V. (1896). Course of Political Economy. Lausanne.
| .. [3] Reiss, R.D., Thomas, M.(2001), Statistical Analysis of Extreme
| Values, Birkhauser Verlag, Basel, pp 23-30.
| .. [4] Wikipedia, "Pareto distribution",
| https://en.wikipedia.org/wiki/Pareto_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a, m = 3., 2. # shape and mode
| >>> s = (np.random.default_rng().pareto(a, 1000) + 1) * m
|
| Display the histogram of the samples, along with the probability
| density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, _ = plt.hist(s, 100, density=True)
| >>> fit = a*m**a / bins**(a+1)
| >>> plt.plot(bins, max(count)*fit/max(fit), linewidth=2, color='r')
| >>> plt.show()
|
| permutation(...)
| permutation(x, axis=0)
|
| Randomly permute a sequence, or return a permuted range.
|
| Parameters
| ----------
| x : int or array_like
| If `x` is an integer, randomly permute ``np.arange(x)``.
| If `x` is an array, make a copy and shuffle the elements
| randomly.
| axis : int, optional
| The axis which `x` is shuffled along. Default is 0.
|
| Returns
| -------
| out : ndarray
| Permuted sequence or array range.
|
| Examples
| --------
| >>> rng = np.random.default_rng()
| >>> rng.permutation(10)
| array([1, 7, 4, 3, 0, 9, 2, 5, 8, 6]) # random
|
| >>> rng.permutation([1, 4, 9, 12, 15])
| array([15, 1, 9, 4, 12]) # random
|
| >>> arr = np.arange(9).reshape((3, 3))
| >>> rng.permutation(arr)
| array([[6, 7, 8], # random
| [0, 1, 2],
| [3, 4, 5]])
|
| >>> rng.permutation("abc")
| Traceback (most recent call last):
| ...
| numpy.exceptions.AxisError: axis 0 is out of bounds for array of dimension 0
|
| >>> arr = np.arange(9).reshape((3, 3))
| >>> rng.permutation(arr, axis=1)
| array([[0, 2, 1], # random
| [3, 5, 4],
| [6, 8, 7]])
|
| permuted(...)
| permuted(x, axis=None, out=None)
|
| Randomly permute `x` along axis `axis`.
|
| Unlike `shuffle`, each slice along the given axis is shuffled
| independently of the others.
|
| Parameters
| ----------
| x : array_like, at least one-dimensional
| Array to be shuffled.
| axis : int, optional
| Slices of `x` in this axis are shuffled. Each slice
| is shuffled independently of the others. If `axis` is
| None, the flattened array is shuffled.
| out : ndarray, optional
| If given, this is the destination of the shuffled array.
| If `out` is None, a shuffled copy of the array is returned.
|
| Returns
| -------
| ndarray
| If `out` is None, a shuffled copy of `x` is returned.
| Otherwise, the shuffled array is stored in `out`,
| and `out` is returned
|
| See Also
| --------
| shuffle
| permutation
|
| Notes
| -----
| An important distinction between methods ``shuffle`` and ``permuted`` is
| how they both treat the ``axis`` parameter which can be found at
| :ref:`generator-handling-axis-parameter`.
|
| Examples
| --------
| Create a `numpy.random.Generator` instance:
|
| >>> rng = np.random.default_rng()
|
| Create a test array:
|
| >>> x = np.arange(24).reshape(3, 8)
| >>> x
| array([[ 0, 1, 2, 3, 4, 5, 6, 7],
| [ 8, 9, 10, 11, 12, 13, 14, 15],
| [16, 17, 18, 19, 20, 21, 22, 23]])
|
| Shuffle the rows of `x`:
|
| >>> y = rng.permuted(x, axis=1)
| >>> y
| array([[ 4, 3, 6, 7, 1, 2, 5, 0], # random
| [15, 10, 14, 9, 12, 11, 8, 13],
| [17, 16, 20, 21, 18, 22, 23, 19]])
|
| `x` has not been modified:
|
| >>> x
| array([[ 0, 1, 2, 3, 4, 5, 6, 7],
| [ 8, 9, 10, 11, 12, 13, 14, 15],
| [16, 17, 18, 19, 20, 21, 22, 23]])
|
| To shuffle the rows of `x` in-place, pass `x` as the `out`
| parameter:
|
| >>> y = rng.permuted(x, axis=1, out=x)
| >>> x
| array([[ 3, 0, 4, 7, 1, 6, 2, 5], # random
| [ 8, 14, 13, 9, 12, 11, 15, 10],
| [17, 18, 16, 22, 19, 23, 20, 21]])
|
| Note that when the ``out`` parameter is given, the return
| value is ``out``:
|
| >>> y is x
| True
|
| poisson(...)
| poisson(lam=1.0, size=None)
|
| Draw samples from a Poisson distribution.
|
| The Poisson distribution is the limit of the binomial distribution
| for large N.
|
| Parameters
| ----------
| lam : float or array_like of floats
| Expected number of events occurring in a fixed-time interval,
| must be >= 0. A sequence must be broadcastable over the requested
| size.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``lam`` is a scalar. Otherwise,
| ``np.array(lam).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Poisson distribution.
|
| Notes
| -----
| The Poisson distribution
|
| .. math:: f(k; \lambda)=\frac{\lambda^k e^{-\lambda}}{k!}
|
| For events with an expected separation :math:`\lambda` the Poisson
| distribution :math:`f(k; \lambda)` describes the probability of
| :math:`k` events occurring within the observed
| interval :math:`\lambda`.
|
| Because the output is limited to the range of the C int64 type, a
| ValueError is raised when `lam` is within 10 sigma of the maximum
| representable value.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Poisson Distribution."
| From MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/PoissonDistribution.html
| .. [2] Wikipedia, "Poisson distribution",
| https://en.wikipedia.org/wiki/Poisson_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> import numpy as np
| >>> rng = np.random.default_rng()
| >>> s = rng.poisson(5, 10000)
|
| Display histogram of the sample:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 14, density=True)
| >>> plt.show()
|
| Draw each 100 values for lambda 100 and 500:
|
| >>> s = rng.poisson(lam=(100., 500.), size=(100, 2))
|
| power(...)
| power(a, size=None)
|
| Draws samples in [0, 1] from a power distribution with positive
| exponent a - 1.
|
| Also known as the power function distribution.
|
| Parameters
| ----------
| a : float or array_like of floats
| Parameter of the distribution. Must be non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized power distribution.
|
| Raises
| ------
| ValueError
| If a <= 0.
|
| Notes
| -----
| The probability density function is
|
| .. math:: P(x; a) = ax^{a-1}, 0 \le x \le 1, a>0.
|
| The power function distribution is just the inverse of the Pareto
| distribution. It may also be seen as a special case of the Beta
| distribution.
|
| It is used, for example, in modeling the over-reporting of insurance
| claims.
|
| References
| ----------
| .. [1] Christian Kleiber, Samuel Kotz, "Statistical size distributions
| in economics and actuarial sciences", Wiley, 2003.
| .. [2] Heckert, N. A. and Filliben, James J. "NIST Handbook 148:
| Dataplot Reference Manual, Volume 2: Let Subcommands and Library
| Functions", National Institute of Standards and Technology
| Handbook Series, June 2003.
| https://www.itl.nist.gov/div898/software/dataplot/refman2/auxillar/powpdf.pdf
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> rng = np.random.default_rng()
| >>> a = 5. # shape
| >>> samples = 1000
| >>> s = rng.power(a, samples)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, bins=30)
| >>> x = np.linspace(0, 1, 100)
| >>> y = a*x**(a-1.)
| >>> normed_y = samples*np.diff(bins)[0]*y
| >>> plt.plot(x, normed_y)
| >>> plt.show()
|
| Compare the power function distribution to the inverse of the Pareto.
|
| >>> from scipy import stats # doctest: +SKIP
| >>> rvs = rng.power(5, 1000000)
| >>> rvsp = rng.pareto(5, 1000000)
| >>> xx = np.linspace(0,1,100)
| >>> powpdf = stats.powerlaw.pdf(xx,5) # doctest: +SKIP
|
| >>> plt.figure()
| >>> plt.hist(rvs, bins=50, density=True)
| >>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
| >>> plt.title('power(5)')
|
| >>> plt.figure()
| >>> plt.hist(1./(1.+rvsp), bins=50, density=True)
| >>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
| >>> plt.title('inverse of 1 + Generator.pareto(5)')
|
| >>> plt.figure()
| >>> plt.hist(1./(1.+rvsp), bins=50, density=True)
| >>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
| >>> plt.title('inverse of stats.pareto(5)')
|
| random(...)
| random(size=None, dtype=np.float64, out=None)
|
| Return random floats in the half-open interval [0.0, 1.0).
|
| Results are from the "continuous uniform" distribution over the
| stated interval. To sample :math:`Unif[a, b), b > a` use `uniform`
| or multiply the output of `random` by ``(b - a)`` and add ``a``::
|
| (b - a) * random() + a
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| dtype : dtype, optional
| Desired dtype of the result, only `float64` and `float32` are supported.
| Byteorder must be native. The default value is np.float64.
| out : ndarray, optional
| Alternative output array in which to place the result. If size is not None,
| it must have the same shape as the provided size and must match the type of
| the output values.
|
| Returns
| -------
| out : float or ndarray of floats
| Array of random floats of shape `size` (unless ``size=None``, in which
| case a single float is returned).
|
| See Also
| --------
| uniform : Draw samples from the parameterized uniform distribution.
|
| Examples
| --------
| >>> rng = np.random.default_rng()
| >>> rng.random()
| 0.47108547995356098 # random
| >>> type(rng.random())
| <class 'float'>
| >>> rng.random((5,))
| array([ 0.30220482, 0.86820401, 0.1654503 , 0.11659149, 0.54323428]) # random
|
| Three-by-two array of random numbers from [-5, 0):
|
| >>> 5 * rng.random((3, 2)) - 5
| array([[-3.99149989, -0.52338984], # random
| [-2.99091858, -0.79479508],
| [-1.23204345, -1.75224494]])
|
| rayleigh(...)
| rayleigh(scale=1.0, size=None)
|
| Draw samples from a Rayleigh distribution.
|
| The :math:`\chi` and Weibull distributions are generalizations of the
| Rayleigh.
|
| Parameters
| ----------
| scale : float or array_like of floats, optional
| Scale, also equals the mode. Must be non-negative. Default is 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``scale`` is a scalar. Otherwise,
| ``np.array(scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Rayleigh distribution.
|
| Notes
| -----
| The probability density function for the Rayleigh distribution is
|
| .. math:: P(x;scale) = \frac{x}{scale^2}e^{\frac{-x^2}{2 \cdotp scale^2}}
|
| The Rayleigh distribution would arise, for example, if the East
| and North components of the wind velocity had identical zero-mean
| Gaussian distributions. Then the wind speed would have a Rayleigh
| distribution.
|
| References
| ----------
| .. [1] Brighton Webs Ltd., "Rayleigh Distribution,"
| https://web.archive.org/web/20090514091424/http://brighton-webs.co.uk:80/distributions/rayleigh.asp
| .. [2] Wikipedia, "Rayleigh distribution"
| https://en.wikipedia.org/wiki/Rayleigh_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram
|
| >>> from matplotlib.pyplot import hist
| >>> rng = np.random.default_rng()
| >>> values = hist(rng.rayleigh(3, 100000), bins=200, density=True)
|
| Wave heights tend to follow a Rayleigh distribution. If the mean wave
| height is 1 meter, what fraction of waves are likely to be larger than 3
| meters?
|
| >>> meanvalue = 1
| >>> modevalue = np.sqrt(2 / np.pi) * meanvalue
| >>> s = rng.rayleigh(modevalue, 1000000)
|
| The percentage of waves larger than 3 meters is:
|
| >>> 100.*sum(s>3)/1000000.
| 0.087300000000000003 # random
|
| shuffle(...)
| shuffle(x, axis=0)
|
| Modify an array or sequence in-place by shuffling its contents.
|
| The order of sub-arrays is changed but their contents remains the same.
|
| Parameters
| ----------
| x : ndarray or MutableSequence
| The array, list or mutable sequence to be shuffled.
| axis : int, optional
| The axis which `x` is shuffled along. Default is 0.
| It is only supported on `ndarray` objects.
|
| Returns
| -------
| None
|
| See Also
| --------
| permuted
| permutation
|
| Notes
| -----
| An important distinction between methods ``shuffle`` and ``permuted`` is
| how they both treat the ``axis`` parameter which can be found at
| :ref:`generator-handling-axis-parameter`.
|
| Examples
| --------
| >>> rng = np.random.default_rng()
| >>> arr = np.arange(10)
| >>> arr
| array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
| >>> rng.shuffle(arr)
| >>> arr
| array([2, 0, 7, 5, 1, 4, 8, 9, 3, 6]) # random
|
| >>> arr = np.arange(9).reshape((3, 3))
| >>> arr
| array([[0, 1, 2],
| [3, 4, 5],
| [6, 7, 8]])
| >>> rng.shuffle(arr)
| >>> arr
| array([[3, 4, 5], # random
| [6, 7, 8],
| [0, 1, 2]])
|
| >>> arr = np.arange(9).reshape((3, 3))
| >>> arr
| array([[0, 1, 2],
| [3, 4, 5],
| [6, 7, 8]])
| >>> rng.shuffle(arr, axis=1)
| >>> arr
| array([[2, 0, 1], # random
| [5, 3, 4],
| [8, 6, 7]])
|
| spawn(...)
| spawn(n_children)
|
| Create new independent child generators.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children.
|
| .. versionadded:: 1.25.0
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| child_generators : list of Generators
|
| Raises
| ------
| TypeError
| When the underlying SeedSequence does not implement spawning.
|
| See Also
| --------
| random.BitGenerator.spawn, random.SeedSequence.spawn :
| Equivalent method on the bit generator and seed sequence.
| bit_generator :
| The bit generator instance used by the generator.
|
| Examples
| --------
| Starting from a seeded default generator:
|
| >>> # High quality entropy created with: f"0x{secrets.randbits(128):x}"
| >>> entropy = 0x3034c61a9ae04ff8cb62ab8ec2c4b501
| >>> rng = np.random.default_rng(entropy)
|
| Create two new generators for example for parallel execution:
|
| >>> child_rng1, child_rng2 = rng.spawn(2)
|
| Drawn numbers from each are independent but derived from the initial
| seeding entropy:
|
| >>> rng.uniform(), child_rng1.uniform(), child_rng2.uniform()
| (0.19029263503854454, 0.9475673279178444, 0.4702687338396767)
|
| It is safe to spawn additional children from the original ``rng`` or
| the children:
|
| >>> more_child_rngs = rng.spawn(20)
| >>> nested_spawn = child_rng1.spawn(20)
|
| standard_cauchy(...)
| standard_cauchy(size=None)
|
| Draw samples from a standard Cauchy distribution with mode = 0.
|
| Also known as the Lorentz distribution.
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| samples : ndarray or scalar
| The drawn samples.
|
| Notes
| -----
| The probability density function for the full Cauchy distribution is
|
| .. math:: P(x; x_0, \gamma) = \frac{1}{\pi \gamma \bigl[ 1+
| (\frac{x-x_0}{\gamma})^2 \bigr] }
|
| and the Standard Cauchy distribution just sets :math:`x_0=0` and
| :math:`\gamma=1`
|
| The Cauchy distribution arises in the solution to the driven harmonic
| oscillator problem, and also describes spectral line broadening. It
| also describes the distribution of values at which a line tilted at
| a random angle will cut the x axis.
|
| When studying hypothesis tests that assume normality, seeing how the
| tests perform on data from a Cauchy distribution is a good indicator of
| their sensitivity to a heavy-tailed distribution, since the Cauchy looks
| very much like a Gaussian distribution, but with heavier tails.
|
| References
| ----------
| .. [1] NIST/SEMATECH e-Handbook of Statistical Methods, "Cauchy
| Distribution",
| https://www.itl.nist.gov/div898/handbook/eda/section3/eda3663.htm
| .. [2] Weisstein, Eric W. "Cauchy Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/CauchyDistribution.html
| .. [3] Wikipedia, "Cauchy distribution"
| https://en.wikipedia.org/wiki/Cauchy_distribution
|
| Examples
| --------
| Draw samples and plot the distribution:
|
| >>> import matplotlib.pyplot as plt
| >>> s = np.random.default_rng().standard_cauchy(1000000)
| >>> s = s[(s>-25) & (s<25)] # truncate distribution so it plots well
| >>> plt.hist(s, bins=100)
| >>> plt.show()
|
| standard_exponential(...)
| standard_exponential(size=None, dtype=np.float64, method='zig', out=None)
|
| Draw samples from the standard exponential distribution.
|
| `standard_exponential` is identical to the exponential distribution
| with a scale parameter of 1.
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| dtype : dtype, optional
| Desired dtype of the result, only `float64` and `float32` are supported.
| Byteorder must be native. The default value is np.float64.
| method : str, optional
| Either 'inv' or 'zig'. 'inv' uses the default inverse CDF method.
| 'zig' uses the much faster Ziggurat method of Marsaglia and Tsang.
| out : ndarray, optional
| Alternative output array in which to place the result. If size is not None,
| it must have the same shape as the provided size and must match the type of
| the output values.
|
| Returns
| -------
| out : float or ndarray
| Drawn samples.
|
| Examples
| --------
| Output a 3x8000 array:
|
| >>> n = np.random.default_rng().standard_exponential((3, 8000))
|
| standard_gamma(...)
| standard_gamma(shape, size=None, dtype=np.float64, out=None)
|
| Draw samples from a standard Gamma distribution.
|
| Samples are drawn from a Gamma distribution with specified parameters,
| shape (sometimes designated "k") and scale=1.
|
| Parameters
| ----------
| shape : float or array_like of floats
| Parameter, must be non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``shape`` is a scalar. Otherwise,
| ``np.array(shape).size`` samples are drawn.
| dtype : dtype, optional
| Desired dtype of the result, only `float64` and `float32` are supported.
| Byteorder must be native. The default value is np.float64.
| out : ndarray, optional
| Alternative output array in which to place the result. If size is
| not None, it must have the same shape as the provided size and
| must match the type of the output values.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized standard gamma distribution.
|
| See Also
| --------
| scipy.stats.gamma : probability density function, distribution or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the Gamma distribution is
|
| .. math:: p(x) = x^{k-1}\frac{e^{-x/\theta}}{\theta^k\Gamma(k)},
|
| where :math:`k` is the shape and :math:`\theta` the scale,
| and :math:`\Gamma` is the Gamma function.
|
| The Gamma distribution is often used to model the times to failure of
| electronic components, and arises naturally in processes for which the
| waiting times between Poisson distributed events are relevant.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Gamma Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/GammaDistribution.html
| .. [2] Wikipedia, "Gamma distribution",
| https://en.wikipedia.org/wiki/Gamma_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> shape, scale = 2., 1. # mean and width
| >>> s = np.random.default_rng().standard_gamma(shape, 1000000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> import scipy.special as sps # doctest: +SKIP
| >>> count, bins, ignored = plt.hist(s, 50, density=True)
| >>> y = bins**(shape-1) * ((np.exp(-bins/scale))/ # doctest: +SKIP
| ... (sps.gamma(shape) * scale**shape))
| >>> plt.plot(bins, y, linewidth=2, color='r') # doctest: +SKIP
| >>> plt.show()
|
| standard_normal(...)
| standard_normal(size=None, dtype=np.float64, out=None)
|
| Draw samples from a standard Normal distribution (mean=0, stdev=1).
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| dtype : dtype, optional
| Desired dtype of the result, only `float64` and `float32` are supported.
| Byteorder must be native. The default value is np.float64.
| out : ndarray, optional
| Alternative output array in which to place the result. If size is not None,
| it must have the same shape as the provided size and must match the type of
| the output values.
|
| Returns
| -------
| out : float or ndarray
| A floating-point array of shape ``size`` of drawn samples, or a
| single sample if ``size`` was not specified.
|
| See Also
| --------
| normal :
| Equivalent function with additional ``loc`` and ``scale`` arguments
| for setting the mean and standard deviation.
|
| Notes
| -----
| For random samples from the normal distribution with mean ``mu`` and
| standard deviation ``sigma``, use one of::
|
| mu + sigma * rng.standard_normal(size=...)
| rng.normal(mu, sigma, size=...)
|
| Examples
| --------
| >>> rng = np.random.default_rng()
| >>> rng.standard_normal()
| 2.1923875335537315 # random
|
| >>> s = rng.standard_normal(8000)
| >>> s
| array([ 0.6888893 , 0.78096262, -0.89086505, ..., 0.49876311, # random
| -0.38672696, -0.4685006 ]) # random
| >>> s.shape
| (8000,)
| >>> s = rng.standard_normal(size=(3, 4, 2))
| >>> s.shape
| (3, 4, 2)
|
| Two-by-four array of samples from the normal distribution with
| mean 3 and standard deviation 2.5:
|
| >>> 3 + 2.5 * rng.standard_normal(size=(2, 4))
| array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
| [ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
|
| standard_t(...)
| standard_t(df, size=None)
|
| Draw samples from a standard Student's t distribution with `df` degrees
| of freedom.
|
| A special case of the hyperbolic distribution. As `df` gets
| large, the result resembles that of the standard normal
| distribution (`standard_normal`).
|
| Parameters
| ----------
| df : float or array_like of floats
| Degrees of freedom, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``df`` is a scalar. Otherwise,
| ``np.array(df).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized standard Student's t distribution.
|
| Notes
| -----
| The probability density function for the t distribution is
|
| .. math:: P(x, df) = \frac{\Gamma(\frac{df+1}{2})}{\sqrt{\pi df}
| \Gamma(\frac{df}{2})}\Bigl( 1+\frac{x^2}{df} \Bigr)^{-(df+1)/2}
|
| The t test is based on an assumption that the data come from a
| Normal distribution. The t test provides a way to test whether
| the sample mean (that is the mean calculated from the data) is
| a good estimate of the true mean.
|
| The derivation of the t-distribution was first published in
| 1908 by William Gosset while working for the Guinness Brewery
| in Dublin. Due to proprietary issues, he had to publish under
| a pseudonym, and so he used the name Student.
|
| References
| ----------
| .. [1] Dalgaard, Peter, "Introductory Statistics With R",
| Springer, 2002.
| .. [2] Wikipedia, "Student's t-distribution"
| https://en.wikipedia.org/wiki/Student's_t-distribution
|
| Examples
| --------
| From Dalgaard page 83 [1]_, suppose the daily energy intake for 11
| women in kilojoules (kJ) is:
|
| >>> intake = np.array([5260., 5470, 5640, 6180, 6390, 6515, 6805, 7515, \
| ... 7515, 8230, 8770])
|
| Does their energy intake deviate systematically from the recommended
| value of 7725 kJ? Our null hypothesis will be the absence of deviation,
| and the alternate hypothesis will be the presence of an effect that could be
| either positive or negative, hence making our test 2-tailed.
|
| Because we are estimating the mean and we have N=11 values in our sample,
| we have N-1=10 degrees of freedom. We set our significance level to 95% and
| compute the t statistic using the empirical mean and empirical standard
| deviation of our intake. We use a ddof of 1 to base the computation of our
| empirical standard deviation on an unbiased estimate of the variance (note:
| the final estimate is not unbiased due to the concave nature of the square
| root).
|
| >>> np.mean(intake)
| 6753.636363636364
| >>> intake.std(ddof=1)
| 1142.1232221373727
| >>> t = (np.mean(intake)-7725)/(intake.std(ddof=1)/np.sqrt(len(intake)))
| >>> t
| -2.8207540608310198
|
| We draw 1000000 samples from Student's t distribution with the adequate
| degrees of freedom.
|
| >>> import matplotlib.pyplot as plt
| >>> s = np.random.default_rng().standard_t(10, size=1000000)
| >>> h = plt.hist(s, bins=100, density=True)
|
| Does our t statistic land in one of the two critical regions found at
| both tails of the distribution?
|
| >>> np.sum(np.abs(t) < np.abs(s)) / float(len(s))
| 0.018318 #random < 0.05, statistic is in critical region
|
| The probability value for this 2-tailed test is about 1.83%, which is
| lower than the 5% pre-determined significance threshold.
|
| Therefore, the probability of observing values as extreme as our intake
| conditionally on the null hypothesis being true is too low, and we reject
| the null hypothesis of no deviation.
|
| triangular(...)
| triangular(left, mode, right, size=None)
|
| Draw samples from the triangular distribution over the
| interval ``[left, right]``.
|
| The triangular distribution is a continuous probability
| distribution with lower limit left, peak at mode, and upper
| limit right. Unlike the other distributions, these parameters
| directly define the shape of the pdf.
|
| Parameters
| ----------
| left : float or array_like of floats
| Lower limit.
| mode : float or array_like of floats
| The value where the peak of the distribution occurs.
| The value must fulfill the condition ``left <= mode <= right``.
| right : float or array_like of floats
| Upper limit, must be larger than `left`.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``left``, ``mode``, and ``right``
| are all scalars. Otherwise, ``np.broadcast(left, mode, right).size``
| samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized triangular distribution.
|
| Notes
| -----
| The probability density function for the triangular distribution is
|
| .. math:: P(x;l, m, r) = \begin{cases}
| \frac{2(x-l)}{(r-l)(m-l)}& \text{for $l \leq x \leq m$},\\
| \frac{2(r-x)}{(r-l)(r-m)}& \text{for $m \leq x \leq r$},\\
| 0& \text{otherwise}.
| \end{cases}
|
| The triangular distribution is often used in ill-defined
| problems where the underlying distribution is not known, but
| some knowledge of the limits and mode exists. Often it is used
| in simulations.
|
| References
| ----------
| .. [1] Wikipedia, "Triangular distribution"
| https://en.wikipedia.org/wiki/Triangular_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram:
|
| >>> import matplotlib.pyplot as plt
| >>> h = plt.hist(np.random.default_rng().triangular(-3, 0, 8, 100000), bins=200,
| ... density=True)
| >>> plt.show()
|
| uniform(...)
| uniform(low=0.0, high=1.0, size=None)
|
| Draw samples from a uniform distribution.
|
| Samples are uniformly distributed over the half-open interval
| ``[low, high)`` (includes low, but excludes high). In other words,
| any value within the given interval is equally likely to be drawn
| by `uniform`.
|
| Parameters
| ----------
| low : float or array_like of floats, optional
| Lower boundary of the output interval. All values generated will be
| greater than or equal to low. The default value is 0.
| high : float or array_like of floats
| Upper boundary of the output interval. All values generated will be
| less than high. The high limit may be included in the returned array of
| floats due to floating-point rounding in the equation
| ``low + (high-low) * random_sample()``. high - low must be
| non-negative. The default value is 1.0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``low`` and ``high`` are both scalars.
| Otherwise, ``np.broadcast(low, high).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized uniform distribution.
|
| See Also
| --------
| integers : Discrete uniform distribution, yielding integers.
| random : Floats uniformly distributed over ``[0, 1)``.
|
| Notes
| -----
| The probability density function of the uniform distribution is
|
| .. math:: p(x) = \frac{1}{b - a}
|
| anywhere within the interval ``[a, b)``, and zero elsewhere.
|
| When ``high`` == ``low``, values of ``low`` will be returned.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> s = np.random.default_rng().uniform(-1,0,1000)
|
| All values are within the given interval:
|
| >>> np.all(s >= -1)
| True
| >>> np.all(s < 0)
| True
|
| Display the histogram of the samples, along with the
| probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 15, density=True)
| >>> plt.plot(bins, np.ones_like(bins), linewidth=2, color='r')
| >>> plt.show()
|
| vonmises(...)
| vonmises(mu, kappa, size=None)
|
| Draw samples from a von Mises distribution.
|
| Samples are drawn from a von Mises distribution with specified mode
| (mu) and dispersion (kappa), on the interval [-pi, pi].
|
| The von Mises distribution (also known as the circular normal
| distribution) is a continuous probability distribution on the unit
| circle. It may be thought of as the circular analogue of the normal
| distribution.
|
| Parameters
| ----------
| mu : float or array_like of floats
| Mode ("center") of the distribution.
| kappa : float or array_like of floats
| Dispersion of the distribution, has to be >=0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``mu`` and ``kappa`` are both scalars.
| Otherwise, ``np.broadcast(mu, kappa).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized von Mises distribution.
|
| See Also
| --------
| scipy.stats.vonmises : probability density function, distribution, or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the von Mises distribution is
|
| .. math:: p(x) = \frac{e^{\kappa cos(x-\mu)}}{2\pi I_0(\kappa)},
|
| where :math:`\mu` is the mode and :math:`\kappa` the dispersion,
| and :math:`I_0(\kappa)` is the modified Bessel function of order 0.
|
| The von Mises is named for Richard Edler von Mises, who was born in
| Austria-Hungary, in what is now the Ukraine. He fled to the United
| States in 1939 and became a professor at Harvard. He worked in
| probability theory, aerodynamics, fluid mechanics, and philosophy of
| science.
|
| References
| ----------
| .. [1] Abramowitz, M. and Stegun, I. A. (Eds.). "Handbook of
| Mathematical Functions with Formulas, Graphs, and Mathematical
| Tables, 9th printing," New York: Dover, 1972.
| .. [2] von Mises, R., "Mathematical Theory of Probability
| and Statistics", New York: Academic Press, 1964.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> mu, kappa = 0.0, 4.0 # mean and dispersion
| >>> s = np.random.default_rng().vonmises(mu, kappa, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> from scipy.special import i0 # doctest: +SKIP
| >>> plt.hist(s, 50, density=True)
| >>> x = np.linspace(-np.pi, np.pi, num=51)
| >>> y = np.exp(kappa*np.cos(x-mu))/(2*np.pi*i0(kappa)) # doctest: +SKIP
| >>> plt.plot(x, y, linewidth=2, color='r') # doctest: +SKIP
| >>> plt.show()
|
| wald(...)
| wald(mean, scale, size=None)
|
| Draw samples from a Wald, or inverse Gaussian, distribution.
|
| As the scale approaches infinity, the distribution becomes more like a
| Gaussian. Some references claim that the Wald is an inverse Gaussian
| with mean equal to 1, but this is by no means universal.
|
| The inverse Gaussian distribution was first studied in relationship to
| Brownian motion. In 1956 M.C.K. Tweedie used the name inverse Gaussian
| because there is an inverse relationship between the time to cover a
| unit distance and distance covered in unit time.
|
| Parameters
| ----------
| mean : float or array_like of floats
| Distribution mean, must be > 0.
| scale : float or array_like of floats
| Scale parameter, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``mean`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(mean, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Wald distribution.
|
| Notes
| -----
| The probability density function for the Wald distribution is
|
| .. math:: P(x;mean,scale) = \sqrt{\frac{scale}{2\pi x^3}}e^
| \frac{-scale(x-mean)^2}{2\cdotp mean^2x}
|
| As noted above the inverse Gaussian distribution first arise
| from attempts to model Brownian motion. It is also a
| competitor to the Weibull for use in reliability modeling and
| modeling stock returns and interest rate processes.
|
| References
| ----------
| .. [1] Brighton Webs Ltd., Wald Distribution,
| https://web.archive.org/web/20090423014010/http://www.brighton-webs.co.uk:80/distributions/wald.asp
| .. [2] Chhikara, Raj S., and Folks, J. Leroy, "The Inverse Gaussian
| Distribution: Theory : Methodology, and Applications", CRC Press,
| 1988.
| .. [3] Wikipedia, "Inverse Gaussian distribution"
| https://en.wikipedia.org/wiki/Inverse_Gaussian_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram:
|
| >>> import matplotlib.pyplot as plt
| >>> h = plt.hist(np.random.default_rng().wald(3, 2, 100000), bins=200, density=True)
| >>> plt.show()
|
| weibull(...)
| weibull(a, size=None)
|
| Draw samples from a Weibull distribution.
|
| Draw samples from a 1-parameter Weibull distribution with the given
| shape parameter `a`.
|
| .. math:: X = (-ln(U))^{1/a}
|
| Here, U is drawn from the uniform distribution over (0,1].
|
| The more common 2-parameter Weibull, including a scale parameter
| :math:`\lambda` is just :math:`X = \lambda(-ln(U))^{1/a}`.
|
| Parameters
| ----------
| a : float or array_like of floats
| Shape parameter of the distribution. Must be nonnegative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Weibull distribution.
|
| See Also
| --------
| scipy.stats.weibull_max
| scipy.stats.weibull_min
| scipy.stats.genextreme
| gumbel
|
| Notes
| -----
| The Weibull (or Type III asymptotic extreme value distribution
| for smallest values, SEV Type III, or Rosin-Rammler
| distribution) is one of a class of Generalized Extreme Value
| (GEV) distributions used in modeling extreme value problems.
| This class includes the Gumbel and Frechet distributions.
|
| The probability density for the Weibull distribution is
|
| .. math:: p(x) = \frac{a}
| {\lambda}(\frac{x}{\lambda})^{a-1}e^{-(x/\lambda)^a},
|
| where :math:`a` is the shape and :math:`\lambda` the scale.
|
| The function has its peak (the mode) at
| :math:`\lambda(\frac{a-1}{a})^{1/a}`.
|
| When ``a = 1``, the Weibull distribution reduces to the exponential
| distribution.
|
| References
| ----------
| .. [1] Waloddi Weibull, Royal Technical University, Stockholm,
| 1939 "A Statistical Theory Of The Strength Of Materials",
| Ingeniorsvetenskapsakademiens Handlingar Nr 151, 1939,
| Generalstabens Litografiska Anstalts Forlag, Stockholm.
| .. [2] Waloddi Weibull, "A Statistical Distribution Function of
| Wide Applicability", Journal Of Applied Mechanics ASME Paper
| 1951.
| .. [3] Wikipedia, "Weibull distribution",
| https://en.wikipedia.org/wiki/Weibull_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> rng = np.random.default_rng()
| >>> a = 5. # shape
| >>> s = rng.weibull(a, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> x = np.arange(1,100.)/50.
| >>> def weib(x,n,a):
| ... return (a / n) * (x / n)**(a - 1) * np.exp(-(x / n)**a)
|
| >>> count, bins, ignored = plt.hist(rng.weibull(5.,1000))
| >>> x = np.arange(1,100.)/50.
| >>> scale = count.max()/weib(x, 1., 5.).max()
| >>> plt.plot(x, weib(x, 1., 5.)*scale)
| >>> plt.show()
|
| zipf(...)
| zipf(a, size=None)
|
| Draw samples from a Zipf distribution.
|
| Samples are drawn from a Zipf distribution with specified parameter
| `a` > 1.
|
| The Zipf distribution (also known as the zeta distribution) is a
| discrete probability distribution that satisfies Zipf's law: the
| frequency of an item is inversely proportional to its rank in a
| frequency table.
|
| Parameters
| ----------
| a : float or array_like of floats
| Distribution parameter. Must be greater than 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Zipf distribution.
|
| See Also
| --------
| scipy.stats.zipf : probability density function, distribution, or
| cumulative density function, etc.
|
| Notes
| -----
| The probability density for the Zipf distribution is
|
| .. math:: p(k) = \frac{k^{-a}}{\zeta(a)},
|
| for integers :math:`k \geq 1`, where :math:`\zeta` is the Riemann Zeta
| function.
|
| It is named for the American linguist George Kingsley Zipf, who noted
| that the frequency of any word in a sample of a language is inversely
| proportional to its rank in the frequency table.
|
| References
| ----------
| .. [1] Zipf, G. K., "Selected Studies of the Principle of Relative
| Frequency in Language," Cambridge, MA: Harvard Univ. Press,
| 1932.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a = 4.0
| >>> n = 20000
| >>> s = np.random.default_rng().zipf(a, size=n)
|
| Display the histogram of the samples, along with
| the expected histogram based on the probability
| density function:
|
| >>> import matplotlib.pyplot as plt
| >>> from scipy.special import zeta # doctest: +SKIP
|
| `bincount` provides a fast histogram for small integers.
|
| >>> count = np.bincount(s)
| >>> k = np.arange(1, s.max() + 1)
|
| >>> plt.bar(k, count[1:], alpha=0.5, label='sample count')
| >>> plt.plot(k, n*(k**-a)/zeta(a), 'k.-', alpha=0.5,
| ... label='expected count') # doctest: +SKIP
| >>> plt.semilogy()
| >>> plt.grid(alpha=0.4)
| >>> plt.legend()
| >>> plt.title(f'Zipf sample, a={a}, size={n}')
| >>> plt.show()
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| bit_generator
| Gets the bit generator instance used by the generator
|
| Returns
| -------
| bit_generator : BitGenerator
| The bit generator instance used by the generator
class MT19937(numpy.random.bit_generator.BitGenerator)
| MT19937(seed=None)
|
| Container for the Mersenne Twister pseudo-random number generator.
|
| Parameters
| ----------
| seed : {None, int, array_like[ints], SeedSequence}, optional
| A seed to initialize the `BitGenerator`. If None, then fresh,
| unpredictable entropy will be pulled from the OS. If an ``int`` or
| ``array_like[ints]`` is passed, then it will be passed to
| `SeedSequence` to derive the initial `BitGenerator` state. One may also
| pass in a `SeedSequence` instance.
|
| Attributes
| ----------
| lock: threading.Lock
| Lock instance that is shared so that the same bit git generator can
| be used in multiple Generators without corrupting the state. Code that
| generates values from a bit generator should hold the bit generator's
| lock.
|
| Notes
| -----
| ``MT19937`` provides a capsule containing function pointers that produce
| doubles, and unsigned 32 and 64- bit integers [1]_. These are not
| directly consumable in Python and must be consumed by a ``Generator``
| or similar object that supports low-level access.
|
| The Python stdlib module "random" also contains a Mersenne Twister
| pseudo-random number generator.
|
| **State and Seeding**
|
| The ``MT19937`` state vector consists of a 624-element array of
| 32-bit unsigned integers plus a single integer value between 0 and 624
| that indexes the current position within the main array.
|
| The input seed is processed by `SeedSequence` to fill the whole state. The
| first element is reset such that only its most significant bit is set.
|
| **Parallel Features**
|
| The preferred way to use a BitGenerator in parallel applications is to use
| the `SeedSequence.spawn` method to obtain entropy values, and to use these
| to generate new BitGenerators:
|
| >>> from numpy.random import Generator, MT19937, SeedSequence
| >>> sg = SeedSequence(1234)
| >>> rg = [Generator(MT19937(s)) for s in sg.spawn(10)]
|
| Another method is to use `MT19937.jumped` which advances the state as-if
| :math:`2^{128}` random numbers have been generated ([1]_, [2]_). This
| allows the original sequence to be split so that distinct segments can be
| used in each worker process. All generators should be chained to ensure
| that the segments come from the same sequence.
|
| >>> from numpy.random import Generator, MT19937, SeedSequence
| >>> sg = SeedSequence(1234)
| >>> bit_generator = MT19937(sg)
| >>> rg = []
| >>> for _ in range(10):
| ... rg.append(Generator(bit_generator))
| ... # Chain the BitGenerators
| ... bit_generator = bit_generator.jumped()
|
| **Compatibility Guarantee**
|
| ``MT19937`` makes a guarantee that a fixed seed will always produce
| the same random integer stream.
|
| References
| ----------
| .. [1] Hiroshi Haramoto, Makoto Matsumoto, and Pierre L'Ecuyer, "A Fast
| Jump Ahead Algorithm for Linear Recurrences in a Polynomial Space",
| Sequences and Their Applications - SETA, 290--298, 2008.
| .. [2] Hiroshi Haramoto, Makoto Matsumoto, Takuji Nishimura, François
| Panneton, Pierre L'Ecuyer, "Efficient Jump Ahead for F2-Linear
| Random Number Generators", INFORMS JOURNAL ON COMPUTING, Vol. 20,
| No. 3, Summer 2008, pp. 385-390.
|
| Method resolution order:
| MT19937
| numpy.random.bit_generator.BitGenerator
| builtins.object
|
| Methods defined here:
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce_cython__(...)
|
| __setstate_cython__(...)
|
| jumped(...)
| jumped(jumps=1)
|
| Returns a new bit generator with the state jumped
|
| The state of the returned bit generator is jumped as-if
| 2**(128 * jumps) random numbers have been generated.
|
| Parameters
| ----------
| jumps : integer, positive
| Number of times to jump the state of the bit generator returned
|
| Returns
| -------
| bit_generator : MT19937
| New instance of generator jumped iter times
|
| Notes
| -----
| The jump step is computed using a modified version of Matsumoto's
| implementation of Horner's method. The step polynomial is precomputed
| to perform 2**128 steps. The jumped state has been verified to match
| the state produced using Matsumoto's original code.
|
| References
| ----------
| .. [1] Matsumoto, M, Generating multiple disjoint streams of
| pseudorandom number sequences. Accessed on: May 6, 2020.
| http://www.math.sci.hiroshima-u.ac.jp/~m-mat/MT/JUMP/
| .. [2] Hiroshi Haramoto, Makoto Matsumoto, Takuji Nishimura, François
| Panneton, Pierre L'Ecuyer, "Efficient Jump Ahead for F2-Linear
| Random Number Generators", INFORMS JOURNAL ON COMPUTING, Vol. 20,
| No. 3, Summer 2008, pp. 385-390.
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| state
| Get or set the PRNG state
|
| Returns
| -------
| state : dict
| Dictionary containing the information required to describe the
| state of the PRNG
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| __pyx_vtable__ = <capsule object NULL>
|
| ----------------------------------------------------------------------
| Methods inherited from numpy.random.bit_generator.BitGenerator:
|
| __getstate__(...)
| Helper for pickle.
|
| __reduce__(...)
| Helper for pickle.
|
| __setstate__(...)
|
| random_raw(...)
| random_raw(self, size=None)
|
| Return randoms as generated by the underlying BitGenerator
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| output : bool, optional
| Output values. Used for performance testing since the generated
| values are not returned.
|
| Returns
| -------
| out : uint or ndarray
| Drawn samples.
|
| Notes
| -----
| This method directly exposes the raw underlying pseudo-random
| number generator. All values are returned as unsigned 64-bit
| values irrespective of the number of bits produced by the PRNG.
|
| See the class docstring for the number of bits returned.
|
| spawn(...)
| spawn(n_children)
|
| Create new independent child bit generators.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children. Some bit generators also implement ``jumped``
| as a different approach for creating independent streams.
|
| .. versionadded:: 1.25.0
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| child_bit_generators : list of BitGenerators
|
| Raises
| ------
| TypeError
| When the underlying SeedSequence does not implement spawning.
|
| See Also
| --------
| random.Generator.spawn, random.SeedSequence.spawn :
| Equivalent method on the generator and seed sequence.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from numpy.random.bit_generator.BitGenerator:
|
| capsule
|
| cffi
| CFFI interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing CFFI wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| ctypes
| ctypes interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing ctypes wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| lock
|
| seed_seq
| Get the seed sequence used to initialize the bit generator.
|
| .. versionadded:: 1.25.0
|
| Returns
| -------
| seed_seq : ISeedSequence
| The SeedSequence object used to initialize the BitGenerator.
| This is normally a `np.random.SeedSequence` instance.
class PCG64(numpy.random.bit_generator.BitGenerator)
| PCG64(seed=None)
|
| BitGenerator for the PCG-64 pseudo-random number generator.
|
| Parameters
| ----------
| seed : {None, int, array_like[ints], SeedSequence}, optional
| A seed to initialize the `BitGenerator`. If None, then fresh,
| unpredictable entropy will be pulled from the OS. If an ``int`` or
| ``array_like[ints]`` is passed, then it will be passed to
| `SeedSequence` to derive the initial `BitGenerator` state. One may also
| pass in a `SeedSequence` instance.
|
| Notes
| -----
| PCG-64 is a 128-bit implementation of O'Neill's permutation congruential
| generator ([1]_, [2]_). PCG-64 has a period of :math:`2^{128}` and supports
| advancing an arbitrary number of steps as well as :math:`2^{127}` streams.
| The specific member of the PCG family that we use is PCG XSL RR 128/64
| as described in the paper ([2]_).
|
| ``PCG64`` provides a capsule containing function pointers that produce
| doubles, and unsigned 32 and 64- bit integers. These are not
| directly consumable in Python and must be consumed by a ``Generator``
| or similar object that supports low-level access.
|
| Supports the method :meth:`advance` to advance the RNG an arbitrary number of
| steps. The state of the PCG-64 RNG is represented by 2 128-bit unsigned
| integers.
|
| **State and Seeding**
|
| The ``PCG64`` state vector consists of 2 unsigned 128-bit values,
| which are represented externally as Python ints. One is the state of the
| PRNG, which is advanced by a linear congruential generator (LCG). The
| second is a fixed odd increment used in the LCG.
|
| The input seed is processed by `SeedSequence` to generate both values. The
| increment is not independently settable.
|
| **Parallel Features**
|
| The preferred way to use a BitGenerator in parallel applications is to use
| the `SeedSequence.spawn` method to obtain entropy values, and to use these
| to generate new BitGenerators:
|
| >>> from numpy.random import Generator, PCG64, SeedSequence
| >>> sg = SeedSequence(1234)
| >>> rg = [Generator(PCG64(s)) for s in sg.spawn(10)]
|
| **Compatibility Guarantee**
|
| ``PCG64`` makes a guarantee that a fixed seed will always produce
| the same random integer stream.
|
| References
| ----------
| .. [1] `"PCG, A Family of Better Random Number Generators"
| <http://www.pcg-random.org/>`_
| .. [2] O'Neill, Melissa E. `"PCG: A Family of Simple Fast Space-Efficient
| Statistically Good Algorithms for Random Number Generation"
| <https://www.cs.hmc.edu/tr/hmc-cs-2014-0905.pdf>`_
|
| Method resolution order:
| PCG64
| numpy.random.bit_generator.BitGenerator
| builtins.object
|
| Methods defined here:
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce_cython__(...)
|
| __setstate_cython__(...)
|
| advance(...)
| advance(delta)
|
| Advance the underlying RNG as-if delta draws have occurred.
|
| Parameters
| ----------
| delta : integer, positive
| Number of draws to advance the RNG. Must be less than the
| size state variable in the underlying RNG.
|
| Returns
| -------
| self : PCG64
| RNG advanced delta steps
|
| Notes
| -----
| Advancing a RNG updates the underlying RNG state as-if a given
| number of calls to the underlying RNG have been made. In general
| there is not a one-to-one relationship between the number output
| random values from a particular distribution and the number of
| draws from the core RNG. This occurs for two reasons:
|
| * The random values are simulated using a rejection-based method
| and so, on average, more than one value from the underlying
| RNG is required to generate an single draw.
| * The number of bits required to generate a simulated value
| differs from the number of bits generated by the underlying
| RNG. For example, two 16-bit integer values can be simulated
| from a single draw of a 32-bit RNG.
|
| Advancing the RNG state resets any pre-computed random numbers.
| This is required to ensure exact reproducibility.
|
| jumped(...)
| jumped(jumps=1)
|
| Returns a new bit generator with the state jumped.
|
| Jumps the state as-if jumps * 210306068529402873165736369884012333109
| random numbers have been generated.
|
| Parameters
| ----------
| jumps : integer, positive
| Number of times to jump the state of the bit generator returned
|
| Returns
| -------
| bit_generator : PCG64
| New instance of generator jumped iter times
|
| Notes
| -----
| The step size is phi-1 when multiplied by 2**128 where phi is the
| golden ratio.
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| state
| Get or set the PRNG state
|
| Returns
| -------
| state : dict
| Dictionary containing the information required to describe the
| state of the PRNG
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| __pyx_vtable__ = <capsule object NULL>
|
| ----------------------------------------------------------------------
| Methods inherited from numpy.random.bit_generator.BitGenerator:
|
| __getstate__(...)
| Helper for pickle.
|
| __reduce__(...)
| Helper for pickle.
|
| __setstate__(...)
|
| random_raw(...)
| random_raw(self, size=None)
|
| Return randoms as generated by the underlying BitGenerator
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| output : bool, optional
| Output values. Used for performance testing since the generated
| values are not returned.
|
| Returns
| -------
| out : uint or ndarray
| Drawn samples.
|
| Notes
| -----
| This method directly exposes the raw underlying pseudo-random
| number generator. All values are returned as unsigned 64-bit
| values irrespective of the number of bits produced by the PRNG.
|
| See the class docstring for the number of bits returned.
|
| spawn(...)
| spawn(n_children)
|
| Create new independent child bit generators.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children. Some bit generators also implement ``jumped``
| as a different approach for creating independent streams.
|
| .. versionadded:: 1.25.0
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| child_bit_generators : list of BitGenerators
|
| Raises
| ------
| TypeError
| When the underlying SeedSequence does not implement spawning.
|
| See Also
| --------
| random.Generator.spawn, random.SeedSequence.spawn :
| Equivalent method on the generator and seed sequence.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from numpy.random.bit_generator.BitGenerator:
|
| capsule
|
| cffi
| CFFI interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing CFFI wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| ctypes
| ctypes interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing ctypes wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| lock
|
| seed_seq
| Get the seed sequence used to initialize the bit generator.
|
| .. versionadded:: 1.25.0
|
| Returns
| -------
| seed_seq : ISeedSequence
| The SeedSequence object used to initialize the BitGenerator.
| This is normally a `np.random.SeedSequence` instance.
class PCG64DXSM(numpy.random.bit_generator.BitGenerator)
| PCG64DXSM(seed=None)
|
| BitGenerator for the PCG-64 DXSM pseudo-random number generator.
|
| Parameters
| ----------
| seed : {None, int, array_like[ints], SeedSequence}, optional
| A seed to initialize the `BitGenerator`. If None, then fresh,
| unpredictable entropy will be pulled from the OS. If an ``int`` or
| ``array_like[ints]`` is passed, then it will be passed to
| `SeedSequence` to derive the initial `BitGenerator` state. One may also
| pass in a `SeedSequence` instance.
|
| Notes
| -----
| PCG-64 DXSM is a 128-bit implementation of O'Neill's permutation congruential
| generator ([1]_, [2]_). PCG-64 DXSM has a period of :math:`2^{128}` and supports
| advancing an arbitrary number of steps as well as :math:`2^{127}` streams.
| The specific member of the PCG family that we use is PCG CM DXSM 128/64. It
| differs from ``PCG64`` in that it uses the stronger DXSM output function,
| a 64-bit "cheap multiplier" in the LCG, and outputs from the state before
| advancing it rather than advance-then-output.
|
| ``PCG64DXSM`` provides a capsule containing function pointers that produce
| doubles, and unsigned 32 and 64- bit integers. These are not
| directly consumable in Python and must be consumed by a ``Generator``
| or similar object that supports low-level access.
|
| Supports the method :meth:`advance` to advance the RNG an arbitrary number of
| steps. The state of the PCG-64 DXSM RNG is represented by 2 128-bit unsigned
| integers.
|
| **State and Seeding**
|
| The ``PCG64DXSM`` state vector consists of 2 unsigned 128-bit values,
| which are represented externally as Python ints. One is the state of the
| PRNG, which is advanced by a linear congruential generator (LCG). The
| second is a fixed odd increment used in the LCG.
|
| The input seed is processed by `SeedSequence` to generate both values. The
| increment is not independently settable.
|
| **Parallel Features**
|
| The preferred way to use a BitGenerator in parallel applications is to use
| the `SeedSequence.spawn` method to obtain entropy values, and to use these
| to generate new BitGenerators:
|
| >>> from numpy.random import Generator, PCG64DXSM, SeedSequence
| >>> sg = SeedSequence(1234)
| >>> rg = [Generator(PCG64DXSM(s)) for s in sg.spawn(10)]
|
| **Compatibility Guarantee**
|
| ``PCG64DXSM`` makes a guarantee that a fixed seed will always produce
| the same random integer stream.
|
| References
| ----------
| .. [1] `"PCG, A Family of Better Random Number Generators"
| <http://www.pcg-random.org/>`_
| .. [2] O'Neill, Melissa E. `"PCG: A Family of Simple Fast Space-Efficient
| Statistically Good Algorithms for Random Number Generation"
| <https://www.cs.hmc.edu/tr/hmc-cs-2014-0905.pdf>`_
|
| Method resolution order:
| PCG64DXSM
| numpy.random.bit_generator.BitGenerator
| builtins.object
|
| Methods defined here:
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce_cython__(...)
|
| __setstate_cython__(...)
|
| advance(...)
| advance(delta)
|
| Advance the underlying RNG as-if delta draws have occurred.
|
| Parameters
| ----------
| delta : integer, positive
| Number of draws to advance the RNG. Must be less than the
| size state variable in the underlying RNG.
|
| Returns
| -------
| self : PCG64
| RNG advanced delta steps
|
| Notes
| -----
| Advancing a RNG updates the underlying RNG state as-if a given
| number of calls to the underlying RNG have been made. In general
| there is not a one-to-one relationship between the number output
| random values from a particular distribution and the number of
| draws from the core RNG. This occurs for two reasons:
|
| * The random values are simulated using a rejection-based method
| and so, on average, more than one value from the underlying
| RNG is required to generate an single draw.
| * The number of bits required to generate a simulated value
| differs from the number of bits generated by the underlying
| RNG. For example, two 16-bit integer values can be simulated
| from a single draw of a 32-bit RNG.
|
| Advancing the RNG state resets any pre-computed random numbers.
| This is required to ensure exact reproducibility.
|
| jumped(...)
| jumped(jumps=1)
|
| Returns a new bit generator with the state jumped.
|
| Jumps the state as-if jumps * 210306068529402873165736369884012333109
| random numbers have been generated.
|
| Parameters
| ----------
| jumps : integer, positive
| Number of times to jump the state of the bit generator returned
|
| Returns
| -------
| bit_generator : PCG64DXSM
| New instance of generator jumped iter times
|
| Notes
| -----
| The step size is phi-1 when multiplied by 2**128 where phi is the
| golden ratio.
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| state
| Get or set the PRNG state
|
| Returns
| -------
| state : dict
| Dictionary containing the information required to describe the
| state of the PRNG
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| __pyx_vtable__ = <capsule object NULL>
|
| ----------------------------------------------------------------------
| Methods inherited from numpy.random.bit_generator.BitGenerator:
|
| __getstate__(...)
| Helper for pickle.
|
| __reduce__(...)
| Helper for pickle.
|
| __setstate__(...)
|
| random_raw(...)
| random_raw(self, size=None)
|
| Return randoms as generated by the underlying BitGenerator
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| output : bool, optional
| Output values. Used for performance testing since the generated
| values are not returned.
|
| Returns
| -------
| out : uint or ndarray
| Drawn samples.
|
| Notes
| -----
| This method directly exposes the raw underlying pseudo-random
| number generator. All values are returned as unsigned 64-bit
| values irrespective of the number of bits produced by the PRNG.
|
| See the class docstring for the number of bits returned.
|
| spawn(...)
| spawn(n_children)
|
| Create new independent child bit generators.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children. Some bit generators also implement ``jumped``
| as a different approach for creating independent streams.
|
| .. versionadded:: 1.25.0
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| child_bit_generators : list of BitGenerators
|
| Raises
| ------
| TypeError
| When the underlying SeedSequence does not implement spawning.
|
| See Also
| --------
| random.Generator.spawn, random.SeedSequence.spawn :
| Equivalent method on the generator and seed sequence.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from numpy.random.bit_generator.BitGenerator:
|
| capsule
|
| cffi
| CFFI interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing CFFI wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| ctypes
| ctypes interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing ctypes wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| lock
|
| seed_seq
| Get the seed sequence used to initialize the bit generator.
|
| .. versionadded:: 1.25.0
|
| Returns
| -------
| seed_seq : ISeedSequence
| The SeedSequence object used to initialize the BitGenerator.
| This is normally a `np.random.SeedSequence` instance.
class Philox(numpy.random.bit_generator.BitGenerator)
| Philox(seed=None, counter=None, key=None)
|
| Container for the Philox (4x64) pseudo-random number generator.
|
| Parameters
| ----------
| seed : {None, int, array_like[ints], SeedSequence}, optional
| A seed to initialize the `BitGenerator`. If None, then fresh,
| unpredictable entropy will be pulled from the OS. If an ``int`` or
| ``array_like[ints]`` is passed, then it will be passed to
| `SeedSequence` to derive the initial `BitGenerator` state. One may also
| pass in a `SeedSequence` instance.
| counter : {None, int, array_like}, optional
| Counter to use in the Philox state. Can be either
| a Python int (long in 2.x) in [0, 2**256) or a 4-element uint64 array.
| If not provided, the RNG is initialized at 0.
| key : {None, int, array_like}, optional
| Key to use in the Philox state. Unlike ``seed``, the value in key is
| directly set. Can be either a Python int in [0, 2**128) or a 2-element
| uint64 array. `key` and ``seed`` cannot both be used.
|
| Attributes
| ----------
| lock: threading.Lock
| Lock instance that is shared so that the same bit git generator can
| be used in multiple Generators without corrupting the state. Code that
| generates values from a bit generator should hold the bit generator's
| lock.
|
| Notes
| -----
| Philox is a 64-bit PRNG that uses a counter-based design based on weaker
| (and faster) versions of cryptographic functions [1]_. Instances using
| different values of the key produce independent sequences. Philox has a
| period of :math:`2^{256} - 1` and supports arbitrary advancing and jumping
| the sequence in increments of :math:`2^{128}`. These features allow
| multiple non-overlapping sequences to be generated.
|
| ``Philox`` provides a capsule containing function pointers that produce
| doubles, and unsigned 32 and 64- bit integers. These are not
| directly consumable in Python and must be consumed by a ``Generator``
| or similar object that supports low-level access.
|
| **State and Seeding**
|
| The ``Philox`` state vector consists of a 256-bit value encoded as
| a 4-element uint64 array and a 128-bit value encoded as a 2-element uint64
| array. The former is a counter which is incremented by 1 for every 4 64-bit
| randoms produced. The second is a key which determined the sequence
| produced. Using different keys produces independent sequences.
|
| The input ``seed`` is processed by `SeedSequence` to generate the key. The
| counter is set to 0.
|
| Alternately, one can omit the ``seed`` parameter and set the ``key`` and
| ``counter`` directly.
|
| **Parallel Features**
|
| The preferred way to use a BitGenerator in parallel applications is to use
| the `SeedSequence.spawn` method to obtain entropy values, and to use these
| to generate new BitGenerators:
|
| >>> from numpy.random import Generator, Philox, SeedSequence
| >>> sg = SeedSequence(1234)
| >>> rg = [Generator(Philox(s)) for s in sg.spawn(10)]
|
| ``Philox`` can be used in parallel applications by calling the ``jumped``
| method to advances the state as-if :math:`2^{128}` random numbers have
| been generated. Alternatively, ``advance`` can be used to advance the
| counter for any positive step in [0, 2**256). When using ``jumped``, all
| generators should be chained to ensure that the segments come from the same
| sequence.
|
| >>> from numpy.random import Generator, Philox
| >>> bit_generator = Philox(1234)
| >>> rg = []
| >>> for _ in range(10):
| ... rg.append(Generator(bit_generator))
| ... bit_generator = bit_generator.jumped()
|
| Alternatively, ``Philox`` can be used in parallel applications by using
| a sequence of distinct keys where each instance uses different key.
|
| >>> key = 2**96 + 2**33 + 2**17 + 2**9
| >>> rg = [Generator(Philox(key=key+i)) for i in range(10)]
|
| **Compatibility Guarantee**
|
| ``Philox`` makes a guarantee that a fixed ``seed`` will always produce
| the same random integer stream.
|
| Examples
| --------
| >>> from numpy.random import Generator, Philox
| >>> rg = Generator(Philox(1234))
| >>> rg.standard_normal()
| 0.123 # random
|
| References
| ----------
| .. [1] John K. Salmon, Mark A. Moraes, Ron O. Dror, and David E. Shaw,
| "Parallel Random Numbers: As Easy as 1, 2, 3," Proceedings of
| the International Conference for High Performance Computing,
| Networking, Storage and Analysis (SC11), New York, NY: ACM, 2011.
|
| Method resolution order:
| Philox
| numpy.random.bit_generator.BitGenerator
| builtins.object
|
| Methods defined here:
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce_cython__(...)
|
| __setstate_cython__(...)
|
| advance(...)
| advance(delta)
|
| Advance the underlying RNG as-if delta draws have occurred.
|
| Parameters
| ----------
| delta : integer, positive
| Number of draws to advance the RNG. Must be less than the
| size state variable in the underlying RNG.
|
| Returns
| -------
| self : Philox
| RNG advanced delta steps
|
| Notes
| -----
| Advancing a RNG updates the underlying RNG state as-if a given
| number of calls to the underlying RNG have been made. In general
| there is not a one-to-one relationship between the number output
| random values from a particular distribution and the number of
| draws from the core RNG. This occurs for two reasons:
|
| * The random values are simulated using a rejection-based method
| and so, on average, more than one value from the underlying
| RNG is required to generate an single draw.
| * The number of bits required to generate a simulated value
| differs from the number of bits generated by the underlying
| RNG. For example, two 16-bit integer values can be simulated
| from a single draw of a 32-bit RNG.
|
| Advancing the RNG state resets any pre-computed random numbers.
| This is required to ensure exact reproducibility.
|
| jumped(...)
| jumped(jumps=1)
|
| Returns a new bit generator with the state jumped
|
| The state of the returned bit generator is jumped as-if
| (2**128) * jumps random numbers have been generated.
|
| Parameters
| ----------
| jumps : integer, positive
| Number of times to jump the state of the bit generator returned
|
| Returns
| -------
| bit_generator : Philox
| New instance of generator jumped iter times
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| state
| Get or set the PRNG state
|
| Returns
| -------
| state : dict
| Dictionary containing the information required to describe the
| state of the PRNG
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| __pyx_vtable__ = <capsule object NULL>
|
| ----------------------------------------------------------------------
| Methods inherited from numpy.random.bit_generator.BitGenerator:
|
| __getstate__(...)
| Helper for pickle.
|
| __reduce__(...)
| Helper for pickle.
|
| __setstate__(...)
|
| random_raw(...)
| random_raw(self, size=None)
|
| Return randoms as generated by the underlying BitGenerator
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| output : bool, optional
| Output values. Used for performance testing since the generated
| values are not returned.
|
| Returns
| -------
| out : uint or ndarray
| Drawn samples.
|
| Notes
| -----
| This method directly exposes the raw underlying pseudo-random
| number generator. All values are returned as unsigned 64-bit
| values irrespective of the number of bits produced by the PRNG.
|
| See the class docstring for the number of bits returned.
|
| spawn(...)
| spawn(n_children)
|
| Create new independent child bit generators.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children. Some bit generators also implement ``jumped``
| as a different approach for creating independent streams.
|
| .. versionadded:: 1.25.0
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| child_bit_generators : list of BitGenerators
|
| Raises
| ------
| TypeError
| When the underlying SeedSequence does not implement spawning.
|
| See Also
| --------
| random.Generator.spawn, random.SeedSequence.spawn :
| Equivalent method on the generator and seed sequence.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from numpy.random.bit_generator.BitGenerator:
|
| capsule
|
| cffi
| CFFI interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing CFFI wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| ctypes
| ctypes interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing ctypes wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| lock
|
| seed_seq
| Get the seed sequence used to initialize the bit generator.
|
| .. versionadded:: 1.25.0
|
| Returns
| -------
| seed_seq : ISeedSequence
| The SeedSequence object used to initialize the BitGenerator.
| This is normally a `np.random.SeedSequence` instance.
class RandomState(builtins.object)
| RandomState(seed=None)
|
| Container for the slow Mersenne Twister pseudo-random number generator.
| Consider using a different BitGenerator with the Generator container
| instead.
|
| `RandomState` and `Generator` expose a number of methods for generating
| random numbers drawn from a variety of probability distributions. In
| addition to the distribution-specific arguments, each method takes a
| keyword argument `size` that defaults to ``None``. If `size` is ``None``,
| then a single value is generated and returned. If `size` is an integer,
| then a 1-D array filled with generated values is returned. If `size` is a
| tuple, then an array with that shape is filled and returned.
|
| **Compatibility Guarantee**
|
| A fixed bit generator using a fixed seed and a fixed series of calls to
| 'RandomState' methods using the same parameters will always produce the
| same results up to roundoff error except when the values were incorrect.
| `RandomState` is effectively frozen and will only receive updates that
| are required by changes in the internals of Numpy. More substantial
| changes, including algorithmic improvements, are reserved for
| `Generator`.
|
| Parameters
| ----------
| seed : {None, int, array_like, BitGenerator}, optional
| Random seed used to initialize the pseudo-random number generator or
| an instantized BitGenerator. If an integer or array, used as a seed for
| the MT19937 BitGenerator. Values can be any integer between 0 and
| 2**32 - 1 inclusive, an array (or other sequence) of such integers,
| or ``None`` (the default). If `seed` is ``None``, then the `MT19937`
| BitGenerator is initialized by reading data from ``/dev/urandom``
| (or the Windows analogue) if available or seed from the clock
| otherwise.
|
| Notes
| -----
| The Python stdlib module "random" also contains a Mersenne Twister
| pseudo-random number generator with a number of methods that are similar
| to the ones available in `RandomState`. `RandomState`, besides being
| NumPy-aware, has the advantage that it provides a much larger number
| of probability distributions to choose from.
|
| See Also
| --------
| Generator
| MT19937
| numpy.random.BitGenerator
|
| Methods defined here:
|
| __getstate__(...)
| Helper for pickle.
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce__(...)
| Helper for pickle.
|
| __repr__(...)
| Return repr(self).
|
| __setstate__(...)
|
| __str__(self, /)
| Return str(self).
|
| beta(...)
| beta(a, b, size=None)
|
| Draw samples from a Beta distribution.
|
| The Beta distribution is a special case of the Dirichlet distribution,
| and is related to the Gamma distribution. It has the probability
| distribution function
|
| .. math:: f(x; a,b) = \frac{1}{B(\alpha, \beta)} x^{\alpha - 1}
| (1 - x)^{\beta - 1},
|
| where the normalization, B, is the beta function,
|
| .. math:: B(\alpha, \beta) = \int_0^1 t^{\alpha - 1}
| (1 - t)^{\beta - 1} dt.
|
| It is often seen in Bayesian inference and order statistics.
|
| .. note::
| New code should use the `~numpy.random.Generator.beta`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
|
| Parameters
| ----------
| a : float or array_like of floats
| Alpha, positive (>0).
| b : float or array_like of floats
| Beta, positive (>0).
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` and ``b`` are both scalars.
| Otherwise, ``np.broadcast(a, b).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized beta distribution.
|
| See Also
| --------
| random.Generator.beta: which should be used for new code.
|
| binomial(...)
| binomial(n, p, size=None)
|
| Draw samples from a binomial distribution.
|
| Samples are drawn from a binomial distribution with specified
| parameters, n trials and p probability of success where
| n an integer >= 0 and p is in the interval [0,1]. (n may be
| input as a float, but it is truncated to an integer in use)
|
| .. note::
| New code should use the `~numpy.random.Generator.binomial`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| n : int or array_like of ints
| Parameter of the distribution, >= 0. Floats are also accepted,
| but they will be truncated to integers.
| p : float or array_like of floats
| Parameter of the distribution, >= 0 and <=1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``n`` and ``p`` are both scalars.
| Otherwise, ``np.broadcast(n, p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized binomial distribution, where
| each sample is equal to the number of successes over the n trials.
|
| See Also
| --------
| scipy.stats.binom : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.binomial: which should be used for new code.
|
| Notes
| -----
| The probability density for the binomial distribution is
|
| .. math:: P(N) = \binom{n}{N}p^N(1-p)^{n-N},
|
| where :math:`n` is the number of trials, :math:`p` is the probability
| of success, and :math:`N` is the number of successes.
|
| When estimating the standard error of a proportion in a population by
| using a random sample, the normal distribution works well unless the
| product p*n <=5, where p = population proportion estimate, and n =
| number of samples, in which case the binomial distribution is used
| instead. For example, a sample of 15 people shows 4 who are left
| handed, and 11 who are right handed. Then p = 4/15 = 27%. 0.27*15 = 4,
| so the binomial distribution should be used in this case.
|
| References
| ----------
| .. [1] Dalgaard, Peter, "Introductory Statistics with R",
| Springer-Verlag, 2002.
| .. [2] Glantz, Stanton A. "Primer of Biostatistics.", McGraw-Hill,
| Fifth Edition, 2002.
| .. [3] Lentner, Marvin, "Elementary Applied Statistics", Bogden
| and Quigley, 1972.
| .. [4] Weisstein, Eric W. "Binomial Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/BinomialDistribution.html
| .. [5] Wikipedia, "Binomial distribution",
| https://en.wikipedia.org/wiki/Binomial_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> n, p = 10, .5 # number of trials, probability of each trial
| >>> s = np.random.binomial(n, p, 1000)
| # result of flipping a coin 10 times, tested 1000 times.
|
| A real world example. A company drills 9 wild-cat oil exploration
| wells, each with an estimated probability of success of 0.1. All nine
| wells fail. What is the probability of that happening?
|
| Let's do 20,000 trials of the model, and count the number that
| generate zero positive results.
|
| >>> sum(np.random.binomial(9, 0.1, 20000) == 0)/20000.
| # answer = 0.38885, or 38%.
|
| bytes(...)
| bytes(length)
|
| Return random bytes.
|
| .. note::
| New code should use the `~numpy.random.Generator.bytes`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| length : int
| Number of random bytes.
|
| Returns
| -------
| out : bytes
| String of length `length`.
|
| See Also
| --------
| random.Generator.bytes: which should be used for new code.
|
| Examples
| --------
| >>> np.random.bytes(10)
| b' eh\x85\x022SZ\xbf\xa4' #random
|
| chisquare(...)
| chisquare(df, size=None)
|
| Draw samples from a chi-square distribution.
|
| When `df` independent random variables, each with standard normal
| distributions (mean 0, variance 1), are squared and summed, the
| resulting distribution is chi-square (see Notes). This distribution
| is often used in hypothesis testing.
|
| .. note::
| New code should use the `~numpy.random.Generator.chisquare`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| df : float or array_like of floats
| Number of degrees of freedom, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``df`` is a scalar. Otherwise,
| ``np.array(df).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized chi-square distribution.
|
| Raises
| ------
| ValueError
| When `df` <= 0 or when an inappropriate `size` (e.g. ``size=-1``)
| is given.
|
| See Also
| --------
| random.Generator.chisquare: which should be used for new code.
|
| Notes
| -----
| The variable obtained by summing the squares of `df` independent,
| standard normally distributed random variables:
|
| .. math:: Q = \sum_{i=0}^{\mathtt{df}} X^2_i
|
| is chi-square distributed, denoted
|
| .. math:: Q \sim \chi^2_k.
|
| The probability density function of the chi-squared distribution is
|
| .. math:: p(x) = \frac{(1/2)^{k/2}}{\Gamma(k/2)}
| x^{k/2 - 1} e^{-x/2},
|
| where :math:`\Gamma` is the gamma function,
|
| .. math:: \Gamma(x) = \int_0^{-\infty} t^{x - 1} e^{-t} dt.
|
| References
| ----------
| .. [1] NIST "Engineering Statistics Handbook"
| https://www.itl.nist.gov/div898/handbook/eda/section3/eda3666.htm
|
| Examples
| --------
| >>> np.random.chisquare(2,4)
| array([ 1.89920014, 9.00867716, 3.13710533, 5.62318272]) # random
|
| choice(...)
| choice(a, size=None, replace=True, p=None)
|
| Generates a random sample from a given 1-D array
|
| .. versionadded:: 1.7.0
|
| .. note::
| New code should use the `~numpy.random.Generator.choice`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| a : 1-D array-like or int
| If an ndarray, a random sample is generated from its elements.
| If an int, the random sample is generated as if it were ``np.arange(a)``
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| replace : boolean, optional
| Whether the sample is with or without replacement. Default is True,
| meaning that a value of ``a`` can be selected multiple times.
| p : 1-D array-like, optional
| The probabilities associated with each entry in a.
| If not given, the sample assumes a uniform distribution over all
| entries in ``a``.
|
| Returns
| -------
| samples : single item or ndarray
| The generated random samples
|
| Raises
| ------
| ValueError
| If a is an int and less than zero, if a or p are not 1-dimensional,
| if a is an array-like of size 0, if p is not a vector of
| probabilities, if a and p have different lengths, or if
| replace=False and the sample size is greater than the population
| size
|
| See Also
| --------
| randint, shuffle, permutation
| random.Generator.choice: which should be used in new code
|
| Notes
| -----
| Setting user-specified probabilities through ``p`` uses a more general but less
| efficient sampler than the default. The general sampler produces a different sample
| than the optimized sampler even if each element of ``p`` is 1 / len(a).
|
| Sampling random rows from a 2-D array is not possible with this function,
| but is possible with `Generator.choice` through its ``axis`` keyword.
|
| Examples
| --------
| Generate a uniform random sample from np.arange(5) of size 3:
|
| >>> np.random.choice(5, 3)
| array([0, 3, 4]) # random
| >>> #This is equivalent to np.random.randint(0,5,3)
|
| Generate a non-uniform random sample from np.arange(5) of size 3:
|
| >>> np.random.choice(5, 3, p=[0.1, 0, 0.3, 0.6, 0])
| array([3, 3, 0]) # random
|
| Generate a uniform random sample from np.arange(5) of size 3 without
| replacement:
|
| >>> np.random.choice(5, 3, replace=False)
| array([3,1,0]) # random
| >>> #This is equivalent to np.random.permutation(np.arange(5))[:3]
|
| Generate a non-uniform random sample from np.arange(5) of size
| 3 without replacement:
|
| >>> np.random.choice(5, 3, replace=False, p=[0.1, 0, 0.3, 0.6, 0])
| array([2, 3, 0]) # random
|
| Any of the above can be repeated with an arbitrary array-like
| instead of just integers. For instance:
|
| >>> aa_milne_arr = ['pooh', 'rabbit', 'piglet', 'Christopher']
| >>> np.random.choice(aa_milne_arr, 5, p=[0.5, 0.1, 0.1, 0.3])
| array(['pooh', 'pooh', 'pooh', 'Christopher', 'piglet'], # random
| dtype='<U11')
|
| dirichlet(...)
| dirichlet(alpha, size=None)
|
| Draw samples from the Dirichlet distribution.
|
| Draw `size` samples of dimension k from a Dirichlet distribution. A
| Dirichlet-distributed random variable can be seen as a multivariate
| generalization of a Beta distribution. The Dirichlet distribution
| is a conjugate prior of a multinomial distribution in Bayesian
| inference.
|
| .. note::
| New code should use the `~numpy.random.Generator.dirichlet`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| alpha : sequence of floats, length k
| Parameter of the distribution (length ``k`` for sample of
| length ``k``).
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| vector of length ``k`` is returned.
|
| Returns
| -------
| samples : ndarray,
| The drawn samples, of shape ``(size, k)``.
|
| Raises
| ------
| ValueError
| If any value in ``alpha`` is less than or equal to zero
|
| See Also
| --------
| random.Generator.dirichlet: which should be used for new code.
|
| Notes
| -----
| The Dirichlet distribution is a distribution over vectors
| :math:`x` that fulfil the conditions :math:`x_i>0` and
| :math:`\sum_{i=1}^k x_i = 1`.
|
| The probability density function :math:`p` of a
| Dirichlet-distributed random vector :math:`X` is
| proportional to
|
| .. math:: p(x) \propto \prod_{i=1}^{k}{x^{\alpha_i-1}_i},
|
| where :math:`\alpha` is a vector containing the positive
| concentration parameters.
|
| The method uses the following property for computation: let :math:`Y`
| be a random vector which has components that follow a standard gamma
| distribution, then :math:`X = \frac{1}{\sum_{i=1}^k{Y_i}} Y`
| is Dirichlet-distributed
|
| References
| ----------
| .. [1] David McKay, "Information Theory, Inference and Learning
| Algorithms," chapter 23,
| http://www.inference.org.uk/mackay/itila/
| .. [2] Wikipedia, "Dirichlet distribution",
| https://en.wikipedia.org/wiki/Dirichlet_distribution
|
| Examples
| --------
| Taking an example cited in Wikipedia, this distribution can be used if
| one wanted to cut strings (each of initial length 1.0) into K pieces
| with different lengths, where each piece had, on average, a designated
| average length, but allowing some variation in the relative sizes of
| the pieces.
|
| >>> s = np.random.dirichlet((10, 5, 3), 20).transpose()
|
| >>> import matplotlib.pyplot as plt
| >>> plt.barh(range(20), s[0])
| >>> plt.barh(range(20), s[1], left=s[0], color='g')
| >>> plt.barh(range(20), s[2], left=s[0]+s[1], color='r')
| >>> plt.title("Lengths of Strings")
|
| exponential(...)
| exponential(scale=1.0, size=None)
|
| Draw samples from an exponential distribution.
|
| Its probability density function is
|
| .. math:: f(x; \frac{1}{\beta}) = \frac{1}{\beta} \exp(-\frac{x}{\beta}),
|
| for ``x > 0`` and 0 elsewhere. :math:`\beta` is the scale parameter,
| which is the inverse of the rate parameter :math:`\lambda = 1/\beta`.
| The rate parameter is an alternative, widely used parameterization
| of the exponential distribution [3]_.
|
| The exponential distribution is a continuous analogue of the
| geometric distribution. It describes many common situations, such as
| the size of raindrops measured over many rainstorms [1]_, or the time
| between page requests to Wikipedia [2]_.
|
| .. note::
| New code should use the `~numpy.random.Generator.exponential`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| scale : float or array_like of floats
| The scale parameter, :math:`\beta = 1/\lambda`. Must be
| non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``scale`` is a scalar. Otherwise,
| ``np.array(scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized exponential distribution.
|
| Examples
| --------
| A real world example: Assume a company has 10000 customer support
| agents and the average time between customer calls is 4 minutes.
|
| >>> n = 10000
| >>> time_between_calls = np.random.default_rng().exponential(scale=4, size=n)
|
| What is the probability that a customer will call in the next
| 4 to 5 minutes?
|
| >>> x = ((time_between_calls < 5).sum())/n
| >>> y = ((time_between_calls < 4).sum())/n
| >>> x-y
| 0.08 # may vary
|
| See Also
| --------
| random.Generator.exponential: which should be used for new code.
|
| References
| ----------
| .. [1] Peyton Z. Peebles Jr., "Probability, Random Variables and
| Random Signal Principles", 4th ed, 2001, p. 57.
| .. [2] Wikipedia, "Poisson process",
| https://en.wikipedia.org/wiki/Poisson_process
| .. [3] Wikipedia, "Exponential distribution",
| https://en.wikipedia.org/wiki/Exponential_distribution
|
| f(...)
| f(dfnum, dfden, size=None)
|
| Draw samples from an F distribution.
|
| Samples are drawn from an F distribution with specified parameters,
| `dfnum` (degrees of freedom in numerator) and `dfden` (degrees of
| freedom in denominator), where both parameters must be greater than
| zero.
|
| The random variate of the F distribution (also known as the
| Fisher distribution) is a continuous probability distribution
| that arises in ANOVA tests, and is the ratio of two chi-square
| variates.
|
| .. note::
| New code should use the `~numpy.random.Generator.f`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| dfnum : float or array_like of floats
| Degrees of freedom in numerator, must be > 0.
| dfden : float or array_like of float
| Degrees of freedom in denominator, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``dfnum`` and ``dfden`` are both scalars.
| Otherwise, ``np.broadcast(dfnum, dfden).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Fisher distribution.
|
| See Also
| --------
| scipy.stats.f : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.f: which should be used for new code.
|
| Notes
| -----
| The F statistic is used to compare in-group variances to between-group
| variances. Calculating the distribution depends on the sampling, and
| so it is a function of the respective degrees of freedom in the
| problem. The variable `dfnum` is the number of samples minus one, the
| between-groups degrees of freedom, while `dfden` is the within-groups
| degrees of freedom, the sum of the number of samples in each group
| minus the number of groups.
|
| References
| ----------
| .. [1] Glantz, Stanton A. "Primer of Biostatistics.", McGraw-Hill,
| Fifth Edition, 2002.
| .. [2] Wikipedia, "F-distribution",
| https://en.wikipedia.org/wiki/F-distribution
|
| Examples
| --------
| An example from Glantz[1], pp 47-40:
|
| Two groups, children of diabetics (25 people) and children from people
| without diabetes (25 controls). Fasting blood glucose was measured,
| case group had a mean value of 86.1, controls had a mean value of
| 82.2. Standard deviations were 2.09 and 2.49 respectively. Are these
| data consistent with the null hypothesis that the parents diabetic
| status does not affect their children's blood glucose levels?
| Calculating the F statistic from the data gives a value of 36.01.
|
| Draw samples from the distribution:
|
| >>> dfnum = 1. # between group degrees of freedom
| >>> dfden = 48. # within groups degrees of freedom
| >>> s = np.random.f(dfnum, dfden, 1000)
|
| The lower bound for the top 1% of the samples is :
|
| >>> np.sort(s)[-10]
| 7.61988120985 # random
|
| So there is about a 1% chance that the F statistic will exceed 7.62,
| the measured value is 36, so the null hypothesis is rejected at the 1%
| level.
|
| gamma(...)
| gamma(shape, scale=1.0, size=None)
|
| Draw samples from a Gamma distribution.
|
| Samples are drawn from a Gamma distribution with specified parameters,
| `shape` (sometimes designated "k") and `scale` (sometimes designated
| "theta"), where both parameters are > 0.
|
| .. note::
| New code should use the `~numpy.random.Generator.gamma`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| shape : float or array_like of floats
| The shape of the gamma distribution. Must be non-negative.
| scale : float or array_like of floats, optional
| The scale of the gamma distribution. Must be non-negative.
| Default is equal to 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``shape`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(shape, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized gamma distribution.
|
| See Also
| --------
| scipy.stats.gamma : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.gamma: which should be used for new code.
|
| Notes
| -----
| The probability density for the Gamma distribution is
|
| .. math:: p(x) = x^{k-1}\frac{e^{-x/\theta}}{\theta^k\Gamma(k)},
|
| where :math:`k` is the shape and :math:`\theta` the scale,
| and :math:`\Gamma` is the Gamma function.
|
| The Gamma distribution is often used to model the times to failure of
| electronic components, and arises naturally in processes for which the
| waiting times between Poisson distributed events are relevant.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Gamma Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/GammaDistribution.html
| .. [2] Wikipedia, "Gamma distribution",
| https://en.wikipedia.org/wiki/Gamma_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> shape, scale = 2., 2. # mean=4, std=2*sqrt(2)
| >>> s = np.random.gamma(shape, scale, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> import scipy.special as sps # doctest: +SKIP
| >>> count, bins, ignored = plt.hist(s, 50, density=True)
| >>> y = bins**(shape-1)*(np.exp(-bins/scale) / # doctest: +SKIP
| ... (sps.gamma(shape)*scale**shape))
| >>> plt.plot(bins, y, linewidth=2, color='r') # doctest: +SKIP
| >>> plt.show()
|
| geometric(...)
| geometric(p, size=None)
|
| Draw samples from the geometric distribution.
|
| Bernoulli trials are experiments with one of two outcomes:
| success or failure (an example of such an experiment is flipping
| a coin). The geometric distribution models the number of trials
| that must be run in order to achieve success. It is therefore
| supported on the positive integers, ``k = 1, 2, ...``.
|
| The probability mass function of the geometric distribution is
|
| .. math:: f(k) = (1 - p)^{k - 1} p
|
| where `p` is the probability of success of an individual trial.
|
| .. note::
| New code should use the `~numpy.random.Generator.geometric`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| p : float or array_like of floats
| The probability of success of an individual trial.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``p`` is a scalar. Otherwise,
| ``np.array(p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized geometric distribution.
|
| See Also
| --------
| random.Generator.geometric: which should be used for new code.
|
| Examples
| --------
| Draw ten thousand values from the geometric distribution,
| with the probability of an individual success equal to 0.35:
|
| >>> z = np.random.geometric(p=0.35, size=10000)
|
| How many trials succeeded after a single run?
|
| >>> (z == 1).sum() / 10000.
| 0.34889999999999999 #random
|
| get_state(...)
| get_state(legacy=True)
|
| Return a tuple representing the internal state of the generator.
|
| For more details, see `set_state`.
|
| Parameters
| ----------
| legacy : bool, optional
| Flag indicating to return a legacy tuple state when the BitGenerator
| is MT19937, instead of a dict. Raises ValueError if the underlying
| bit generator is not an instance of MT19937.
|
| Returns
| -------
| out : {tuple(str, ndarray of 624 uints, int, int, float), dict}
| If legacy is True, the returned tuple has the following items:
|
| 1. the string 'MT19937'.
| 2. a 1-D array of 624 unsigned integer keys.
| 3. an integer ``pos``.
| 4. an integer ``has_gauss``.
| 5. a float ``cached_gaussian``.
|
| If `legacy` is False, or the BitGenerator is not MT19937, then
| state is returned as a dictionary.
|
| See Also
| --------
| set_state
|
| Notes
| -----
| `set_state` and `get_state` are not needed to work with any of the
| random distributions in NumPy. If the internal state is manually altered,
| the user should know exactly what he/she is doing.
|
| gumbel(...)
| gumbel(loc=0.0, scale=1.0, size=None)
|
| Draw samples from a Gumbel distribution.
|
| Draw samples from a Gumbel distribution with specified location and
| scale. For more information on the Gumbel distribution, see
| Notes and References below.
|
| .. note::
| New code should use the `~numpy.random.Generator.gumbel`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| loc : float or array_like of floats, optional
| The location of the mode of the distribution. Default is 0.
| scale : float or array_like of floats, optional
| The scale parameter of the distribution. Default is 1. Must be non-
| negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Gumbel distribution.
|
| See Also
| --------
| scipy.stats.gumbel_l
| scipy.stats.gumbel_r
| scipy.stats.genextreme
| weibull
| random.Generator.gumbel: which should be used for new code.
|
| Notes
| -----
| The Gumbel (or Smallest Extreme Value (SEV) or the Smallest Extreme
| Value Type I) distribution is one of a class of Generalized Extreme
| Value (GEV) distributions used in modeling extreme value problems.
| The Gumbel is a special case of the Extreme Value Type I distribution
| for maximums from distributions with "exponential-like" tails.
|
| The probability density for the Gumbel distribution is
|
| .. math:: p(x) = \frac{e^{-(x - \mu)/ \beta}}{\beta} e^{ -e^{-(x - \mu)/
| \beta}},
|
| where :math:`\mu` is the mode, a location parameter, and
| :math:`\beta` is the scale parameter.
|
| The Gumbel (named for German mathematician Emil Julius Gumbel) was used
| very early in the hydrology literature, for modeling the occurrence of
| flood events. It is also used for modeling maximum wind speed and
| rainfall rates. It is a "fat-tailed" distribution - the probability of
| an event in the tail of the distribution is larger than if one used a
| Gaussian, hence the surprisingly frequent occurrence of 100-year
| floods. Floods were initially modeled as a Gaussian process, which
| underestimated the frequency of extreme events.
|
| It is one of a class of extreme value distributions, the Generalized
| Extreme Value (GEV) distributions, which also includes the Weibull and
| Frechet.
|
| The function has a mean of :math:`\mu + 0.57721\beta` and a variance
| of :math:`\frac{\pi^2}{6}\beta^2`.
|
| References
| ----------
| .. [1] Gumbel, E. J., "Statistics of Extremes,"
| New York: Columbia University Press, 1958.
| .. [2] Reiss, R.-D. and Thomas, M., "Statistical Analysis of Extreme
| Values from Insurance, Finance, Hydrology and Other Fields,"
| Basel: Birkhauser Verlag, 2001.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> mu, beta = 0, 0.1 # location and scale
| >>> s = np.random.gumbel(mu, beta, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 30, density=True)
| >>> plt.plot(bins, (1/beta)*np.exp(-(bins - mu)/beta)
| ... * np.exp( -np.exp( -(bins - mu) /beta) ),
| ... linewidth=2, color='r')
| >>> plt.show()
|
| Show how an extreme value distribution can arise from a Gaussian process
| and compare to a Gaussian:
|
| >>> means = []
| >>> maxima = []
| >>> for i in range(0,1000) :
| ... a = np.random.normal(mu, beta, 1000)
| ... means.append(a.mean())
| ... maxima.append(a.max())
| >>> count, bins, ignored = plt.hist(maxima, 30, density=True)
| >>> beta = np.std(maxima) * np.sqrt(6) / np.pi
| >>> mu = np.mean(maxima) - 0.57721*beta
| >>> plt.plot(bins, (1/beta)*np.exp(-(bins - mu)/beta)
| ... * np.exp(-np.exp(-(bins - mu)/beta)),
| ... linewidth=2, color='r')
| >>> plt.plot(bins, 1/(beta * np.sqrt(2 * np.pi))
| ... * np.exp(-(bins - mu)**2 / (2 * beta**2)),
| ... linewidth=2, color='g')
| >>> plt.show()
|
| hypergeometric(...)
| hypergeometric(ngood, nbad, nsample, size=None)
|
| Draw samples from a Hypergeometric distribution.
|
| Samples are drawn from a hypergeometric distribution with specified
| parameters, `ngood` (ways to make a good selection), `nbad` (ways to make
| a bad selection), and `nsample` (number of items sampled, which is less
| than or equal to the sum ``ngood + nbad``).
|
| .. note::
| New code should use the
| `~numpy.random.Generator.hypergeometric`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| ngood : int or array_like of ints
| Number of ways to make a good selection. Must be nonnegative.
| nbad : int or array_like of ints
| Number of ways to make a bad selection. Must be nonnegative.
| nsample : int or array_like of ints
| Number of items sampled. Must be at least 1 and at most
| ``ngood + nbad``.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if `ngood`, `nbad`, and `nsample`
| are all scalars. Otherwise, ``np.broadcast(ngood, nbad, nsample).size``
| samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized hypergeometric distribution. Each
| sample is the number of good items within a randomly selected subset of
| size `nsample` taken from a set of `ngood` good items and `nbad` bad items.
|
| See Also
| --------
| scipy.stats.hypergeom : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.hypergeometric: which should be used for new code.
|
| Notes
| -----
| The probability density for the Hypergeometric distribution is
|
| .. math:: P(x) = \frac{\binom{g}{x}\binom{b}{n-x}}{\binom{g+b}{n}},
|
| where :math:`0 \le x \le n` and :math:`n-b \le x \le g`
|
| for P(x) the probability of ``x`` good results in the drawn sample,
| g = `ngood`, b = `nbad`, and n = `nsample`.
|
| Consider an urn with black and white marbles in it, `ngood` of them
| are black and `nbad` are white. If you draw `nsample` balls without
| replacement, then the hypergeometric distribution describes the
| distribution of black balls in the drawn sample.
|
| Note that this distribution is very similar to the binomial
| distribution, except that in this case, samples are drawn without
| replacement, whereas in the Binomial case samples are drawn with
| replacement (or the sample space is infinite). As the sample space
| becomes large, this distribution approaches the binomial.
|
| References
| ----------
| .. [1] Lentner, Marvin, "Elementary Applied Statistics", Bogden
| and Quigley, 1972.
| .. [2] Weisstein, Eric W. "Hypergeometric Distribution." From
| MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/HypergeometricDistribution.html
| .. [3] Wikipedia, "Hypergeometric distribution",
| https://en.wikipedia.org/wiki/Hypergeometric_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> ngood, nbad, nsamp = 100, 2, 10
| # number of good, number of bad, and number of samples
| >>> s = np.random.hypergeometric(ngood, nbad, nsamp, 1000)
| >>> from matplotlib.pyplot import hist
| >>> hist(s)
| # note that it is very unlikely to grab both bad items
|
| Suppose you have an urn with 15 white and 15 black marbles.
| If you pull 15 marbles at random, how likely is it that
| 12 or more of them are one color?
|
| >>> s = np.random.hypergeometric(15, 15, 15, 100000)
| >>> sum(s>=12)/100000. + sum(s<=3)/100000.
| # answer = 0.003 ... pretty unlikely!
|
| laplace(...)
| laplace(loc=0.0, scale=1.0, size=None)
|
| Draw samples from the Laplace or double exponential distribution with
| specified location (or mean) and scale (decay).
|
| The Laplace distribution is similar to the Gaussian/normal distribution,
| but is sharper at the peak and has fatter tails. It represents the
| difference between two independent, identically distributed exponential
| random variables.
|
| .. note::
| New code should use the `~numpy.random.Generator.laplace`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| loc : float or array_like of floats, optional
| The position, :math:`\mu`, of the distribution peak. Default is 0.
| scale : float or array_like of floats, optional
| :math:`\lambda`, the exponential decay. Default is 1. Must be non-
| negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Laplace distribution.
|
| See Also
| --------
| random.Generator.laplace: which should be used for new code.
|
| Notes
| -----
| It has the probability density function
|
| .. math:: f(x; \mu, \lambda) = \frac{1}{2\lambda}
| \exp\left(-\frac{|x - \mu|}{\lambda}\right).
|
| The first law of Laplace, from 1774, states that the frequency
| of an error can be expressed as an exponential function of the
| absolute magnitude of the error, which leads to the Laplace
| distribution. For many problems in economics and health
| sciences, this distribution seems to model the data better
| than the standard Gaussian distribution.
|
| References
| ----------
| .. [1] Abramowitz, M. and Stegun, I. A. (Eds.). "Handbook of
| Mathematical Functions with Formulas, Graphs, and Mathematical
| Tables, 9th printing," New York: Dover, 1972.
| .. [2] Kotz, Samuel, et. al. "The Laplace Distribution and
| Generalizations, " Birkhauser, 2001.
| .. [3] Weisstein, Eric W. "Laplace Distribution."
| From MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/LaplaceDistribution.html
| .. [4] Wikipedia, "Laplace distribution",
| https://en.wikipedia.org/wiki/Laplace_distribution
|
| Examples
| --------
| Draw samples from the distribution
|
| >>> loc, scale = 0., 1.
| >>> s = np.random.laplace(loc, scale, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 30, density=True)
| >>> x = np.arange(-8., 8., .01)
| >>> pdf = np.exp(-abs(x-loc)/scale)/(2.*scale)
| >>> plt.plot(x, pdf)
|
| Plot Gaussian for comparison:
|
| >>> g = (1/(scale * np.sqrt(2 * np.pi)) *
| ... np.exp(-(x - loc)**2 / (2 * scale**2)))
| >>> plt.plot(x,g)
|
| logistic(...)
| logistic(loc=0.0, scale=1.0, size=None)
|
| Draw samples from a logistic distribution.
|
| Samples are drawn from a logistic distribution with specified
| parameters, loc (location or mean, also median), and scale (>0).
|
| .. note::
| New code should use the `~numpy.random.Generator.logistic`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| loc : float or array_like of floats, optional
| Parameter of the distribution. Default is 0.
| scale : float or array_like of floats, optional
| Parameter of the distribution. Must be non-negative.
| Default is 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized logistic distribution.
|
| See Also
| --------
| scipy.stats.logistic : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.logistic: which should be used for new code.
|
| Notes
| -----
| The probability density for the Logistic distribution is
|
| .. math:: P(x) = P(x) = \frac{e^{-(x-\mu)/s}}{s(1+e^{-(x-\mu)/s})^2},
|
| where :math:`\mu` = location and :math:`s` = scale.
|
| The Logistic distribution is used in Extreme Value problems where it
| can act as a mixture of Gumbel distributions, in Epidemiology, and by
| the World Chess Federation (FIDE) where it is used in the Elo ranking
| system, assuming the performance of each player is a logistically
| distributed random variable.
|
| References
| ----------
| .. [1] Reiss, R.-D. and Thomas M. (2001), "Statistical Analysis of
| Extreme Values, from Insurance, Finance, Hydrology and Other
| Fields," Birkhauser Verlag, Basel, pp 132-133.
| .. [2] Weisstein, Eric W. "Logistic Distribution." From
| MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/LogisticDistribution.html
| .. [3] Wikipedia, "Logistic-distribution",
| https://en.wikipedia.org/wiki/Logistic_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> loc, scale = 10, 1
| >>> s = np.random.logistic(loc, scale, 10000)
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, bins=50)
|
| # plot against distribution
|
| >>> def logist(x, loc, scale):
| ... return np.exp((loc-x)/scale)/(scale*(1+np.exp((loc-x)/scale))**2)
| >>> lgst_val = logist(bins, loc, scale)
| >>> plt.plot(bins, lgst_val * count.max() / lgst_val.max())
| >>> plt.show()
|
| lognormal(...)
| lognormal(mean=0.0, sigma=1.0, size=None)
|
| Draw samples from a log-normal distribution.
|
| Draw samples from a log-normal distribution with specified mean,
| standard deviation, and array shape. Note that the mean and standard
| deviation are not the values for the distribution itself, but of the
| underlying normal distribution it is derived from.
|
| .. note::
| New code should use the `~numpy.random.Generator.lognormal`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| mean : float or array_like of floats, optional
| Mean value of the underlying normal distribution. Default is 0.
| sigma : float or array_like of floats, optional
| Standard deviation of the underlying normal distribution. Must be
| non-negative. Default is 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``mean`` and ``sigma`` are both scalars.
| Otherwise, ``np.broadcast(mean, sigma).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized log-normal distribution.
|
| See Also
| --------
| scipy.stats.lognorm : probability density function, distribution,
| cumulative density function, etc.
| random.Generator.lognormal: which should be used for new code.
|
| Notes
| -----
| A variable `x` has a log-normal distribution if `log(x)` is normally
| distributed. The probability density function for the log-normal
| distribution is:
|
| .. math:: p(x) = \frac{1}{\sigma x \sqrt{2\pi}}
| e^{(-\frac{(ln(x)-\mu)^2}{2\sigma^2})}
|
| where :math:`\mu` is the mean and :math:`\sigma` is the standard
| deviation of the normally distributed logarithm of the variable.
| A log-normal distribution results if a random variable is the *product*
| of a large number of independent, identically-distributed variables in
| the same way that a normal distribution results if the variable is the
| *sum* of a large number of independent, identically-distributed
| variables.
|
| References
| ----------
| .. [1] Limpert, E., Stahel, W. A., and Abbt, M., "Log-normal
| Distributions across the Sciences: Keys and Clues,"
| BioScience, Vol. 51, No. 5, May, 2001.
| https://stat.ethz.ch/~stahel/lognormal/bioscience.pdf
| .. [2] Reiss, R.D. and Thomas, M., "Statistical Analysis of Extreme
| Values," Basel: Birkhauser Verlag, 2001, pp. 31-32.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> mu, sigma = 3., 1. # mean and standard deviation
| >>> s = np.random.lognormal(mu, sigma, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 100, density=True, align='mid')
|
| >>> x = np.linspace(min(bins), max(bins), 10000)
| >>> pdf = (np.exp(-(np.log(x) - mu)**2 / (2 * sigma**2))
| ... / (x * sigma * np.sqrt(2 * np.pi)))
|
| >>> plt.plot(x, pdf, linewidth=2, color='r')
| >>> plt.axis('tight')
| >>> plt.show()
|
| Demonstrate that taking the products of random samples from a uniform
| distribution can be fit well by a log-normal probability density
| function.
|
| >>> # Generate a thousand samples: each is the product of 100 random
| >>> # values, drawn from a normal distribution.
| >>> b = []
| >>> for i in range(1000):
| ... a = 10. + np.random.standard_normal(100)
| ... b.append(np.prod(a))
|
| >>> b = np.array(b) / np.min(b) # scale values to be positive
| >>> count, bins, ignored = plt.hist(b, 100, density=True, align='mid')
| >>> sigma = np.std(np.log(b))
| >>> mu = np.mean(np.log(b))
|
| >>> x = np.linspace(min(bins), max(bins), 10000)
| >>> pdf = (np.exp(-(np.log(x) - mu)**2 / (2 * sigma**2))
| ... / (x * sigma * np.sqrt(2 * np.pi)))
|
| >>> plt.plot(x, pdf, color='r', linewidth=2)
| >>> plt.show()
|
| logseries(...)
| logseries(p, size=None)
|
| Draw samples from a logarithmic series distribution.
|
| Samples are drawn from a log series distribution with specified
| shape parameter, 0 <= ``p`` < 1.
|
| .. note::
| New code should use the `~numpy.random.Generator.logseries`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| p : float or array_like of floats
| Shape parameter for the distribution. Must be in the range [0, 1).
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``p`` is a scalar. Otherwise,
| ``np.array(p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized logarithmic series distribution.
|
| See Also
| --------
| scipy.stats.logser : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.logseries: which should be used for new code.
|
| Notes
| -----
| The probability density for the Log Series distribution is
|
| .. math:: P(k) = \frac{-p^k}{k \ln(1-p)},
|
| where p = probability.
|
| The log series distribution is frequently used to represent species
| richness and occurrence, first proposed by Fisher, Corbet, and
| Williams in 1943 [2]. It may also be used to model the numbers of
| occupants seen in cars [3].
|
| References
| ----------
| .. [1] Buzas, Martin A.; Culver, Stephen J., Understanding regional
| species diversity through the log series distribution of
| occurrences: BIODIVERSITY RESEARCH Diversity & Distributions,
| Volume 5, Number 5, September 1999 , pp. 187-195(9).
| .. [2] Fisher, R.A,, A.S. Corbet, and C.B. Williams. 1943. The
| relation between the number of species and the number of
| individuals in a random sample of an animal population.
| Journal of Animal Ecology, 12:42-58.
| .. [3] D. J. Hand, F. Daly, D. Lunn, E. Ostrowski, A Handbook of Small
| Data Sets, CRC Press, 1994.
| .. [4] Wikipedia, "Logarithmic distribution",
| https://en.wikipedia.org/wiki/Logarithmic_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a = .6
| >>> s = np.random.logseries(a, 10000)
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s)
|
| # plot against distribution
|
| >>> def logseries(k, p):
| ... return -p**k/(k*np.log(1-p))
| >>> plt.plot(bins, logseries(bins, a)*count.max()/
| ... logseries(bins, a).max(), 'r')
| >>> plt.show()
|
| multinomial(...)
| multinomial(n, pvals, size=None)
|
| Draw samples from a multinomial distribution.
|
| The multinomial distribution is a multivariate generalization of the
| binomial distribution. Take an experiment with one of ``p``
| possible outcomes. An example of such an experiment is throwing a dice,
| where the outcome can be 1 through 6. Each sample drawn from the
| distribution represents `n` such experiments. Its values,
| ``X_i = [X_0, X_1, ..., X_p]``, represent the number of times the
| outcome was ``i``.
|
| .. note::
| New code should use the `~numpy.random.Generator.multinomial`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| n : int
| Number of experiments.
| pvals : sequence of floats, length p
| Probabilities of each of the ``p`` different outcomes. These
| must sum to 1 (however, the last element is always assumed to
| account for the remaining probability, as long as
| ``sum(pvals[:-1]) <= 1)``.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| out : ndarray
| The drawn samples, of shape *size*, if that was provided. If not,
| the shape is ``(N,)``.
|
| In other words, each entry ``out[i,j,...,:]`` is an N-dimensional
| value drawn from the distribution.
|
| See Also
| --------
| random.Generator.multinomial: which should be used for new code.
|
| Examples
| --------
| Throw a dice 20 times:
|
| >>> np.random.multinomial(20, [1/6.]*6, size=1)
| array([[4, 1, 7, 5, 2, 1]]) # random
|
| It landed 4 times on 1, once on 2, etc.
|
| Now, throw the dice 20 times, and 20 times again:
|
| >>> np.random.multinomial(20, [1/6.]*6, size=2)
| array([[3, 4, 3, 3, 4, 3], # random
| [2, 4, 3, 4, 0, 7]])
|
| For the first run, we threw 3 times 1, 4 times 2, etc. For the second,
| we threw 2 times 1, 4 times 2, etc.
|
| A loaded die is more likely to land on number 6:
|
| >>> np.random.multinomial(100, [1/7.]*5 + [2/7.])
| array([11, 16, 14, 17, 16, 26]) # random
|
| The probability inputs should be normalized. As an implementation
| detail, the value of the last entry is ignored and assumed to take
| up any leftover probability mass, but this should not be relied on.
| A biased coin which has twice as much weight on one side as on the
| other should be sampled like so:
|
| >>> np.random.multinomial(100, [1.0 / 3, 2.0 / 3]) # RIGHT
| array([38, 62]) # random
|
| not like:
|
| >>> np.random.multinomial(100, [1.0, 2.0]) # WRONG
| Traceback (most recent call last):
| ValueError: pvals < 0, pvals > 1 or pvals contains NaNs
|
| multivariate_normal(...)
| multivariate_normal(mean, cov, size=None, check_valid='warn', tol=1e-8)
|
| Draw random samples from a multivariate normal distribution.
|
| The multivariate normal, multinormal or Gaussian distribution is a
| generalization of the one-dimensional normal distribution to higher
| dimensions. Such a distribution is specified by its mean and
| covariance matrix. These parameters are analogous to the mean
| (average or "center") and variance (standard deviation, or "width,"
| squared) of the one-dimensional normal distribution.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.multivariate_normal`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| mean : 1-D array_like, of length N
| Mean of the N-dimensional distribution.
| cov : 2-D array_like, of shape (N, N)
| Covariance matrix of the distribution. It must be symmetric and
| positive-semidefinite for proper sampling.
| size : int or tuple of ints, optional
| Given a shape of, for example, ``(m,n,k)``, ``m*n*k`` samples are
| generated, and packed in an `m`-by-`n`-by-`k` arrangement. Because
| each sample is `N`-dimensional, the output shape is ``(m,n,k,N)``.
| If no shape is specified, a single (`N`-D) sample is returned.
| check_valid : { 'warn', 'raise', 'ignore' }, optional
| Behavior when the covariance matrix is not positive semidefinite.
| tol : float, optional
| Tolerance when checking the singular values in covariance matrix.
| cov is cast to double before the check.
|
| Returns
| -------
| out : ndarray
| The drawn samples, of shape *size*, if that was provided. If not,
| the shape is ``(N,)``.
|
| In other words, each entry ``out[i,j,...,:]`` is an N-dimensional
| value drawn from the distribution.
|
| See Also
| --------
| random.Generator.multivariate_normal: which should be used for new code.
|
| Notes
| -----
| The mean is a coordinate in N-dimensional space, which represents the
| location where samples are most likely to be generated. This is
| analogous to the peak of the bell curve for the one-dimensional or
| univariate normal distribution.
|
| Covariance indicates the level to which two variables vary together.
| From the multivariate normal distribution, we draw N-dimensional
| samples, :math:`X = [x_1, x_2, ... x_N]`. The covariance matrix
| element :math:`C_{ij}` is the covariance of :math:`x_i` and :math:`x_j`.
| The element :math:`C_{ii}` is the variance of :math:`x_i` (i.e. its
| "spread").
|
| Instead of specifying the full covariance matrix, popular
| approximations include:
|
| - Spherical covariance (`cov` is a multiple of the identity matrix)
| - Diagonal covariance (`cov` has non-negative elements, and only on
| the diagonal)
|
| This geometrical property can be seen in two dimensions by plotting
| generated data-points:
|
| >>> mean = [0, 0]
| >>> cov = [[1, 0], [0, 100]] # diagonal covariance
|
| Diagonal covariance means that points are oriented along x or y-axis:
|
| >>> import matplotlib.pyplot as plt
| >>> x, y = np.random.multivariate_normal(mean, cov, 5000).T
| >>> plt.plot(x, y, 'x')
| >>> plt.axis('equal')
| >>> plt.show()
|
| Note that the covariance matrix must be positive semidefinite (a.k.a.
| nonnegative-definite). Otherwise, the behavior of this method is
| undefined and backwards compatibility is not guaranteed.
|
| References
| ----------
| .. [1] Papoulis, A., "Probability, Random Variables, and Stochastic
| Processes," 3rd ed., New York: McGraw-Hill, 1991.
| .. [2] Duda, R. O., Hart, P. E., and Stork, D. G., "Pattern
| Classification," 2nd ed., New York: Wiley, 2001.
|
| Examples
| --------
| >>> mean = (1, 2)
| >>> cov = [[1, 0], [0, 1]]
| >>> x = np.random.multivariate_normal(mean, cov, (3, 3))
| >>> x.shape
| (3, 3, 2)
|
| Here we generate 800 samples from the bivariate normal distribution
| with mean [0, 0] and covariance matrix [[6, -3], [-3, 3.5]]. The
| expected variances of the first and second components of the sample
| are 6 and 3.5, respectively, and the expected correlation
| coefficient is -3/sqrt(6*3.5) ≈ -0.65465.
|
| >>> cov = np.array([[6, -3], [-3, 3.5]])
| >>> pts = np.random.multivariate_normal([0, 0], cov, size=800)
|
| Check that the mean, covariance, and correlation coefficient of the
| sample are close to the expected values:
|
| >>> pts.mean(axis=0)
| array([ 0.0326911 , -0.01280782]) # may vary
| >>> np.cov(pts.T)
| array([[ 5.96202397, -2.85602287],
| [-2.85602287, 3.47613949]]) # may vary
| >>> np.corrcoef(pts.T)[0, 1]
| -0.6273591314603949 # may vary
|
| We can visualize this data with a scatter plot. The orientation
| of the point cloud illustrates the negative correlation of the
| components of this sample.
|
| >>> import matplotlib.pyplot as plt
| >>> plt.plot(pts[:, 0], pts[:, 1], '.', alpha=0.5)
| >>> plt.axis('equal')
| >>> plt.grid()
| >>> plt.show()
|
| negative_binomial(...)
| negative_binomial(n, p, size=None)
|
| Draw samples from a negative binomial distribution.
|
| Samples are drawn from a negative binomial distribution with specified
| parameters, `n` successes and `p` probability of success where `n`
| is > 0 and `p` is in the interval [0, 1].
|
| .. note::
| New code should use the
| `~numpy.random.Generator.negative_binomial`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| n : float or array_like of floats
| Parameter of the distribution, > 0.
| p : float or array_like of floats
| Parameter of the distribution, >= 0 and <=1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``n`` and ``p`` are both scalars.
| Otherwise, ``np.broadcast(n, p).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized negative binomial distribution,
| where each sample is equal to N, the number of failures that
| occurred before a total of n successes was reached.
|
| See Also
| --------
| random.Generator.negative_binomial: which should be used for new code.
|
| Notes
| -----
| The probability mass function of the negative binomial distribution is
|
| .. math:: P(N;n,p) = \frac{\Gamma(N+n)}{N!\Gamma(n)}p^{n}(1-p)^{N},
|
| where :math:`n` is the number of successes, :math:`p` is the
| probability of success, :math:`N+n` is the number of trials, and
| :math:`\Gamma` is the gamma function. When :math:`n` is an integer,
| :math:`\frac{\Gamma(N+n)}{N!\Gamma(n)} = \binom{N+n-1}{N}`, which is
| the more common form of this term in the pmf. The negative
| binomial distribution gives the probability of N failures given n
| successes, with a success on the last trial.
|
| If one throws a die repeatedly until the third time a "1" appears,
| then the probability distribution of the number of non-"1"s that
| appear before the third "1" is a negative binomial distribution.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Negative Binomial Distribution." From
| MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/NegativeBinomialDistribution.html
| .. [2] Wikipedia, "Negative binomial distribution",
| https://en.wikipedia.org/wiki/Negative_binomial_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| A real world example. A company drills wild-cat oil
| exploration wells, each with an estimated probability of
| success of 0.1. What is the probability of having one success
| for each successive well, that is what is the probability of a
| single success after drilling 5 wells, after 6 wells, etc.?
|
| >>> s = np.random.negative_binomial(1, 0.1, 100000)
| >>> for i in range(1, 11): # doctest: +SKIP
| ... probability = sum(s<i) / 100000.
| ... print(i, "wells drilled, probability of one success =", probability)
|
| noncentral_chisquare(...)
| noncentral_chisquare(df, nonc, size=None)
|
| Draw samples from a noncentral chi-square distribution.
|
| The noncentral :math:`\chi^2` distribution is a generalization of
| the :math:`\chi^2` distribution.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.noncentral_chisquare`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| df : float or array_like of floats
| Degrees of freedom, must be > 0.
|
| .. versionchanged:: 1.10.0
| Earlier NumPy versions required dfnum > 1.
| nonc : float or array_like of floats
| Non-centrality, must be non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``df`` and ``nonc`` are both scalars.
| Otherwise, ``np.broadcast(df, nonc).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized noncentral chi-square distribution.
|
| See Also
| --------
| random.Generator.noncentral_chisquare: which should be used for new code.
|
| Notes
| -----
| The probability density function for the noncentral Chi-square
| distribution is
|
| .. math:: P(x;df,nonc) = \sum^{\infty}_{i=0}
| \frac{e^{-nonc/2}(nonc/2)^{i}}{i!}
| P_{Y_{df+2i}}(x),
|
| where :math:`Y_{q}` is the Chi-square with q degrees of freedom.
|
| References
| ----------
| .. [1] Wikipedia, "Noncentral chi-squared distribution"
| https://en.wikipedia.org/wiki/Noncentral_chi-squared_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram
|
| >>> import matplotlib.pyplot as plt
| >>> values = plt.hist(np.random.noncentral_chisquare(3, 20, 100000),
| ... bins=200, density=True)
| >>> plt.show()
|
| Draw values from a noncentral chisquare with very small noncentrality,
| and compare to a chisquare.
|
| >>> plt.figure()
| >>> values = plt.hist(np.random.noncentral_chisquare(3, .0000001, 100000),
| ... bins=np.arange(0., 25, .1), density=True)
| >>> values2 = plt.hist(np.random.chisquare(3, 100000),
| ... bins=np.arange(0., 25, .1), density=True)
| >>> plt.plot(values[1][0:-1], values[0]-values2[0], 'ob')
| >>> plt.show()
|
| Demonstrate how large values of non-centrality lead to a more symmetric
| distribution.
|
| >>> plt.figure()
| >>> values = plt.hist(np.random.noncentral_chisquare(3, 20, 100000),
| ... bins=200, density=True)
| >>> plt.show()
|
| noncentral_f(...)
| noncentral_f(dfnum, dfden, nonc, size=None)
|
| Draw samples from the noncentral F distribution.
|
| Samples are drawn from an F distribution with specified parameters,
| `dfnum` (degrees of freedom in numerator) and `dfden` (degrees of
| freedom in denominator), where both parameters > 1.
| `nonc` is the non-centrality parameter.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.noncentral_f`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| dfnum : float or array_like of floats
| Numerator degrees of freedom, must be > 0.
|
| .. versionchanged:: 1.14.0
| Earlier NumPy versions required dfnum > 1.
| dfden : float or array_like of floats
| Denominator degrees of freedom, must be > 0.
| nonc : float or array_like of floats
| Non-centrality parameter, the sum of the squares of the numerator
| means, must be >= 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``dfnum``, ``dfden``, and ``nonc``
| are all scalars. Otherwise, ``np.broadcast(dfnum, dfden, nonc).size``
| samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized noncentral Fisher distribution.
|
| See Also
| --------
| random.Generator.noncentral_f: which should be used for new code.
|
| Notes
| -----
| When calculating the power of an experiment (power = probability of
| rejecting the null hypothesis when a specific alternative is true) the
| non-central F statistic becomes important. When the null hypothesis is
| true, the F statistic follows a central F distribution. When the null
| hypothesis is not true, then it follows a non-central F statistic.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Noncentral F-Distribution."
| From MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/NoncentralF-Distribution.html
| .. [2] Wikipedia, "Noncentral F-distribution",
| https://en.wikipedia.org/wiki/Noncentral_F-distribution
|
| Examples
| --------
| In a study, testing for a specific alternative to the null hypothesis
| requires use of the Noncentral F distribution. We need to calculate the
| area in the tail of the distribution that exceeds the value of the F
| distribution for the null hypothesis. We'll plot the two probability
| distributions for comparison.
|
| >>> dfnum = 3 # between group deg of freedom
| >>> dfden = 20 # within groups degrees of freedom
| >>> nonc = 3.0
| >>> nc_vals = np.random.noncentral_f(dfnum, dfden, nonc, 1000000)
| >>> NF = np.histogram(nc_vals, bins=50, density=True)
| >>> c_vals = np.random.f(dfnum, dfden, 1000000)
| >>> F = np.histogram(c_vals, bins=50, density=True)
| >>> import matplotlib.pyplot as plt
| >>> plt.plot(F[1][1:], F[0])
| >>> plt.plot(NF[1][1:], NF[0])
| >>> plt.show()
|
| normal(...)
| normal(loc=0.0, scale=1.0, size=None)
|
| Draw random samples from a normal (Gaussian) distribution.
|
| The probability density function of the normal distribution, first
| derived by De Moivre and 200 years later by both Gauss and Laplace
| independently [2]_, is often called the bell curve because of
| its characteristic shape (see the example below).
|
| The normal distributions occurs often in nature. For example, it
| describes the commonly occurring distribution of samples influenced
| by a large number of tiny, random disturbances, each with its own
| unique distribution [2]_.
|
| .. note::
| New code should use the `~numpy.random.Generator.normal`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| loc : float or array_like of floats
| Mean ("centre") of the distribution.
| scale : float or array_like of floats
| Standard deviation (spread or "width") of the distribution. Must be
| non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``loc`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized normal distribution.
|
| See Also
| --------
| scipy.stats.norm : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.normal: which should be used for new code.
|
| Notes
| -----
| The probability density for the Gaussian distribution is
|
| .. math:: p(x) = \frac{1}{\sqrt{ 2 \pi \sigma^2 }}
| e^{ - \frac{ (x - \mu)^2 } {2 \sigma^2} },
|
| where :math:`\mu` is the mean and :math:`\sigma` the standard
| deviation. The square of the standard deviation, :math:`\sigma^2`,
| is called the variance.
|
| The function has its peak at the mean, and its "spread" increases with
| the standard deviation (the function reaches 0.607 times its maximum at
| :math:`x + \sigma` and :math:`x - \sigma` [2]_). This implies that
| normal is more likely to return samples lying close to the mean, rather
| than those far away.
|
| References
| ----------
| .. [1] Wikipedia, "Normal distribution",
| https://en.wikipedia.org/wiki/Normal_distribution
| .. [2] P. R. Peebles Jr., "Central Limit Theorem" in "Probability,
| Random Variables and Random Signal Principles", 4th ed., 2001,
| pp. 51, 51, 125.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> mu, sigma = 0, 0.1 # mean and standard deviation
| >>> s = np.random.normal(mu, sigma, 1000)
|
| Verify the mean and the variance:
|
| >>> abs(mu - np.mean(s))
| 0.0 # may vary
|
| >>> abs(sigma - np.std(s, ddof=1))
| 0.1 # may vary
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 30, density=True)
| >>> plt.plot(bins, 1/(sigma * np.sqrt(2 * np.pi)) *
| ... np.exp( - (bins - mu)**2 / (2 * sigma**2) ),
| ... linewidth=2, color='r')
| >>> plt.show()
|
| Two-by-four array of samples from the normal distribution with
| mean 3 and standard deviation 2.5:
|
| >>> np.random.normal(3, 2.5, size=(2, 4))
| array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
| [ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
|
| pareto(...)
| pareto(a, size=None)
|
| Draw samples from a Pareto II or Lomax distribution with
| specified shape.
|
| The Lomax or Pareto II distribution is a shifted Pareto
| distribution. The classical Pareto distribution can be
| obtained from the Lomax distribution by adding 1 and
| multiplying by the scale parameter ``m`` (see Notes). The
| smallest value of the Lomax distribution is zero while for the
| classical Pareto distribution it is ``mu``, where the standard
| Pareto distribution has location ``mu = 1``. Lomax can also
| be considered as a simplified version of the Generalized
| Pareto distribution (available in SciPy), with the scale set
| to one and the location set to zero.
|
| The Pareto distribution must be greater than zero, and is
| unbounded above. It is also known as the "80-20 rule". In
| this distribution, 80 percent of the weights are in the lowest
| 20 percent of the range, while the other 20 percent fill the
| remaining 80 percent of the range.
|
| .. note::
| New code should use the `~numpy.random.Generator.pareto`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| a : float or array_like of floats
| Shape of the distribution. Must be positive.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Pareto distribution.
|
| See Also
| --------
| scipy.stats.lomax : probability density function, distribution or
| cumulative density function, etc.
| scipy.stats.genpareto : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.pareto: which should be used for new code.
|
| Notes
| -----
| The probability density for the Pareto distribution is
|
| .. math:: p(x) = \frac{am^a}{x^{a+1}}
|
| where :math:`a` is the shape and :math:`m` the scale.
|
| The Pareto distribution, named after the Italian economist
| Vilfredo Pareto, is a power law probability distribution
| useful in many real world problems. Outside the field of
| economics it is generally referred to as the Bradford
| distribution. Pareto developed the distribution to describe
| the distribution of wealth in an economy. It has also found
| use in insurance, web page access statistics, oil field sizes,
| and many other problems, including the download frequency for
| projects in Sourceforge [1]_. It is one of the so-called
| "fat-tailed" distributions.
|
| References
| ----------
| .. [1] Francis Hunt and Paul Johnson, On the Pareto Distribution of
| Sourceforge projects.
| .. [2] Pareto, V. (1896). Course of Political Economy. Lausanne.
| .. [3] Reiss, R.D., Thomas, M.(2001), Statistical Analysis of Extreme
| Values, Birkhauser Verlag, Basel, pp 23-30.
| .. [4] Wikipedia, "Pareto distribution",
| https://en.wikipedia.org/wiki/Pareto_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a, m = 3., 2. # shape and mode
| >>> s = (np.random.pareto(a, 1000) + 1) * m
|
| Display the histogram of the samples, along with the probability
| density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, _ = plt.hist(s, 100, density=True)
| >>> fit = a*m**a / bins**(a+1)
| >>> plt.plot(bins, max(count)*fit/max(fit), linewidth=2, color='r')
| >>> plt.show()
|
| permutation(...)
| permutation(x)
|
| Randomly permute a sequence, or return a permuted range.
|
| If `x` is a multi-dimensional array, it is only shuffled along its
| first index.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.permutation`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| x : int or array_like
| If `x` is an integer, randomly permute ``np.arange(x)``.
| If `x` is an array, make a copy and shuffle the elements
| randomly.
|
| Returns
| -------
| out : ndarray
| Permuted sequence or array range.
|
| See Also
| --------
| random.Generator.permutation: which should be used for new code.
|
| Examples
| --------
| >>> np.random.permutation(10)
| array([1, 7, 4, 3, 0, 9, 2, 5, 8, 6]) # random
|
| >>> np.random.permutation([1, 4, 9, 12, 15])
| array([15, 1, 9, 4, 12]) # random
|
| >>> arr = np.arange(9).reshape((3, 3))
| >>> np.random.permutation(arr)
| array([[6, 7, 8], # random
| [0, 1, 2],
| [3, 4, 5]])
|
| poisson(...)
| poisson(lam=1.0, size=None)
|
| Draw samples from a Poisson distribution.
|
| The Poisson distribution is the limit of the binomial distribution
| for large N.
|
| .. note::
| New code should use the `~numpy.random.Generator.poisson`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| lam : float or array_like of floats
| Expected number of events occurring in a fixed-time interval,
| must be >= 0. A sequence must be broadcastable over the requested
| size.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``lam`` is a scalar. Otherwise,
| ``np.array(lam).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Poisson distribution.
|
| See Also
| --------
| random.Generator.poisson: which should be used for new code.
|
| Notes
| -----
| The Poisson distribution
|
| .. math:: f(k; \lambda)=\frac{\lambda^k e^{-\lambda}}{k!}
|
| For events with an expected separation :math:`\lambda` the Poisson
| distribution :math:`f(k; \lambda)` describes the probability of
| :math:`k` events occurring within the observed
| interval :math:`\lambda`.
|
| Because the output is limited to the range of the C int64 type, a
| ValueError is raised when `lam` is within 10 sigma of the maximum
| representable value.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Poisson Distribution."
| From MathWorld--A Wolfram Web Resource.
| http://mathworld.wolfram.com/PoissonDistribution.html
| .. [2] Wikipedia, "Poisson distribution",
| https://en.wikipedia.org/wiki/Poisson_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> import numpy as np
| >>> s = np.random.poisson(5, 10000)
|
| Display histogram of the sample:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 14, density=True)
| >>> plt.show()
|
| Draw each 100 values for lambda 100 and 500:
|
| >>> s = np.random.poisson(lam=(100., 500.), size=(100, 2))
|
| power(...)
| power(a, size=None)
|
| Draws samples in [0, 1] from a power distribution with positive
| exponent a - 1.
|
| Also known as the power function distribution.
|
| .. note::
| New code should use the `~numpy.random.Generator.power`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| a : float or array_like of floats
| Parameter of the distribution. Must be non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized power distribution.
|
| Raises
| ------
| ValueError
| If a <= 0.
|
| See Also
| --------
| random.Generator.power: which should be used for new code.
|
| Notes
| -----
| The probability density function is
|
| .. math:: P(x; a) = ax^{a-1}, 0 \le x \le 1, a>0.
|
| The power function distribution is just the inverse of the Pareto
| distribution. It may also be seen as a special case of the Beta
| distribution.
|
| It is used, for example, in modeling the over-reporting of insurance
| claims.
|
| References
| ----------
| .. [1] Christian Kleiber, Samuel Kotz, "Statistical size distributions
| in economics and actuarial sciences", Wiley, 2003.
| .. [2] Heckert, N. A. and Filliben, James J. "NIST Handbook 148:
| Dataplot Reference Manual, Volume 2: Let Subcommands and Library
| Functions", National Institute of Standards and Technology
| Handbook Series, June 2003.
| https://www.itl.nist.gov/div898/software/dataplot/refman2/auxillar/powpdf.pdf
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a = 5. # shape
| >>> samples = 1000
| >>> s = np.random.power(a, samples)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, bins=30)
| >>> x = np.linspace(0, 1, 100)
| >>> y = a*x**(a-1.)
| >>> normed_y = samples*np.diff(bins)[0]*y
| >>> plt.plot(x, normed_y)
| >>> plt.show()
|
| Compare the power function distribution to the inverse of the Pareto.
|
| >>> from scipy import stats # doctest: +SKIP
| >>> rvs = np.random.power(5, 1000000)
| >>> rvsp = np.random.pareto(5, 1000000)
| >>> xx = np.linspace(0,1,100)
| >>> powpdf = stats.powerlaw.pdf(xx,5) # doctest: +SKIP
|
| >>> plt.figure()
| >>> plt.hist(rvs, bins=50, density=True)
| >>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
| >>> plt.title('np.random.power(5)')
|
| >>> plt.figure()
| >>> plt.hist(1./(1.+rvsp), bins=50, density=True)
| >>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
| >>> plt.title('inverse of 1 + np.random.pareto(5)')
|
| >>> plt.figure()
| >>> plt.hist(1./(1.+rvsp), bins=50, density=True)
| >>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
| >>> plt.title('inverse of stats.pareto(5)')
|
| rand(...)
| rand(d0, d1, ..., dn)
|
| Random values in a given shape.
|
| .. note::
| This is a convenience function for users porting code from Matlab,
| and wraps `random_sample`. That function takes a
| tuple to specify the size of the output, which is consistent with
| other NumPy functions like `numpy.zeros` and `numpy.ones`.
|
| Create an array of the given shape and populate it with
| random samples from a uniform distribution
| over ``[0, 1)``.
|
| Parameters
| ----------
| d0, d1, ..., dn : int, optional
| The dimensions of the returned array, must be non-negative.
| If no argument is given a single Python float is returned.
|
| Returns
| -------
| out : ndarray, shape ``(d0, d1, ..., dn)``
| Random values.
|
| See Also
| --------
| random
|
| Examples
| --------
| >>> np.random.rand(3,2)
| array([[ 0.14022471, 0.96360618], #random
| [ 0.37601032, 0.25528411], #random
| [ 0.49313049, 0.94909878]]) #random
|
| randint(...)
| randint(low, high=None, size=None, dtype=int)
|
| Return random integers from `low` (inclusive) to `high` (exclusive).
|
| Return random integers from the "discrete uniform" distribution of
| the specified dtype in the "half-open" interval [`low`, `high`). If
| `high` is None (the default), then results are from [0, `low`).
|
| .. note::
| New code should use the `~numpy.random.Generator.integers`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| low : int or array-like of ints
| Lowest (signed) integers to be drawn from the distribution (unless
| ``high=None``, in which case this parameter is one above the
| *highest* such integer).
| high : int or array-like of ints, optional
| If provided, one above the largest (signed) integer to be drawn
| from the distribution (see above for behavior if ``high=None``).
| If array-like, must contain integer values
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| dtype : dtype, optional
| Desired dtype of the result. Byteorder must be native.
| The default value is int.
|
| .. versionadded:: 1.11.0
|
| Returns
| -------
| out : int or ndarray of ints
| `size`-shaped array of random integers from the appropriate
| distribution, or a single such random int if `size` not provided.
|
| See Also
| --------
| random_integers : similar to `randint`, only for the closed
| interval [`low`, `high`], and 1 is the lowest value if `high` is
| omitted.
| random.Generator.integers: which should be used for new code.
|
| Examples
| --------
| >>> np.random.randint(2, size=10)
| array([1, 0, 0, 0, 1, 1, 0, 0, 1, 0]) # random
| >>> np.random.randint(1, size=10)
| array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0])
|
| Generate a 2 x 4 array of ints between 0 and 4, inclusive:
|
| >>> np.random.randint(5, size=(2, 4))
| array([[4, 0, 2, 1], # random
| [3, 2, 2, 0]])
|
| Generate a 1 x 3 array with 3 different upper bounds
|
| >>> np.random.randint(1, [3, 5, 10])
| array([2, 2, 9]) # random
|
| Generate a 1 by 3 array with 3 different lower bounds
|
| >>> np.random.randint([1, 5, 7], 10)
| array([9, 8, 7]) # random
|
| Generate a 2 by 4 array using broadcasting with dtype of uint8
|
| >>> np.random.randint([1, 3, 5, 7], [[10], [20]], dtype=np.uint8)
| array([[ 8, 6, 9, 7], # random
| [ 1, 16, 9, 12]], dtype=uint8)
|
| randn(...)
| randn(d0, d1, ..., dn)
|
| Return a sample (or samples) from the "standard normal" distribution.
|
| .. note::
| This is a convenience function for users porting code from Matlab,
| and wraps `standard_normal`. That function takes a
| tuple to specify the size of the output, which is consistent with
| other NumPy functions like `numpy.zeros` and `numpy.ones`.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.standard_normal`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| If positive int_like arguments are provided, `randn` generates an array
| of shape ``(d0, d1, ..., dn)``, filled
| with random floats sampled from a univariate "normal" (Gaussian)
| distribution of mean 0 and variance 1. A single float randomly sampled
| from the distribution is returned if no argument is provided.
|
| Parameters
| ----------
| d0, d1, ..., dn : int, optional
| The dimensions of the returned array, must be non-negative.
| If no argument is given a single Python float is returned.
|
| Returns
| -------
| Z : ndarray or float
| A ``(d0, d1, ..., dn)``-shaped array of floating-point samples from
| the standard normal distribution, or a single such float if
| no parameters were supplied.
|
| See Also
| --------
| standard_normal : Similar, but takes a tuple as its argument.
| normal : Also accepts mu and sigma arguments.
| random.Generator.standard_normal: which should be used for new code.
|
| Notes
| -----
| For random samples from the normal distribution with mean ``mu`` and
| standard deviation ``sigma``, use::
|
| sigma * np.random.randn(...) + mu
|
| Examples
| --------
| >>> np.random.randn()
| 2.1923875335537315 # random
|
| Two-by-four array of samples from the normal distribution with
| mean 3 and standard deviation 2.5:
|
| >>> 3 + 2.5 * np.random.randn(2, 4)
| array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
| [ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
|
| random(...)
| random(size=None)
|
| Return random floats in the half-open interval [0.0, 1.0). Alias for
| `random_sample` to ease forward-porting to the new random API.
|
| random_integers(...)
| random_integers(low, high=None, size=None)
|
| Random integers of type `np.int_` between `low` and `high`, inclusive.
|
| Return random integers of type `np.int_` from the "discrete uniform"
| distribution in the closed interval [`low`, `high`]. If `high` is
| None (the default), then results are from [1, `low`]. The `np.int_`
| type translates to the C long integer type and its precision
| is platform dependent.
|
| This function has been deprecated. Use randint instead.
|
| .. deprecated:: 1.11.0
|
| Parameters
| ----------
| low : int
| Lowest (signed) integer to be drawn from the distribution (unless
| ``high=None``, in which case this parameter is the *highest* such
| integer).
| high : int, optional
| If provided, the largest (signed) integer to be drawn from the
| distribution (see above for behavior if ``high=None``).
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| out : int or ndarray of ints
| `size`-shaped array of random integers from the appropriate
| distribution, or a single such random int if `size` not provided.
|
| See Also
| --------
| randint : Similar to `random_integers`, only for the half-open
| interval [`low`, `high`), and 0 is the lowest value if `high` is
| omitted.
|
| Notes
| -----
| To sample from N evenly spaced floating-point numbers between a and b,
| use::
|
| a + (b - a) * (np.random.random_integers(N) - 1) / (N - 1.)
|
| Examples
| --------
| >>> np.random.random_integers(5)
| 4 # random
| >>> type(np.random.random_integers(5))
| <class 'numpy.int64'>
| >>> np.random.random_integers(5, size=(3,2))
| array([[5, 4], # random
| [3, 3],
| [4, 5]])
|
| Choose five random numbers from the set of five evenly-spaced
| numbers between 0 and 2.5, inclusive (*i.e.*, from the set
| :math:`{0, 5/8, 10/8, 15/8, 20/8}`):
|
| >>> 2.5 * (np.random.random_integers(5, size=(5,)) - 1) / 4.
| array([ 0.625, 1.25 , 0.625, 0.625, 2.5 ]) # random
|
| Roll two six sided dice 1000 times and sum the results:
|
| >>> d1 = np.random.random_integers(1, 6, 1000)
| >>> d2 = np.random.random_integers(1, 6, 1000)
| >>> dsums = d1 + d2
|
| Display results as a histogram:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(dsums, 11, density=True)
| >>> plt.show()
|
| random_sample(...)
| random_sample(size=None)
|
| Return random floats in the half-open interval [0.0, 1.0).
|
| Results are from the "continuous uniform" distribution over the
| stated interval. To sample :math:`Unif[a, b), b > a` multiply
| the output of `random_sample` by `(b-a)` and add `a`::
|
| (b - a) * random_sample() + a
|
| .. note::
| New code should use the `~numpy.random.Generator.random`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| out : float or ndarray of floats
| Array of random floats of shape `size` (unless ``size=None``, in which
| case a single float is returned).
|
| See Also
| --------
| random.Generator.random: which should be used for new code.
|
| Examples
| --------
| >>> np.random.random_sample()
| 0.47108547995356098 # random
| >>> type(np.random.random_sample())
| <class 'float'>
| >>> np.random.random_sample((5,))
| array([ 0.30220482, 0.86820401, 0.1654503 , 0.11659149, 0.54323428]) # random
|
| Three-by-two array of random numbers from [-5, 0):
|
| >>> 5 * np.random.random_sample((3, 2)) - 5
| array([[-3.99149989, -0.52338984], # random
| [-2.99091858, -0.79479508],
| [-1.23204345, -1.75224494]])
|
| rayleigh(...)
| rayleigh(scale=1.0, size=None)
|
| Draw samples from a Rayleigh distribution.
|
| The :math:`\chi` and Weibull distributions are generalizations of the
| Rayleigh.
|
| .. note::
| New code should use the `~numpy.random.Generator.rayleigh`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| scale : float or array_like of floats, optional
| Scale, also equals the mode. Must be non-negative. Default is 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``scale`` is a scalar. Otherwise,
| ``np.array(scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Rayleigh distribution.
|
| See Also
| --------
| random.Generator.rayleigh: which should be used for new code.
|
| Notes
| -----
| The probability density function for the Rayleigh distribution is
|
| .. math:: P(x;scale) = \frac{x}{scale^2}e^{\frac{-x^2}{2 \cdotp scale^2}}
|
| The Rayleigh distribution would arise, for example, if the East
| and North components of the wind velocity had identical zero-mean
| Gaussian distributions. Then the wind speed would have a Rayleigh
| distribution.
|
| References
| ----------
| .. [1] Brighton Webs Ltd., "Rayleigh Distribution,"
| https://web.archive.org/web/20090514091424/http://brighton-webs.co.uk:80/distributions/rayleigh.asp
| .. [2] Wikipedia, "Rayleigh distribution"
| https://en.wikipedia.org/wiki/Rayleigh_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram
|
| >>> from matplotlib.pyplot import hist
| >>> values = hist(np.random.rayleigh(3, 100000), bins=200, density=True)
|
| Wave heights tend to follow a Rayleigh distribution. If the mean wave
| height is 1 meter, what fraction of waves are likely to be larger than 3
| meters?
|
| >>> meanvalue = 1
| >>> modevalue = np.sqrt(2 / np.pi) * meanvalue
| >>> s = np.random.rayleigh(modevalue, 1000000)
|
| The percentage of waves larger than 3 meters is:
|
| >>> 100.*sum(s>3)/1000000.
| 0.087300000000000003 # random
|
| seed(...)
| seed(seed=None)
|
| Reseed a legacy MT19937 BitGenerator
|
| Notes
| -----
| This is a convenience, legacy function.
|
| The best practice is to **not** reseed a BitGenerator, rather to
| recreate a new one. This method is here for legacy reasons.
| This example demonstrates best practice.
|
| >>> from numpy.random import MT19937
| >>> from numpy.random import RandomState, SeedSequence
| >>> rs = RandomState(MT19937(SeedSequence(123456789)))
| # Later, you want to restart the stream
| >>> rs = RandomState(MT19937(SeedSequence(987654321)))
|
| set_state(...)
| set_state(state)
|
| Set the internal state of the generator from a tuple.
|
| For use if one has reason to manually (re-)set the internal state of
| the bit generator used by the RandomState instance. By default,
| RandomState uses the "Mersenne Twister"[1]_ pseudo-random number
| generating algorithm.
|
| Parameters
| ----------
| state : {tuple(str, ndarray of 624 uints, int, int, float), dict}
| The `state` tuple has the following items:
|
| 1. the string 'MT19937', specifying the Mersenne Twister algorithm.
| 2. a 1-D array of 624 unsigned integers ``keys``.
| 3. an integer ``pos``.
| 4. an integer ``has_gauss``.
| 5. a float ``cached_gaussian``.
|
| If state is a dictionary, it is directly set using the BitGenerators
| `state` property.
|
| Returns
| -------
| out : None
| Returns 'None' on success.
|
| See Also
| --------
| get_state
|
| Notes
| -----
| `set_state` and `get_state` are not needed to work with any of the
| random distributions in NumPy. If the internal state is manually altered,
| the user should know exactly what he/she is doing.
|
| For backwards compatibility, the form (str, array of 624 uints, int) is
| also accepted although it is missing some information about the cached
| Gaussian value: ``state = ('MT19937', keys, pos)``.
|
| References
| ----------
| .. [1] M. Matsumoto and T. Nishimura, "Mersenne Twister: A
| 623-dimensionally equidistributed uniform pseudorandom number
| generator," *ACM Trans. on Modeling and Computer Simulation*,
| Vol. 8, No. 1, pp. 3-30, Jan. 1998.
|
| shuffle(...)
| shuffle(x)
|
| Modify a sequence in-place by shuffling its contents.
|
| This function only shuffles the array along the first axis of a
| multi-dimensional array. The order of sub-arrays is changed but
| their contents remains the same.
|
| .. note::
| New code should use the `~numpy.random.Generator.shuffle`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| x : ndarray or MutableSequence
| The array, list or mutable sequence to be shuffled.
|
| Returns
| -------
| None
|
| See Also
| --------
| random.Generator.shuffle: which should be used for new code.
|
| Examples
| --------
| >>> arr = np.arange(10)
| >>> np.random.shuffle(arr)
| >>> arr
| [1 7 5 2 9 4 3 6 0 8] # random
|
| Multi-dimensional arrays are only shuffled along the first axis:
|
| >>> arr = np.arange(9).reshape((3, 3))
| >>> np.random.shuffle(arr)
| >>> arr
| array([[3, 4, 5], # random
| [6, 7, 8],
| [0, 1, 2]])
|
| standard_cauchy(...)
| standard_cauchy(size=None)
|
| Draw samples from a standard Cauchy distribution with mode = 0.
|
| Also known as the Lorentz distribution.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.standard_cauchy`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| samples : ndarray or scalar
| The drawn samples.
|
| See Also
| --------
| random.Generator.standard_cauchy: which should be used for new code.
|
| Notes
| -----
| The probability density function for the full Cauchy distribution is
|
| .. math:: P(x; x_0, \gamma) = \frac{1}{\pi \gamma \bigl[ 1+
| (\frac{x-x_0}{\gamma})^2 \bigr] }
|
| and the Standard Cauchy distribution just sets :math:`x_0=0` and
| :math:`\gamma=1`
|
| The Cauchy distribution arises in the solution to the driven harmonic
| oscillator problem, and also describes spectral line broadening. It
| also describes the distribution of values at which a line tilted at
| a random angle will cut the x axis.
|
| When studying hypothesis tests that assume normality, seeing how the
| tests perform on data from a Cauchy distribution is a good indicator of
| their sensitivity to a heavy-tailed distribution, since the Cauchy looks
| very much like a Gaussian distribution, but with heavier tails.
|
| References
| ----------
| .. [1] NIST/SEMATECH e-Handbook of Statistical Methods, "Cauchy
| Distribution",
| https://www.itl.nist.gov/div898/handbook/eda/section3/eda3663.htm
| .. [2] Weisstein, Eric W. "Cauchy Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/CauchyDistribution.html
| .. [3] Wikipedia, "Cauchy distribution"
| https://en.wikipedia.org/wiki/Cauchy_distribution
|
| Examples
| --------
| Draw samples and plot the distribution:
|
| >>> import matplotlib.pyplot as plt
| >>> s = np.random.standard_cauchy(1000000)
| >>> s = s[(s>-25) & (s<25)] # truncate distribution so it plots well
| >>> plt.hist(s, bins=100)
| >>> plt.show()
|
| standard_exponential(...)
| standard_exponential(size=None)
|
| Draw samples from the standard exponential distribution.
|
| `standard_exponential` is identical to the exponential distribution
| with a scale parameter of 1.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.standard_exponential`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| out : float or ndarray
| Drawn samples.
|
| See Also
| --------
| random.Generator.standard_exponential: which should be used for new code.
|
| Examples
| --------
| Output a 3x8000 array:
|
| >>> n = np.random.standard_exponential((3, 8000))
|
| standard_gamma(...)
| standard_gamma(shape, size=None)
|
| Draw samples from a standard Gamma distribution.
|
| Samples are drawn from a Gamma distribution with specified parameters,
| shape (sometimes designated "k") and scale=1.
|
| .. note::
| New code should use the
| `~numpy.random.Generator.standard_gamma`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| shape : float or array_like of floats
| Parameter, must be non-negative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``shape`` is a scalar. Otherwise,
| ``np.array(shape).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized standard gamma distribution.
|
| See Also
| --------
| scipy.stats.gamma : probability density function, distribution or
| cumulative density function, etc.
| random.Generator.standard_gamma: which should be used for new code.
|
| Notes
| -----
| The probability density for the Gamma distribution is
|
| .. math:: p(x) = x^{k-1}\frac{e^{-x/\theta}}{\theta^k\Gamma(k)},
|
| where :math:`k` is the shape and :math:`\theta` the scale,
| and :math:`\Gamma` is the Gamma function.
|
| The Gamma distribution is often used to model the times to failure of
| electronic components, and arises naturally in processes for which the
| waiting times between Poisson distributed events are relevant.
|
| References
| ----------
| .. [1] Weisstein, Eric W. "Gamma Distribution." From MathWorld--A
| Wolfram Web Resource.
| http://mathworld.wolfram.com/GammaDistribution.html
| .. [2] Wikipedia, "Gamma distribution",
| https://en.wikipedia.org/wiki/Gamma_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> shape, scale = 2., 1. # mean and width
| >>> s = np.random.standard_gamma(shape, 1000000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> import scipy.special as sps # doctest: +SKIP
| >>> count, bins, ignored = plt.hist(s, 50, density=True)
| >>> y = bins**(shape-1) * ((np.exp(-bins/scale))/ # doctest: +SKIP
| ... (sps.gamma(shape) * scale**shape))
| >>> plt.plot(bins, y, linewidth=2, color='r') # doctest: +SKIP
| >>> plt.show()
|
| standard_normal(...)
| standard_normal(size=None)
|
| Draw samples from a standard Normal distribution (mean=0, stdev=1).
|
| .. note::
| New code should use the
| `~numpy.random.Generator.standard_normal`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| out : float or ndarray
| A floating-point array of shape ``size`` of drawn samples, or a
| single sample if ``size`` was not specified.
|
| See Also
| --------
| normal :
| Equivalent function with additional ``loc`` and ``scale`` arguments
| for setting the mean and standard deviation.
| random.Generator.standard_normal: which should be used for new code.
|
| Notes
| -----
| For random samples from the normal distribution with mean ``mu`` and
| standard deviation ``sigma``, use one of::
|
| mu + sigma * np.random.standard_normal(size=...)
| np.random.normal(mu, sigma, size=...)
|
| Examples
| --------
| >>> np.random.standard_normal()
| 2.1923875335537315 #random
|
| >>> s = np.random.standard_normal(8000)
| >>> s
| array([ 0.6888893 , 0.78096262, -0.89086505, ..., 0.49876311, # random
| -0.38672696, -0.4685006 ]) # random
| >>> s.shape
| (8000,)
| >>> s = np.random.standard_normal(size=(3, 4, 2))
| >>> s.shape
| (3, 4, 2)
|
| Two-by-four array of samples from the normal distribution with
| mean 3 and standard deviation 2.5:
|
| >>> 3 + 2.5 * np.random.standard_normal(size=(2, 4))
| array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
| [ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
|
| standard_t(...)
| standard_t(df, size=None)
|
| Draw samples from a standard Student's t distribution with `df` degrees
| of freedom.
|
| A special case of the hyperbolic distribution. As `df` gets
| large, the result resembles that of the standard normal
| distribution (`standard_normal`).
|
| .. note::
| New code should use the `~numpy.random.Generator.standard_t`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| df : float or array_like of floats
| Degrees of freedom, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``df`` is a scalar. Otherwise,
| ``np.array(df).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized standard Student's t distribution.
|
| See Also
| --------
| random.Generator.standard_t: which should be used for new code.
|
| Notes
| -----
| The probability density function for the t distribution is
|
| .. math:: P(x, df) = \frac{\Gamma(\frac{df+1}{2})}{\sqrt{\pi df}
| \Gamma(\frac{df}{2})}\Bigl( 1+\frac{x^2}{df} \Bigr)^{-(df+1)/2}
|
| The t test is based on an assumption that the data come from a
| Normal distribution. The t test provides a way to test whether
| the sample mean (that is the mean calculated from the data) is
| a good estimate of the true mean.
|
| The derivation of the t-distribution was first published in
| 1908 by William Gosset while working for the Guinness Brewery
| in Dublin. Due to proprietary issues, he had to publish under
| a pseudonym, and so he used the name Student.
|
| References
| ----------
| .. [1] Dalgaard, Peter, "Introductory Statistics With R",
| Springer, 2002.
| .. [2] Wikipedia, "Student's t-distribution"
| https://en.wikipedia.org/wiki/Student's_t-distribution
|
| Examples
| --------
| From Dalgaard page 83 [1]_, suppose the daily energy intake for 11
| women in kilojoules (kJ) is:
|
| >>> intake = np.array([5260., 5470, 5640, 6180, 6390, 6515, 6805, 7515, \
| ... 7515, 8230, 8770])
|
| Does their energy intake deviate systematically from the recommended
| value of 7725 kJ? Our null hypothesis will be the absence of deviation,
| and the alternate hypothesis will be the presence of an effect that could be
| either positive or negative, hence making our test 2-tailed.
|
| Because we are estimating the mean and we have N=11 values in our sample,
| we have N-1=10 degrees of freedom. We set our significance level to 95% and
| compute the t statistic using the empirical mean and empirical standard
| deviation of our intake. We use a ddof of 1 to base the computation of our
| empirical standard deviation on an unbiased estimate of the variance (note:
| the final estimate is not unbiased due to the concave nature of the square
| root).
|
| >>> np.mean(intake)
| 6753.636363636364
| >>> intake.std(ddof=1)
| 1142.1232221373727
| >>> t = (np.mean(intake)-7725)/(intake.std(ddof=1)/np.sqrt(len(intake)))
| >>> t
| -2.8207540608310198
|
| We draw 1000000 samples from Student's t distribution with the adequate
| degrees of freedom.
|
| >>> import matplotlib.pyplot as plt
| >>> s = np.random.standard_t(10, size=1000000)
| >>> h = plt.hist(s, bins=100, density=True)
|
| Does our t statistic land in one of the two critical regions found at
| both tails of the distribution?
|
| >>> np.sum(np.abs(t) < np.abs(s)) / float(len(s))
| 0.018318 #random < 0.05, statistic is in critical region
|
| The probability value for this 2-tailed test is about 1.83%, which is
| lower than the 5% pre-determined significance threshold.
|
| Therefore, the probability of observing values as extreme as our intake
| conditionally on the null hypothesis being true is too low, and we reject
| the null hypothesis of no deviation.
|
| tomaxint(...)
| tomaxint(size=None)
|
| Return a sample of uniformly distributed random integers in the interval
| [0, ``np.iinfo(np.int_).max``]. The `np.int_` type translates to the C long
| integer type and its precision is platform dependent.
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
|
| Returns
| -------
| out : ndarray
| Drawn samples, with shape `size`.
|
| See Also
| --------
| randint : Uniform sampling over a given half-open interval of integers.
| random_integers : Uniform sampling over a given closed interval of
| integers.
|
| Examples
| --------
| >>> rs = np.random.RandomState() # need a RandomState object
| >>> rs.tomaxint((2,2,2))
| array([[[1170048599, 1600360186], # random
| [ 739731006, 1947757578]],
| [[1871712945, 752307660],
| [1601631370, 1479324245]]])
| >>> rs.tomaxint((2,2,2)) < np.iinfo(np.int_).max
| array([[[ True, True],
| [ True, True]],
| [[ True, True],
| [ True, True]]])
|
| triangular(...)
| triangular(left, mode, right, size=None)
|
| Draw samples from the triangular distribution over the
| interval ``[left, right]``.
|
| The triangular distribution is a continuous probability
| distribution with lower limit left, peak at mode, and upper
| limit right. Unlike the other distributions, these parameters
| directly define the shape of the pdf.
|
| .. note::
| New code should use the `~numpy.random.Generator.triangular`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| left : float or array_like of floats
| Lower limit.
| mode : float or array_like of floats
| The value where the peak of the distribution occurs.
| The value must fulfill the condition ``left <= mode <= right``.
| right : float or array_like of floats
| Upper limit, must be larger than `left`.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``left``, ``mode``, and ``right``
| are all scalars. Otherwise, ``np.broadcast(left, mode, right).size``
| samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized triangular distribution.
|
| See Also
| --------
| random.Generator.triangular: which should be used for new code.
|
| Notes
| -----
| The probability density function for the triangular distribution is
|
| .. math:: P(x;l, m, r) = \begin{cases}
| \frac{2(x-l)}{(r-l)(m-l)}& \text{for $l \leq x \leq m$},\\
| \frac{2(r-x)}{(r-l)(r-m)}& \text{for $m \leq x \leq r$},\\
| 0& \text{otherwise}.
| \end{cases}
|
| The triangular distribution is often used in ill-defined
| problems where the underlying distribution is not known, but
| some knowledge of the limits and mode exists. Often it is used
| in simulations.
|
| References
| ----------
| .. [1] Wikipedia, "Triangular distribution"
| https://en.wikipedia.org/wiki/Triangular_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram:
|
| >>> import matplotlib.pyplot as plt
| >>> h = plt.hist(np.random.triangular(-3, 0, 8, 100000), bins=200,
| ... density=True)
| >>> plt.show()
|
| uniform(...)
| uniform(low=0.0, high=1.0, size=None)
|
| Draw samples from a uniform distribution.
|
| Samples are uniformly distributed over the half-open interval
| ``[low, high)`` (includes low, but excludes high). In other words,
| any value within the given interval is equally likely to be drawn
| by `uniform`.
|
| .. note::
| New code should use the `~numpy.random.Generator.uniform`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| low : float or array_like of floats, optional
| Lower boundary of the output interval. All values generated will be
| greater than or equal to low. The default value is 0.
| high : float or array_like of floats
| Upper boundary of the output interval. All values generated will be
| less than or equal to high. The high limit may be included in the
| returned array of floats due to floating-point rounding in the
| equation ``low + (high-low) * random_sample()``. The default value
| is 1.0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``low`` and ``high`` are both scalars.
| Otherwise, ``np.broadcast(low, high).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized uniform distribution.
|
| See Also
| --------
| randint : Discrete uniform distribution, yielding integers.
| random_integers : Discrete uniform distribution over the closed
| interval ``[low, high]``.
| random_sample : Floats uniformly distributed over ``[0, 1)``.
| random : Alias for `random_sample`.
| rand : Convenience function that accepts dimensions as input, e.g.,
| ``rand(2,2)`` would generate a 2-by-2 array of floats,
| uniformly distributed over ``[0, 1)``.
| random.Generator.uniform: which should be used for new code.
|
| Notes
| -----
| The probability density function of the uniform distribution is
|
| .. math:: p(x) = \frac{1}{b - a}
|
| anywhere within the interval ``[a, b)``, and zero elsewhere.
|
| When ``high`` == ``low``, values of ``low`` will be returned.
| If ``high`` < ``low``, the results are officially undefined
| and may eventually raise an error, i.e. do not rely on this
| function to behave when passed arguments satisfying that
| inequality condition. The ``high`` limit may be included in the
| returned array of floats due to floating-point rounding in the
| equation ``low + (high-low) * random_sample()``. For example:
|
| >>> x = np.float32(5*0.99999999)
| >>> x
| 5.0
|
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> s = np.random.uniform(-1,0,1000)
|
| All values are within the given interval:
|
| >>> np.all(s >= -1)
| True
| >>> np.all(s < 0)
| True
|
| Display the histogram of the samples, along with the
| probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> count, bins, ignored = plt.hist(s, 15, density=True)
| >>> plt.plot(bins, np.ones_like(bins), linewidth=2, color='r')
| >>> plt.show()
|
| vonmises(...)
| vonmises(mu, kappa, size=None)
|
| Draw samples from a von Mises distribution.
|
| Samples are drawn from a von Mises distribution with specified mode
| (mu) and dispersion (kappa), on the interval [-pi, pi].
|
| The von Mises distribution (also known as the circular normal
| distribution) is a continuous probability distribution on the unit
| circle. It may be thought of as the circular analogue of the normal
| distribution.
|
| .. note::
| New code should use the `~numpy.random.Generator.vonmises`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| mu : float or array_like of floats
| Mode ("center") of the distribution.
| kappa : float or array_like of floats
| Dispersion of the distribution, has to be >=0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``mu`` and ``kappa`` are both scalars.
| Otherwise, ``np.broadcast(mu, kappa).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized von Mises distribution.
|
| See Also
| --------
| scipy.stats.vonmises : probability density function, distribution, or
| cumulative density function, etc.
| random.Generator.vonmises: which should be used for new code.
|
| Notes
| -----
| The probability density for the von Mises distribution is
|
| .. math:: p(x) = \frac{e^{\kappa cos(x-\mu)}}{2\pi I_0(\kappa)},
|
| where :math:`\mu` is the mode and :math:`\kappa` the dispersion,
| and :math:`I_0(\kappa)` is the modified Bessel function of order 0.
|
| The von Mises is named for Richard Edler von Mises, who was born in
| Austria-Hungary, in what is now the Ukraine. He fled to the United
| States in 1939 and became a professor at Harvard. He worked in
| probability theory, aerodynamics, fluid mechanics, and philosophy of
| science.
|
| References
| ----------
| .. [1] Abramowitz, M. and Stegun, I. A. (Eds.). "Handbook of
| Mathematical Functions with Formulas, Graphs, and Mathematical
| Tables, 9th printing," New York: Dover, 1972.
| .. [2] von Mises, R., "Mathematical Theory of Probability
| and Statistics", New York: Academic Press, 1964.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> mu, kappa = 0.0, 4.0 # mean and dispersion
| >>> s = np.random.vonmises(mu, kappa, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> from scipy.special import i0 # doctest: +SKIP
| >>> plt.hist(s, 50, density=True)
| >>> x = np.linspace(-np.pi, np.pi, num=51)
| >>> y = np.exp(kappa*np.cos(x-mu))/(2*np.pi*i0(kappa)) # doctest: +SKIP
| >>> plt.plot(x, y, linewidth=2, color='r') # doctest: +SKIP
| >>> plt.show()
|
| wald(...)
| wald(mean, scale, size=None)
|
| Draw samples from a Wald, or inverse Gaussian, distribution.
|
| As the scale approaches infinity, the distribution becomes more like a
| Gaussian. Some references claim that the Wald is an inverse Gaussian
| with mean equal to 1, but this is by no means universal.
|
| The inverse Gaussian distribution was first studied in relationship to
| Brownian motion. In 1956 M.C.K. Tweedie used the name inverse Gaussian
| because there is an inverse relationship between the time to cover a
| unit distance and distance covered in unit time.
|
| .. note::
| New code should use the `~numpy.random.Generator.wald`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| mean : float or array_like of floats
| Distribution mean, must be > 0.
| scale : float or array_like of floats
| Scale parameter, must be > 0.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``mean`` and ``scale`` are both scalars.
| Otherwise, ``np.broadcast(mean, scale).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Wald distribution.
|
| See Also
| --------
| random.Generator.wald: which should be used for new code.
|
| Notes
| -----
| The probability density function for the Wald distribution is
|
| .. math:: P(x;mean,scale) = \sqrt{\frac{scale}{2\pi x^3}}e^
| \frac{-scale(x-mean)^2}{2\cdotp mean^2x}
|
| As noted above the inverse Gaussian distribution first arise
| from attempts to model Brownian motion. It is also a
| competitor to the Weibull for use in reliability modeling and
| modeling stock returns and interest rate processes.
|
| References
| ----------
| .. [1] Brighton Webs Ltd., Wald Distribution,
| https://web.archive.org/web/20090423014010/http://www.brighton-webs.co.uk:80/distributions/wald.asp
| .. [2] Chhikara, Raj S., and Folks, J. Leroy, "The Inverse Gaussian
| Distribution: Theory : Methodology, and Applications", CRC Press,
| 1988.
| .. [3] Wikipedia, "Inverse Gaussian distribution"
| https://en.wikipedia.org/wiki/Inverse_Gaussian_distribution
|
| Examples
| --------
| Draw values from the distribution and plot the histogram:
|
| >>> import matplotlib.pyplot as plt
| >>> h = plt.hist(np.random.wald(3, 2, 100000), bins=200, density=True)
| >>> plt.show()
|
| weibull(...)
| weibull(a, size=None)
|
| Draw samples from a Weibull distribution.
|
| Draw samples from a 1-parameter Weibull distribution with the given
| shape parameter `a`.
|
| .. math:: X = (-ln(U))^{1/a}
|
| Here, U is drawn from the uniform distribution over (0,1].
|
| The more common 2-parameter Weibull, including a scale parameter
| :math:`\lambda` is just :math:`X = \lambda(-ln(U))^{1/a}`.
|
| .. note::
| New code should use the `~numpy.random.Generator.weibull`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| a : float or array_like of floats
| Shape parameter of the distribution. Must be nonnegative.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Weibull distribution.
|
| See Also
| --------
| scipy.stats.weibull_max
| scipy.stats.weibull_min
| scipy.stats.genextreme
| gumbel
| random.Generator.weibull: which should be used for new code.
|
| Notes
| -----
| The Weibull (or Type III asymptotic extreme value distribution
| for smallest values, SEV Type III, or Rosin-Rammler
| distribution) is one of a class of Generalized Extreme Value
| (GEV) distributions used in modeling extreme value problems.
| This class includes the Gumbel and Frechet distributions.
|
| The probability density for the Weibull distribution is
|
| .. math:: p(x) = \frac{a}
| {\lambda}(\frac{x}{\lambda})^{a-1}e^{-(x/\lambda)^a},
|
| where :math:`a` is the shape and :math:`\lambda` the scale.
|
| The function has its peak (the mode) at
| :math:`\lambda(\frac{a-1}{a})^{1/a}`.
|
| When ``a = 1``, the Weibull distribution reduces to the exponential
| distribution.
|
| References
| ----------
| .. [1] Waloddi Weibull, Royal Technical University, Stockholm,
| 1939 "A Statistical Theory Of The Strength Of Materials",
| Ingeniorsvetenskapsakademiens Handlingar Nr 151, 1939,
| Generalstabens Litografiska Anstalts Forlag, Stockholm.
| .. [2] Waloddi Weibull, "A Statistical Distribution Function of
| Wide Applicability", Journal Of Applied Mechanics ASME Paper
| 1951.
| .. [3] Wikipedia, "Weibull distribution",
| https://en.wikipedia.org/wiki/Weibull_distribution
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a = 5. # shape
| >>> s = np.random.weibull(a, 1000)
|
| Display the histogram of the samples, along with
| the probability density function:
|
| >>> import matplotlib.pyplot as plt
| >>> x = np.arange(1,100.)/50.
| >>> def weib(x,n,a):
| ... return (a / n) * (x / n)**(a - 1) * np.exp(-(x / n)**a)
|
| >>> count, bins, ignored = plt.hist(np.random.weibull(5.,1000))
| >>> x = np.arange(1,100.)/50.
| >>> scale = count.max()/weib(x, 1., 5.).max()
| >>> plt.plot(x, weib(x, 1., 5.)*scale)
| >>> plt.show()
|
| zipf(...)
| zipf(a, size=None)
|
| Draw samples from a Zipf distribution.
|
| Samples are drawn from a Zipf distribution with specified parameter
| `a` > 1.
|
| The Zipf distribution (also known as the zeta distribution) is a
| discrete probability distribution that satisfies Zipf's law: the
| frequency of an item is inversely proportional to its rank in a
| frequency table.
|
| .. note::
| New code should use the `~numpy.random.Generator.zipf`
| method of a `~numpy.random.Generator` instance instead;
| please see the :ref:`random-quick-start`.
|
| Parameters
| ----------
| a : float or array_like of floats
| Distribution parameter. Must be greater than 1.
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. If size is ``None`` (default),
| a single value is returned if ``a`` is a scalar. Otherwise,
| ``np.array(a).size`` samples are drawn.
|
| Returns
| -------
| out : ndarray or scalar
| Drawn samples from the parameterized Zipf distribution.
|
| See Also
| --------
| scipy.stats.zipf : probability density function, distribution, or
| cumulative density function, etc.
| random.Generator.zipf: which should be used for new code.
|
| Notes
| -----
| The probability density for the Zipf distribution is
|
| .. math:: p(k) = \frac{k^{-a}}{\zeta(a)},
|
| for integers :math:`k \geq 1`, where :math:`\zeta` is the Riemann Zeta
| function.
|
| It is named for the American linguist George Kingsley Zipf, who noted
| that the frequency of any word in a sample of a language is inversely
| proportional to its rank in the frequency table.
|
| References
| ----------
| .. [1] Zipf, G. K., "Selected Studies of the Principle of Relative
| Frequency in Language," Cambridge, MA: Harvard Univ. Press,
| 1932.
|
| Examples
| --------
| Draw samples from the distribution:
|
| >>> a = 4.0
| >>> n = 20000
| >>> s = np.random.zipf(a, n)
|
| Display the histogram of the samples, along with
| the expected histogram based on the probability
| density function:
|
| >>> import matplotlib.pyplot as plt
| >>> from scipy.special import zeta # doctest: +SKIP
|
| `bincount` provides a fast histogram for small integers.
|
| >>> count = np.bincount(s)
| >>> k = np.arange(1, s.max() + 1)
|
| >>> plt.bar(k, count[1:], alpha=0.5, label='sample count')
| >>> plt.plot(k, n*(k**-a)/zeta(a), 'k.-', alpha=0.5,
| ... label='expected count') # doctest: +SKIP
| >>> plt.semilogy()
| >>> plt.grid(alpha=0.4)
| >>> plt.legend()
| >>> plt.title(f'Zipf sample, a={a}, size={n}')
| >>> plt.show()
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| __pyx_vtable__ = <capsule object NULL>
class SFC64(numpy.random.bit_generator.BitGenerator)
| SFC64(seed=None)
|
| BitGenerator for Chris Doty-Humphrey's Small Fast Chaotic PRNG.
|
| Parameters
| ----------
| seed : {None, int, array_like[ints], SeedSequence}, optional
| A seed to initialize the `BitGenerator`. If None, then fresh,
| unpredictable entropy will be pulled from the OS. If an ``int`` or
| ``array_like[ints]`` is passed, then it will be passed to
| `SeedSequence` to derive the initial `BitGenerator` state. One may also
| pass in a `SeedSequence` instance.
|
| Notes
| -----
| ``SFC64`` is a 256-bit implementation of Chris Doty-Humphrey's Small Fast
| Chaotic PRNG ([1]_). ``SFC64`` has a few different cycles that one might be
| on, depending on the seed; the expected period will be about
| :math:`2^{255}` ([2]_). ``SFC64`` incorporates a 64-bit counter which means
| that the absolute minimum cycle length is :math:`2^{64}` and that distinct
| seeds will not run into each other for at least :math:`2^{64}` iterations.
|
| ``SFC64`` provides a capsule containing function pointers that produce
| doubles, and unsigned 32 and 64- bit integers. These are not
| directly consumable in Python and must be consumed by a ``Generator``
| or similar object that supports low-level access.
|
| **State and Seeding**
|
| The ``SFC64`` state vector consists of 4 unsigned 64-bit values. The last
| is a 64-bit counter that increments by 1 each iteration.
|
| The input seed is processed by `SeedSequence` to generate the first
| 3 values, then the ``SFC64`` algorithm is iterated a small number of times
| to mix.
|
| **Compatibility Guarantee**
|
| ``SFC64`` makes a guarantee that a fixed seed will always produce the same
| random integer stream.
|
| References
| ----------
| .. [1] `"PractRand"
| <http://pracrand.sourceforge.net/RNG_engines.txt>`_
| .. [2] `"Random Invertible Mapping Statistics"
| <http://www.pcg-random.org/posts/random-invertible-mapping-statistics.html>`_
|
| Method resolution order:
| SFC64
| numpy.random.bit_generator.BitGenerator
| builtins.object
|
| Methods defined here:
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce_cython__(...)
|
| __setstate_cython__(...)
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| state
| Get or set the PRNG state
|
| Returns
| -------
| state : dict
| Dictionary containing the information required to describe the
| state of the PRNG
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| __pyx_vtable__ = <capsule object NULL>
|
| ----------------------------------------------------------------------
| Methods inherited from numpy.random.bit_generator.BitGenerator:
|
| __getstate__(...)
| Helper for pickle.
|
| __reduce__(...)
| Helper for pickle.
|
| __setstate__(...)
|
| random_raw(...)
| random_raw(self, size=None)
|
| Return randoms as generated by the underlying BitGenerator
|
| Parameters
| ----------
| size : int or tuple of ints, optional
| Output shape. If the given shape is, e.g., ``(m, n, k)``, then
| ``m * n * k`` samples are drawn. Default is None, in which case a
| single value is returned.
| output : bool, optional
| Output values. Used for performance testing since the generated
| values are not returned.
|
| Returns
| -------
| out : uint or ndarray
| Drawn samples.
|
| Notes
| -----
| This method directly exposes the raw underlying pseudo-random
| number generator. All values are returned as unsigned 64-bit
| values irrespective of the number of bits produced by the PRNG.
|
| See the class docstring for the number of bits returned.
|
| spawn(...)
| spawn(n_children)
|
| Create new independent child bit generators.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children. Some bit generators also implement ``jumped``
| as a different approach for creating independent streams.
|
| .. versionadded:: 1.25.0
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| child_bit_generators : list of BitGenerators
|
| Raises
| ------
| TypeError
| When the underlying SeedSequence does not implement spawning.
|
| See Also
| --------
| random.Generator.spawn, random.SeedSequence.spawn :
| Equivalent method on the generator and seed sequence.
|
| ----------------------------------------------------------------------
| Data descriptors inherited from numpy.random.bit_generator.BitGenerator:
|
| capsule
|
| cffi
| CFFI interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing CFFI wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| ctypes
| ctypes interface
|
| Returns
| -------
| interface : namedtuple
| Named tuple containing ctypes wrapper
|
| * state_address - Memory address of the state struct
| * state - pointer to the state struct
| * next_uint64 - function pointer to produce 64 bit integers
| * next_uint32 - function pointer to produce 32 bit integers
| * next_double - function pointer to produce doubles
| * bitgen - pointer to the bit generator struct
|
| lock
|
| seed_seq
| Get the seed sequence used to initialize the bit generator.
|
| .. versionadded:: 1.25.0
|
| Returns
| -------
| seed_seq : ISeedSequence
| The SeedSequence object used to initialize the BitGenerator.
| This is normally a `np.random.SeedSequence` instance.
class SeedSequence(builtins.object)
| SeedSequence(entropy=None, *, spawn_key=(), pool_size=4)
|
| SeedSequence mixes sources of entropy in a reproducible way to set the
| initial state for independent and very probably non-overlapping
| BitGenerators.
|
| Once the SeedSequence is instantiated, you can call the `generate_state`
| method to get an appropriately sized seed. Calling `spawn(n) <spawn>` will
| create ``n`` SeedSequences that can be used to seed independent
| BitGenerators, i.e. for different threads.
|
| Parameters
| ----------
| entropy : {None, int, sequence[int]}, optional
| The entropy for creating a `SeedSequence`.
| All integer values must be non-negative.
| spawn_key : {(), sequence[int]}, optional
| An additional source of entropy based on the position of this
| `SeedSequence` in the tree of such objects created with the
| `SeedSequence.spawn` method. Typically, only `SeedSequence.spawn` will
| set this, and users will not.
| pool_size : {int}, optional
| Size of the pooled entropy to store. Default is 4 to give a 128-bit
| entropy pool. 8 (for 256 bits) is another reasonable choice if working
| with larger PRNGs, but there is very little to be gained by selecting
| another value.
| n_children_spawned : {int}, optional
| The number of children already spawned. Only pass this if
| reconstructing a `SeedSequence` from a serialized form.
|
| Notes
| -----
|
| Best practice for achieving reproducible bit streams is to use
| the default ``None`` for the initial entropy, and then use
| `SeedSequence.entropy` to log/pickle the `entropy` for reproducibility:
|
| >>> sq1 = np.random.SeedSequence()
| >>> sq1.entropy
| 243799254704924441050048792905230269161 # random
| >>> sq2 = np.random.SeedSequence(sq1.entropy)
| >>> np.all(sq1.generate_state(10) == sq2.generate_state(10))
| True
|
| Methods defined here:
|
| __init__(self, /, *args, **kwargs)
| Initialize self. See help(type(self)) for accurate signature.
|
| __reduce__ = __reduce_cython__(...)
|
| __repr__(...)
| Return repr(self).
|
| __setstate__ = __setstate_cython__(...)
|
| generate_state(...)
| generate_state(n_words, dtype=np.uint32)
|
| Return the requested number of words for PRNG seeding.
|
| A BitGenerator should call this method in its constructor with
| an appropriate `n_words` parameter to properly seed itself.
|
| Parameters
| ----------
| n_words : int
| dtype : np.uint32 or np.uint64, optional
| The size of each word. This should only be either `uint32` or
| `uint64`. Strings (`'uint32'`, `'uint64'`) are fine. Note that
| requesting `uint64` will draw twice as many bits as `uint32` for
| the same `n_words`. This is a convenience for `BitGenerator`s that
| express their states as `uint64` arrays.
|
| Returns
| -------
| state : uint32 or uint64 array, shape=(n_words,)
|
| spawn(...)
| spawn(n_children)
|
| Spawn a number of child `SeedSequence` s by extending the
| `spawn_key`.
|
| See :ref:`seedsequence-spawn` for additional notes on spawning
| children.
|
| Parameters
| ----------
| n_children : int
|
| Returns
| -------
| seqs : list of `SeedSequence` s
|
| See Also
| --------
| random.Generator.spawn, random.BitGenerator.spawn :
| Equivalent method on the generator and bit generator.
|
| ----------------------------------------------------------------------
| Static methods defined here:
|
| __new__(*args, **kwargs) from builtins.type
| Create and return a new object. See help(type) for accurate signature.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| entropy
|
| n_children_spawned
|
| pool
|
| pool_size
|
| spawn_key
|
| state
|
| ----------------------------------------------------------------------
| Data and other attributes defined here:
|
| __pyx_vtable__ = <capsule object NULL>
FUNCTIONS
beta(...) method of numpy.random.mtrand.RandomState instance
beta(a, b, size=None)
Draw samples from a Beta distribution.
The Beta distribution is a special case of the Dirichlet distribution,
and is related to the Gamma distribution. It has the probability
distribution function
.. math:: f(x; a,b) = \frac{1}{B(\alpha, \beta)} x^{\alpha - 1}
(1 - x)^{\beta - 1},
where the normalization, B, is the beta function,
.. math:: B(\alpha, \beta) = \int_0^1 t^{\alpha - 1}
(1 - t)^{\beta - 1} dt.
It is often seen in Bayesian inference and order statistics.
.. note::
New code should use the `~numpy.random.Generator.beta`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
a : float or array_like of floats
Alpha, positive (>0).
b : float or array_like of floats
Beta, positive (>0).
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``a`` and ``b`` are both scalars.
Otherwise, ``np.broadcast(a, b).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized beta distribution.
See Also
--------
random.Generator.beta: which should be used for new code.
binomial(...) method of numpy.random.mtrand.RandomState instance
binomial(n, p, size=None)
Draw samples from a binomial distribution.
Samples are drawn from a binomial distribution with specified
parameters, n trials and p probability of success where
n an integer >= 0 and p is in the interval [0,1]. (n may be
input as a float, but it is truncated to an integer in use)
.. note::
New code should use the `~numpy.random.Generator.binomial`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
n : int or array_like of ints
Parameter of the distribution, >= 0. Floats are also accepted,
but they will be truncated to integers.
p : float or array_like of floats
Parameter of the distribution, >= 0 and <=1.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``n`` and ``p`` are both scalars.
Otherwise, ``np.broadcast(n, p).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized binomial distribution, where
each sample is equal to the number of successes over the n trials.
See Also
--------
scipy.stats.binom : probability density function, distribution or
cumulative density function, etc.
random.Generator.binomial: which should be used for new code.
Notes
-----
The probability density for the binomial distribution is
.. math:: P(N) = \binom{n}{N}p^N(1-p)^{n-N},
where :math:`n` is the number of trials, :math:`p` is the probability
of success, and :math:`N` is the number of successes.
When estimating the standard error of a proportion in a population by
using a random sample, the normal distribution works well unless the
product p*n <=5, where p = population proportion estimate, and n =
number of samples, in which case the binomial distribution is used
instead. For example, a sample of 15 people shows 4 who are left
handed, and 11 who are right handed. Then p = 4/15 = 27%. 0.27*15 = 4,
so the binomial distribution should be used in this case.
References
----------
.. [1] Dalgaard, Peter, "Introductory Statistics with R",
Springer-Verlag, 2002.
.. [2] Glantz, Stanton A. "Primer of Biostatistics.", McGraw-Hill,
Fifth Edition, 2002.
.. [3] Lentner, Marvin, "Elementary Applied Statistics", Bogden
and Quigley, 1972.
.. [4] Weisstein, Eric W. "Binomial Distribution." From MathWorld--A
Wolfram Web Resource.
http://mathworld.wolfram.com/BinomialDistribution.html
.. [5] Wikipedia, "Binomial distribution",
https://en.wikipedia.org/wiki/Binomial_distribution
Examples
--------
Draw samples from the distribution:
>>> n, p = 10, .5 # number of trials, probability of each trial
>>> s = np.random.binomial(n, p, 1000)
# result of flipping a coin 10 times, tested 1000 times.
A real world example. A company drills 9 wild-cat oil exploration
wells, each with an estimated probability of success of 0.1. All nine
wells fail. What is the probability of that happening?
Let's do 20,000 trials of the model, and count the number that
generate zero positive results.
>>> sum(np.random.binomial(9, 0.1, 20000) == 0)/20000.
# answer = 0.38885, or 38%.
bytes(...) method of numpy.random.mtrand.RandomState instance
bytes(length)
Return random bytes.
.. note::
New code should use the `~numpy.random.Generator.bytes`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
length : int
Number of random bytes.
Returns
-------
out : bytes
String of length `length`.
See Also
--------
random.Generator.bytes: which should be used for new code.
Examples
--------
>>> np.random.bytes(10)
b' eh\x85\x022SZ\xbf\xa4' #random
chisquare(...) method of numpy.random.mtrand.RandomState instance
chisquare(df, size=None)
Draw samples from a chi-square distribution.
When `df` independent random variables, each with standard normal
distributions (mean 0, variance 1), are squared and summed, the
resulting distribution is chi-square (see Notes). This distribution
is often used in hypothesis testing.
.. note::
New code should use the `~numpy.random.Generator.chisquare`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
df : float or array_like of floats
Number of degrees of freedom, must be > 0.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``df`` is a scalar. Otherwise,
``np.array(df).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized chi-square distribution.
Raises
------
ValueError
When `df` <= 0 or when an inappropriate `size` (e.g. ``size=-1``)
is given.
See Also
--------
random.Generator.chisquare: which should be used for new code.
Notes
-----
The variable obtained by summing the squares of `df` independent,
standard normally distributed random variables:
.. math:: Q = \sum_{i=0}^{\mathtt{df}} X^2_i
is chi-square distributed, denoted
.. math:: Q \sim \chi^2_k.
The probability density function of the chi-squared distribution is
.. math:: p(x) = \frac{(1/2)^{k/2}}{\Gamma(k/2)}
x^{k/2 - 1} e^{-x/2},
where :math:`\Gamma` is the gamma function,
.. math:: \Gamma(x) = \int_0^{-\infty} t^{x - 1} e^{-t} dt.
References
----------
.. [1] NIST "Engineering Statistics Handbook"
https://www.itl.nist.gov/div898/handbook/eda/section3/eda3666.htm
Examples
--------
>>> np.random.chisquare(2,4)
array([ 1.89920014, 9.00867716, 3.13710533, 5.62318272]) # random
choice(...) method of numpy.random.mtrand.RandomState instance
choice(a, size=None, replace=True, p=None)
Generates a random sample from a given 1-D array
.. versionadded:: 1.7.0
.. note::
New code should use the `~numpy.random.Generator.choice`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
a : 1-D array-like or int
If an ndarray, a random sample is generated from its elements.
If an int, the random sample is generated as if it were ``np.arange(a)``
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
replace : boolean, optional
Whether the sample is with or without replacement. Default is True,
meaning that a value of ``a`` can be selected multiple times.
p : 1-D array-like, optional
The probabilities associated with each entry in a.
If not given, the sample assumes a uniform distribution over all
entries in ``a``.
Returns
-------
samples : single item or ndarray
The generated random samples
Raises
------
ValueError
If a is an int and less than zero, if a or p are not 1-dimensional,
if a is an array-like of size 0, if p is not a vector of
probabilities, if a and p have different lengths, or if
replace=False and the sample size is greater than the population
size
See Also
--------
randint, shuffle, permutation
random.Generator.choice: which should be used in new code
Notes
-----
Setting user-specified probabilities through ``p`` uses a more general but less
efficient sampler than the default. The general sampler produces a different sample
than the optimized sampler even if each element of ``p`` is 1 / len(a).
Sampling random rows from a 2-D array is not possible with this function,
but is possible with `Generator.choice` through its ``axis`` keyword.
Examples
--------
Generate a uniform random sample from np.arange(5) of size 3:
>>> np.random.choice(5, 3)
array([0, 3, 4]) # random
>>> #This is equivalent to np.random.randint(0,5,3)
Generate a non-uniform random sample from np.arange(5) of size 3:
>>> np.random.choice(5, 3, p=[0.1, 0, 0.3, 0.6, 0])
array([3, 3, 0]) # random
Generate a uniform random sample from np.arange(5) of size 3 without
replacement:
>>> np.random.choice(5, 3, replace=False)
array([3,1,0]) # random
>>> #This is equivalent to np.random.permutation(np.arange(5))[:3]
Generate a non-uniform random sample from np.arange(5) of size
3 without replacement:
>>> np.random.choice(5, 3, replace=False, p=[0.1, 0, 0.3, 0.6, 0])
array([2, 3, 0]) # random
Any of the above can be repeated with an arbitrary array-like
instead of just integers. For instance:
>>> aa_milne_arr = ['pooh', 'rabbit', 'piglet', 'Christopher']
>>> np.random.choice(aa_milne_arr, 5, p=[0.5, 0.1, 0.1, 0.3])
array(['pooh', 'pooh', 'pooh', 'Christopher', 'piglet'], # random
dtype='<U11')
default_rng(...)
default_rng(seed=None)
Construct a new Generator with the default BitGenerator (PCG64).
Parameters
----------
seed : {None, int, array_like[ints], SeedSequence, BitGenerator, Generator}, optional
A seed to initialize the `BitGenerator`. If None, then fresh,
unpredictable entropy will be pulled from the OS. If an ``int`` or
``array_like[ints]`` is passed, then it will be passed to
`SeedSequence` to derive the initial `BitGenerator` state. One may also
pass in a `SeedSequence` instance.
Additionally, when passed a `BitGenerator`, it will be wrapped by
`Generator`. If passed a `Generator`, it will be returned unaltered.
Returns
-------
Generator
The initialized generator object.
Notes
-----
If ``seed`` is not a `BitGenerator` or a `Generator`, a new `BitGenerator`
is instantiated. This function does not manage a default global instance.
See :ref:`seeding_and_entropy` for more information about seeding.
Examples
--------
``default_rng`` is the recommended constructor for the random number class
``Generator``. Here are several ways we can construct a random
number generator using ``default_rng`` and the ``Generator`` class.
Here we use ``default_rng`` to generate a random float:
>>> import numpy as np
>>> rng = np.random.default_rng(12345)
>>> print(rng)
Generator(PCG64)
>>> rfloat = rng.random()
>>> rfloat
0.22733602246716966
>>> type(rfloat)
<class 'float'>
Here we use ``default_rng`` to generate 3 random integers between 0
(inclusive) and 10 (exclusive):
>>> import numpy as np
>>> rng = np.random.default_rng(12345)
>>> rints = rng.integers(low=0, high=10, size=3)
>>> rints
array([6, 2, 7])
>>> type(rints[0])
<class 'numpy.int64'>
Here we specify a seed so that we have reproducible results:
>>> import numpy as np
>>> rng = np.random.default_rng(seed=42)
>>> print(rng)
Generator(PCG64)
>>> arr1 = rng.random((3, 3))
>>> arr1
array([[0.77395605, 0.43887844, 0.85859792],
[0.69736803, 0.09417735, 0.97562235],
[0.7611397 , 0.78606431, 0.12811363]])
If we exit and restart our Python interpreter, we'll see that we
generate the same random numbers again:
>>> import numpy as np
>>> rng = np.random.default_rng(seed=42)
>>> arr2 = rng.random((3, 3))
>>> arr2
array([[0.77395605, 0.43887844, 0.85859792],
[0.69736803, 0.09417735, 0.97562235],
[0.7611397 , 0.78606431, 0.12811363]])
dirichlet(...) method of numpy.random.mtrand.RandomState instance
dirichlet(alpha, size=None)
Draw samples from the Dirichlet distribution.
Draw `size` samples of dimension k from a Dirichlet distribution. A
Dirichlet-distributed random variable can be seen as a multivariate
generalization of a Beta distribution. The Dirichlet distribution
is a conjugate prior of a multinomial distribution in Bayesian
inference.
.. note::
New code should use the `~numpy.random.Generator.dirichlet`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
alpha : sequence of floats, length k
Parameter of the distribution (length ``k`` for sample of
length ``k``).
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
vector of length ``k`` is returned.
Returns
-------
samples : ndarray,
The drawn samples, of shape ``(size, k)``.
Raises
------
ValueError
If any value in ``alpha`` is less than or equal to zero
See Also
--------
random.Generator.dirichlet: which should be used for new code.
Notes
-----
The Dirichlet distribution is a distribution over vectors
:math:`x` that fulfil the conditions :math:`x_i>0` and
:math:`\sum_{i=1}^k x_i = 1`.
The probability density function :math:`p` of a
Dirichlet-distributed random vector :math:`X` is
proportional to
.. math:: p(x) \propto \prod_{i=1}^{k}{x^{\alpha_i-1}_i},
where :math:`\alpha` is a vector containing the positive
concentration parameters.
The method uses the following property for computation: let :math:`Y`
be a random vector which has components that follow a standard gamma
distribution, then :math:`X = \frac{1}{\sum_{i=1}^k{Y_i}} Y`
is Dirichlet-distributed
References
----------
.. [1] David McKay, "Information Theory, Inference and Learning
Algorithms," chapter 23,
http://www.inference.org.uk/mackay/itila/
.. [2] Wikipedia, "Dirichlet distribution",
https://en.wikipedia.org/wiki/Dirichlet_distribution
Examples
--------
Taking an example cited in Wikipedia, this distribution can be used if
one wanted to cut strings (each of initial length 1.0) into K pieces
with different lengths, where each piece had, on average, a designated
average length, but allowing some variation in the relative sizes of
the pieces.
>>> s = np.random.dirichlet((10, 5, 3), 20).transpose()
>>> import matplotlib.pyplot as plt
>>> plt.barh(range(20), s[0])
>>> plt.barh(range(20), s[1], left=s[0], color='g')
>>> plt.barh(range(20), s[2], left=s[0]+s[1], color='r')
>>> plt.title("Lengths of Strings")
exponential(...) method of numpy.random.mtrand.RandomState instance
exponential(scale=1.0, size=None)
Draw samples from an exponential distribution.
Its probability density function is
.. math:: f(x; \frac{1}{\beta}) = \frac{1}{\beta} \exp(-\frac{x}{\beta}),
for ``x > 0`` and 0 elsewhere. :math:`\beta` is the scale parameter,
which is the inverse of the rate parameter :math:`\lambda = 1/\beta`.
The rate parameter is an alternative, widely used parameterization
of the exponential distribution [3]_.
The exponential distribution is a continuous analogue of the
geometric distribution. It describes many common situations, such as
the size of raindrops measured over many rainstorms [1]_, or the time
between page requests to Wikipedia [2]_.
.. note::
New code should use the `~numpy.random.Generator.exponential`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
scale : float or array_like of floats
The scale parameter, :math:`\beta = 1/\lambda`. Must be
non-negative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``scale`` is a scalar. Otherwise,
``np.array(scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized exponential distribution.
Examples
--------
A real world example: Assume a company has 10000 customer support
agents and the average time between customer calls is 4 minutes.
>>> n = 10000
>>> time_between_calls = np.random.default_rng().exponential(scale=4, size=n)
What is the probability that a customer will call in the next
4 to 5 minutes?
>>> x = ((time_between_calls < 5).sum())/n
>>> y = ((time_between_calls < 4).sum())/n
>>> x-y
0.08 # may vary
See Also
--------
random.Generator.exponential: which should be used for new code.
References
----------
.. [1] Peyton Z. Peebles Jr., "Probability, Random Variables and
Random Signal Principles", 4th ed, 2001, p. 57.
.. [2] Wikipedia, "Poisson process",
https://en.wikipedia.org/wiki/Poisson_process
.. [3] Wikipedia, "Exponential distribution",
https://en.wikipedia.org/wiki/Exponential_distribution
f(...) method of numpy.random.mtrand.RandomState instance
f(dfnum, dfden, size=None)
Draw samples from an F distribution.
Samples are drawn from an F distribution with specified parameters,
`dfnum` (degrees of freedom in numerator) and `dfden` (degrees of
freedom in denominator), where both parameters must be greater than
zero.
The random variate of the F distribution (also known as the
Fisher distribution) is a continuous probability distribution
that arises in ANOVA tests, and is the ratio of two chi-square
variates.
.. note::
New code should use the `~numpy.random.Generator.f`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
dfnum : float or array_like of floats
Degrees of freedom in numerator, must be > 0.
dfden : float or array_like of float
Degrees of freedom in denominator, must be > 0.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``dfnum`` and ``dfden`` are both scalars.
Otherwise, ``np.broadcast(dfnum, dfden).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Fisher distribution.
See Also
--------
scipy.stats.f : probability density function, distribution or
cumulative density function, etc.
random.Generator.f: which should be used for new code.
Notes
-----
The F statistic is used to compare in-group variances to between-group
variances. Calculating the distribution depends on the sampling, and
so it is a function of the respective degrees of freedom in the
problem. The variable `dfnum` is the number of samples minus one, the
between-groups degrees of freedom, while `dfden` is the within-groups
degrees of freedom, the sum of the number of samples in each group
minus the number of groups.
References
----------
.. [1] Glantz, Stanton A. "Primer of Biostatistics.", McGraw-Hill,
Fifth Edition, 2002.
.. [2] Wikipedia, "F-distribution",
https://en.wikipedia.org/wiki/F-distribution
Examples
--------
An example from Glantz[1], pp 47-40:
Two groups, children of diabetics (25 people) and children from people
without diabetes (25 controls). Fasting blood glucose was measured,
case group had a mean value of 86.1, controls had a mean value of
82.2. Standard deviations were 2.09 and 2.49 respectively. Are these
data consistent with the null hypothesis that the parents diabetic
status does not affect their children's blood glucose levels?
Calculating the F statistic from the data gives a value of 36.01.
Draw samples from the distribution:
>>> dfnum = 1. # between group degrees of freedom
>>> dfden = 48. # within groups degrees of freedom
>>> s = np.random.f(dfnum, dfden, 1000)
The lower bound for the top 1% of the samples is :
>>> np.sort(s)[-10]
7.61988120985 # random
So there is about a 1% chance that the F statistic will exceed 7.62,
the measured value is 36, so the null hypothesis is rejected at the 1%
level.
gamma(...) method of numpy.random.mtrand.RandomState instance
gamma(shape, scale=1.0, size=None)
Draw samples from a Gamma distribution.
Samples are drawn from a Gamma distribution with specified parameters,
`shape` (sometimes designated "k") and `scale` (sometimes designated
"theta"), where both parameters are > 0.
.. note::
New code should use the `~numpy.random.Generator.gamma`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
shape : float or array_like of floats
The shape of the gamma distribution. Must be non-negative.
scale : float or array_like of floats, optional
The scale of the gamma distribution. Must be non-negative.
Default is equal to 1.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``shape`` and ``scale`` are both scalars.
Otherwise, ``np.broadcast(shape, scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized gamma distribution.
See Also
--------
scipy.stats.gamma : probability density function, distribution or
cumulative density function, etc.
random.Generator.gamma: which should be used for new code.
Notes
-----
The probability density for the Gamma distribution is
.. math:: p(x) = x^{k-1}\frac{e^{-x/\theta}}{\theta^k\Gamma(k)},
where :math:`k` is the shape and :math:`\theta` the scale,
and :math:`\Gamma` is the Gamma function.
The Gamma distribution is often used to model the times to failure of
electronic components, and arises naturally in processes for which the
waiting times between Poisson distributed events are relevant.
References
----------
.. [1] Weisstein, Eric W. "Gamma Distribution." From MathWorld--A
Wolfram Web Resource.
http://mathworld.wolfram.com/GammaDistribution.html
.. [2] Wikipedia, "Gamma distribution",
https://en.wikipedia.org/wiki/Gamma_distribution
Examples
--------
Draw samples from the distribution:
>>> shape, scale = 2., 2. # mean=4, std=2*sqrt(2)
>>> s = np.random.gamma(shape, scale, 1000)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> import scipy.special as sps # doctest: +SKIP
>>> count, bins, ignored = plt.hist(s, 50, density=True)
>>> y = bins**(shape-1)*(np.exp(-bins/scale) / # doctest: +SKIP
... (sps.gamma(shape)*scale**shape))
>>> plt.plot(bins, y, linewidth=2, color='r') # doctest: +SKIP
>>> plt.show()
geometric(...) method of numpy.random.mtrand.RandomState instance
geometric(p, size=None)
Draw samples from the geometric distribution.
Bernoulli trials are experiments with one of two outcomes:
success or failure (an example of such an experiment is flipping
a coin). The geometric distribution models the number of trials
that must be run in order to achieve success. It is therefore
supported on the positive integers, ``k = 1, 2, ...``.
The probability mass function of the geometric distribution is
.. math:: f(k) = (1 - p)^{k - 1} p
where `p` is the probability of success of an individual trial.
.. note::
New code should use the `~numpy.random.Generator.geometric`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
p : float or array_like of floats
The probability of success of an individual trial.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``p`` is a scalar. Otherwise,
``np.array(p).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized geometric distribution.
See Also
--------
random.Generator.geometric: which should be used for new code.
Examples
--------
Draw ten thousand values from the geometric distribution,
with the probability of an individual success equal to 0.35:
>>> z = np.random.geometric(p=0.35, size=10000)
How many trials succeeded after a single run?
>>> (z == 1).sum() / 10000.
0.34889999999999999 #random
get_state(...) method of numpy.random.mtrand.RandomState instance
get_state(legacy=True)
Return a tuple representing the internal state of the generator.
For more details, see `set_state`.
Parameters
----------
legacy : bool, optional
Flag indicating to return a legacy tuple state when the BitGenerator
is MT19937, instead of a dict. Raises ValueError if the underlying
bit generator is not an instance of MT19937.
Returns
-------
out : {tuple(str, ndarray of 624 uints, int, int, float), dict}
If legacy is True, the returned tuple has the following items:
1. the string 'MT19937'.
2. a 1-D array of 624 unsigned integer keys.
3. an integer ``pos``.
4. an integer ``has_gauss``.
5. a float ``cached_gaussian``.
If `legacy` is False, or the BitGenerator is not MT19937, then
state is returned as a dictionary.
See Also
--------
set_state
Notes
-----
`set_state` and `get_state` are not needed to work with any of the
random distributions in NumPy. If the internal state is manually altered,
the user should know exactly what he/she is doing.
gumbel(...) method of numpy.random.mtrand.RandomState instance
gumbel(loc=0.0, scale=1.0, size=None)
Draw samples from a Gumbel distribution.
Draw samples from a Gumbel distribution with specified location and
scale. For more information on the Gumbel distribution, see
Notes and References below.
.. note::
New code should use the `~numpy.random.Generator.gumbel`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
loc : float or array_like of floats, optional
The location of the mode of the distribution. Default is 0.
scale : float or array_like of floats, optional
The scale parameter of the distribution. Default is 1. Must be non-
negative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``loc`` and ``scale`` are both scalars.
Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Gumbel distribution.
See Also
--------
scipy.stats.gumbel_l
scipy.stats.gumbel_r
scipy.stats.genextreme
weibull
random.Generator.gumbel: which should be used for new code.
Notes
-----
The Gumbel (or Smallest Extreme Value (SEV) or the Smallest Extreme
Value Type I) distribution is one of a class of Generalized Extreme
Value (GEV) distributions used in modeling extreme value problems.
The Gumbel is a special case of the Extreme Value Type I distribution
for maximums from distributions with "exponential-like" tails.
The probability density for the Gumbel distribution is
.. math:: p(x) = \frac{e^{-(x - \mu)/ \beta}}{\beta} e^{ -e^{-(x - \mu)/
\beta}},
where :math:`\mu` is the mode, a location parameter, and
:math:`\beta` is the scale parameter.
The Gumbel (named for German mathematician Emil Julius Gumbel) was used
very early in the hydrology literature, for modeling the occurrence of
flood events. It is also used for modeling maximum wind speed and
rainfall rates. It is a "fat-tailed" distribution - the probability of
an event in the tail of the distribution is larger than if one used a
Gaussian, hence the surprisingly frequent occurrence of 100-year
floods. Floods were initially modeled as a Gaussian process, which
underestimated the frequency of extreme events.
It is one of a class of extreme value distributions, the Generalized
Extreme Value (GEV) distributions, which also includes the Weibull and
Frechet.
The function has a mean of :math:`\mu + 0.57721\beta` and a variance
of :math:`\frac{\pi^2}{6}\beta^2`.
References
----------
.. [1] Gumbel, E. J., "Statistics of Extremes,"
New York: Columbia University Press, 1958.
.. [2] Reiss, R.-D. and Thomas, M., "Statistical Analysis of Extreme
Values from Insurance, Finance, Hydrology and Other Fields,"
Basel: Birkhauser Verlag, 2001.
Examples
--------
Draw samples from the distribution:
>>> mu, beta = 0, 0.1 # location and scale
>>> s = np.random.gumbel(mu, beta, 1000)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, 30, density=True)
>>> plt.plot(bins, (1/beta)*np.exp(-(bins - mu)/beta)
... * np.exp( -np.exp( -(bins - mu) /beta) ),
... linewidth=2, color='r')
>>> plt.show()
Show how an extreme value distribution can arise from a Gaussian process
and compare to a Gaussian:
>>> means = []
>>> maxima = []
>>> for i in range(0,1000) :
... a = np.random.normal(mu, beta, 1000)
... means.append(a.mean())
... maxima.append(a.max())
>>> count, bins, ignored = plt.hist(maxima, 30, density=True)
>>> beta = np.std(maxima) * np.sqrt(6) / np.pi
>>> mu = np.mean(maxima) - 0.57721*beta
>>> plt.plot(bins, (1/beta)*np.exp(-(bins - mu)/beta)
... * np.exp(-np.exp(-(bins - mu)/beta)),
... linewidth=2, color='r')
>>> plt.plot(bins, 1/(beta * np.sqrt(2 * np.pi))
... * np.exp(-(bins - mu)**2 / (2 * beta**2)),
... linewidth=2, color='g')
>>> plt.show()
hypergeometric(...) method of numpy.random.mtrand.RandomState instance
hypergeometric(ngood, nbad, nsample, size=None)
Draw samples from a Hypergeometric distribution.
Samples are drawn from a hypergeometric distribution with specified
parameters, `ngood` (ways to make a good selection), `nbad` (ways to make
a bad selection), and `nsample` (number of items sampled, which is less
than or equal to the sum ``ngood + nbad``).
.. note::
New code should use the
`~numpy.random.Generator.hypergeometric`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
ngood : int or array_like of ints
Number of ways to make a good selection. Must be nonnegative.
nbad : int or array_like of ints
Number of ways to make a bad selection. Must be nonnegative.
nsample : int or array_like of ints
Number of items sampled. Must be at least 1 and at most
``ngood + nbad``.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if `ngood`, `nbad`, and `nsample`
are all scalars. Otherwise, ``np.broadcast(ngood, nbad, nsample).size``
samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized hypergeometric distribution. Each
sample is the number of good items within a randomly selected subset of
size `nsample` taken from a set of `ngood` good items and `nbad` bad items.
See Also
--------
scipy.stats.hypergeom : probability density function, distribution or
cumulative density function, etc.
random.Generator.hypergeometric: which should be used for new code.
Notes
-----
The probability density for the Hypergeometric distribution is
.. math:: P(x) = \frac{\binom{g}{x}\binom{b}{n-x}}{\binom{g+b}{n}},
where :math:`0 \le x \le n` and :math:`n-b \le x \le g`
for P(x) the probability of ``x`` good results in the drawn sample,
g = `ngood`, b = `nbad`, and n = `nsample`.
Consider an urn with black and white marbles in it, `ngood` of them
are black and `nbad` are white. If you draw `nsample` balls without
replacement, then the hypergeometric distribution describes the
distribution of black balls in the drawn sample.
Note that this distribution is very similar to the binomial
distribution, except that in this case, samples are drawn without
replacement, whereas in the Binomial case samples are drawn with
replacement (or the sample space is infinite). As the sample space
becomes large, this distribution approaches the binomial.
References
----------
.. [1] Lentner, Marvin, "Elementary Applied Statistics", Bogden
and Quigley, 1972.
.. [2] Weisstein, Eric W. "Hypergeometric Distribution." From
MathWorld--A Wolfram Web Resource.
http://mathworld.wolfram.com/HypergeometricDistribution.html
.. [3] Wikipedia, "Hypergeometric distribution",
https://en.wikipedia.org/wiki/Hypergeometric_distribution
Examples
--------
Draw samples from the distribution:
>>> ngood, nbad, nsamp = 100, 2, 10
# number of good, number of bad, and number of samples
>>> s = np.random.hypergeometric(ngood, nbad, nsamp, 1000)
>>> from matplotlib.pyplot import hist
>>> hist(s)
# note that it is very unlikely to grab both bad items
Suppose you have an urn with 15 white and 15 black marbles.
If you pull 15 marbles at random, how likely is it that
12 or more of them are one color?
>>> s = np.random.hypergeometric(15, 15, 15, 100000)
>>> sum(s>=12)/100000. + sum(s<=3)/100000.
# answer = 0.003 ... pretty unlikely!
laplace(...) method of numpy.random.mtrand.RandomState instance
laplace(loc=0.0, scale=1.0, size=None)
Draw samples from the Laplace or double exponential distribution with
specified location (or mean) and scale (decay).
The Laplace distribution is similar to the Gaussian/normal distribution,
but is sharper at the peak and has fatter tails. It represents the
difference between two independent, identically distributed exponential
random variables.
.. note::
New code should use the `~numpy.random.Generator.laplace`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
loc : float or array_like of floats, optional
The position, :math:`\mu`, of the distribution peak. Default is 0.
scale : float or array_like of floats, optional
:math:`\lambda`, the exponential decay. Default is 1. Must be non-
negative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``loc`` and ``scale`` are both scalars.
Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Laplace distribution.
See Also
--------
random.Generator.laplace: which should be used for new code.
Notes
-----
It has the probability density function
.. math:: f(x; \mu, \lambda) = \frac{1}{2\lambda}
\exp\left(-\frac{|x - \mu|}{\lambda}\right).
The first law of Laplace, from 1774, states that the frequency
of an error can be expressed as an exponential function of the
absolute magnitude of the error, which leads to the Laplace
distribution. For many problems in economics and health
sciences, this distribution seems to model the data better
than the standard Gaussian distribution.
References
----------
.. [1] Abramowitz, M. and Stegun, I. A. (Eds.). "Handbook of
Mathematical Functions with Formulas, Graphs, and Mathematical
Tables, 9th printing," New York: Dover, 1972.
.. [2] Kotz, Samuel, et. al. "The Laplace Distribution and
Generalizations, " Birkhauser, 2001.
.. [3] Weisstein, Eric W. "Laplace Distribution."
From MathWorld--A Wolfram Web Resource.
http://mathworld.wolfram.com/LaplaceDistribution.html
.. [4] Wikipedia, "Laplace distribution",
https://en.wikipedia.org/wiki/Laplace_distribution
Examples
--------
Draw samples from the distribution
>>> loc, scale = 0., 1.
>>> s = np.random.laplace(loc, scale, 1000)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, 30, density=True)
>>> x = np.arange(-8., 8., .01)
>>> pdf = np.exp(-abs(x-loc)/scale)/(2.*scale)
>>> plt.plot(x, pdf)
Plot Gaussian for comparison:
>>> g = (1/(scale * np.sqrt(2 * np.pi)) *
... np.exp(-(x - loc)**2 / (2 * scale**2)))
>>> plt.plot(x,g)
logistic(...) method of numpy.random.mtrand.RandomState instance
logistic(loc=0.0, scale=1.0, size=None)
Draw samples from a logistic distribution.
Samples are drawn from a logistic distribution with specified
parameters, loc (location or mean, also median), and scale (>0).
.. note::
New code should use the `~numpy.random.Generator.logistic`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
loc : float or array_like of floats, optional
Parameter of the distribution. Default is 0.
scale : float or array_like of floats, optional
Parameter of the distribution. Must be non-negative.
Default is 1.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``loc`` and ``scale`` are both scalars.
Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized logistic distribution.
See Also
--------
scipy.stats.logistic : probability density function, distribution or
cumulative density function, etc.
random.Generator.logistic: which should be used for new code.
Notes
-----
The probability density for the Logistic distribution is
.. math:: P(x) = P(x) = \frac{e^{-(x-\mu)/s}}{s(1+e^{-(x-\mu)/s})^2},
where :math:`\mu` = location and :math:`s` = scale.
The Logistic distribution is used in Extreme Value problems where it
can act as a mixture of Gumbel distributions, in Epidemiology, and by
the World Chess Federation (FIDE) where it is used in the Elo ranking
system, assuming the performance of each player is a logistically
distributed random variable.
References
----------
.. [1] Reiss, R.-D. and Thomas M. (2001), "Statistical Analysis of
Extreme Values, from Insurance, Finance, Hydrology and Other
Fields," Birkhauser Verlag, Basel, pp 132-133.
.. [2] Weisstein, Eric W. "Logistic Distribution." From
MathWorld--A Wolfram Web Resource.
http://mathworld.wolfram.com/LogisticDistribution.html
.. [3] Wikipedia, "Logistic-distribution",
https://en.wikipedia.org/wiki/Logistic_distribution
Examples
--------
Draw samples from the distribution:
>>> loc, scale = 10, 1
>>> s = np.random.logistic(loc, scale, 10000)
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, bins=50)
# plot against distribution
>>> def logist(x, loc, scale):
... return np.exp((loc-x)/scale)/(scale*(1+np.exp((loc-x)/scale))**2)
>>> lgst_val = logist(bins, loc, scale)
>>> plt.plot(bins, lgst_val * count.max() / lgst_val.max())
>>> plt.show()
lognormal(...) method of numpy.random.mtrand.RandomState instance
lognormal(mean=0.0, sigma=1.0, size=None)
Draw samples from a log-normal distribution.
Draw samples from a log-normal distribution with specified mean,
standard deviation, and array shape. Note that the mean and standard
deviation are not the values for the distribution itself, but of the
underlying normal distribution it is derived from.
.. note::
New code should use the `~numpy.random.Generator.lognormal`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
mean : float or array_like of floats, optional
Mean value of the underlying normal distribution. Default is 0.
sigma : float or array_like of floats, optional
Standard deviation of the underlying normal distribution. Must be
non-negative. Default is 1.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``mean`` and ``sigma`` are both scalars.
Otherwise, ``np.broadcast(mean, sigma).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized log-normal distribution.
See Also
--------
scipy.stats.lognorm : probability density function, distribution,
cumulative density function, etc.
random.Generator.lognormal: which should be used for new code.
Notes
-----
A variable `x` has a log-normal distribution if `log(x)` is normally
distributed. The probability density function for the log-normal
distribution is:
.. math:: p(x) = \frac{1}{\sigma x \sqrt{2\pi}}
e^{(-\frac{(ln(x)-\mu)^2}{2\sigma^2})}
where :math:`\mu` is the mean and :math:`\sigma` is the standard
deviation of the normally distributed logarithm of the variable.
A log-normal distribution results if a random variable is the *product*
of a large number of independent, identically-distributed variables in
the same way that a normal distribution results if the variable is the
*sum* of a large number of independent, identically-distributed
variables.
References
----------
.. [1] Limpert, E., Stahel, W. A., and Abbt, M., "Log-normal
Distributions across the Sciences: Keys and Clues,"
BioScience, Vol. 51, No. 5, May, 2001.
https://stat.ethz.ch/~stahel/lognormal/bioscience.pdf
.. [2] Reiss, R.D. and Thomas, M., "Statistical Analysis of Extreme
Values," Basel: Birkhauser Verlag, 2001, pp. 31-32.
Examples
--------
Draw samples from the distribution:
>>> mu, sigma = 3., 1. # mean and standard deviation
>>> s = np.random.lognormal(mu, sigma, 1000)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, 100, density=True, align='mid')
>>> x = np.linspace(min(bins), max(bins), 10000)
>>> pdf = (np.exp(-(np.log(x) - mu)**2 / (2 * sigma**2))
... / (x * sigma * np.sqrt(2 * np.pi)))
>>> plt.plot(x, pdf, linewidth=2, color='r')
>>> plt.axis('tight')
>>> plt.show()
Demonstrate that taking the products of random samples from a uniform
distribution can be fit well by a log-normal probability density
function.
>>> # Generate a thousand samples: each is the product of 100 random
>>> # values, drawn from a normal distribution.
>>> b = []
>>> for i in range(1000):
... a = 10. + np.random.standard_normal(100)
... b.append(np.prod(a))
>>> b = np.array(b) / np.min(b) # scale values to be positive
>>> count, bins, ignored = plt.hist(b, 100, density=True, align='mid')
>>> sigma = np.std(np.log(b))
>>> mu = np.mean(np.log(b))
>>> x = np.linspace(min(bins), max(bins), 10000)
>>> pdf = (np.exp(-(np.log(x) - mu)**2 / (2 * sigma**2))
... / (x * sigma * np.sqrt(2 * np.pi)))
>>> plt.plot(x, pdf, color='r', linewidth=2)
>>> plt.show()
logseries(...) method of numpy.random.mtrand.RandomState instance
logseries(p, size=None)
Draw samples from a logarithmic series distribution.
Samples are drawn from a log series distribution with specified
shape parameter, 0 <= ``p`` < 1.
.. note::
New code should use the `~numpy.random.Generator.logseries`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
p : float or array_like of floats
Shape parameter for the distribution. Must be in the range [0, 1).
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``p`` is a scalar. Otherwise,
``np.array(p).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized logarithmic series distribution.
See Also
--------
scipy.stats.logser : probability density function, distribution or
cumulative density function, etc.
random.Generator.logseries: which should be used for new code.
Notes
-----
The probability density for the Log Series distribution is
.. math:: P(k) = \frac{-p^k}{k \ln(1-p)},
where p = probability.
The log series distribution is frequently used to represent species
richness and occurrence, first proposed by Fisher, Corbet, and
Williams in 1943 [2]. It may also be used to model the numbers of
occupants seen in cars [3].
References
----------
.. [1] Buzas, Martin A.; Culver, Stephen J., Understanding regional
species diversity through the log series distribution of
occurrences: BIODIVERSITY RESEARCH Diversity & Distributions,
Volume 5, Number 5, September 1999 , pp. 187-195(9).
.. [2] Fisher, R.A,, A.S. Corbet, and C.B. Williams. 1943. The
relation between the number of species and the number of
individuals in a random sample of an animal population.
Journal of Animal Ecology, 12:42-58.
.. [3] D. J. Hand, F. Daly, D. Lunn, E. Ostrowski, A Handbook of Small
Data Sets, CRC Press, 1994.
.. [4] Wikipedia, "Logarithmic distribution",
https://en.wikipedia.org/wiki/Logarithmic_distribution
Examples
--------
Draw samples from the distribution:
>>> a = .6
>>> s = np.random.logseries(a, 10000)
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s)
# plot against distribution
>>> def logseries(k, p):
... return -p**k/(k*np.log(1-p))
>>> plt.plot(bins, logseries(bins, a)*count.max()/
... logseries(bins, a).max(), 'r')
>>> plt.show()
multinomial(...) method of numpy.random.mtrand.RandomState instance
multinomial(n, pvals, size=None)
Draw samples from a multinomial distribution.
The multinomial distribution is a multivariate generalization of the
binomial distribution. Take an experiment with one of ``p``
possible outcomes. An example of such an experiment is throwing a dice,
where the outcome can be 1 through 6. Each sample drawn from the
distribution represents `n` such experiments. Its values,
``X_i = [X_0, X_1, ..., X_p]``, represent the number of times the
outcome was ``i``.
.. note::
New code should use the `~numpy.random.Generator.multinomial`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
n : int
Number of experiments.
pvals : sequence of floats, length p
Probabilities of each of the ``p`` different outcomes. These
must sum to 1 (however, the last element is always assumed to
account for the remaining probability, as long as
``sum(pvals[:-1]) <= 1)``.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
Returns
-------
out : ndarray
The drawn samples, of shape *size*, if that was provided. If not,
the shape is ``(N,)``.
In other words, each entry ``out[i,j,...,:]`` is an N-dimensional
value drawn from the distribution.
See Also
--------
random.Generator.multinomial: which should be used for new code.
Examples
--------
Throw a dice 20 times:
>>> np.random.multinomial(20, [1/6.]*6, size=1)
array([[4, 1, 7, 5, 2, 1]]) # random
It landed 4 times on 1, once on 2, etc.
Now, throw the dice 20 times, and 20 times again:
>>> np.random.multinomial(20, [1/6.]*6, size=2)
array([[3, 4, 3, 3, 4, 3], # random
[2, 4, 3, 4, 0, 7]])
For the first run, we threw 3 times 1, 4 times 2, etc. For the second,
we threw 2 times 1, 4 times 2, etc.
A loaded die is more likely to land on number 6:
>>> np.random.multinomial(100, [1/7.]*5 + [2/7.])
array([11, 16, 14, 17, 16, 26]) # random
The probability inputs should be normalized. As an implementation
detail, the value of the last entry is ignored and assumed to take
up any leftover probability mass, but this should not be relied on.
A biased coin which has twice as much weight on one side as on the
other should be sampled like so:
>>> np.random.multinomial(100, [1.0 / 3, 2.0 / 3]) # RIGHT
array([38, 62]) # random
not like:
>>> np.random.multinomial(100, [1.0, 2.0]) # WRONG
Traceback (most recent call last):
ValueError: pvals < 0, pvals > 1 or pvals contains NaNs
multivariate_normal(...) method of numpy.random.mtrand.RandomState instance
multivariate_normal(mean, cov, size=None, check_valid='warn', tol=1e-8)
Draw random samples from a multivariate normal distribution.
The multivariate normal, multinormal or Gaussian distribution is a
generalization of the one-dimensional normal distribution to higher
dimensions. Such a distribution is specified by its mean and
covariance matrix. These parameters are analogous to the mean
(average or "center") and variance (standard deviation, or "width,"
squared) of the one-dimensional normal distribution.
.. note::
New code should use the
`~numpy.random.Generator.multivariate_normal`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
mean : 1-D array_like, of length N
Mean of the N-dimensional distribution.
cov : 2-D array_like, of shape (N, N)
Covariance matrix of the distribution. It must be symmetric and
positive-semidefinite for proper sampling.
size : int or tuple of ints, optional
Given a shape of, for example, ``(m,n,k)``, ``m*n*k`` samples are
generated, and packed in an `m`-by-`n`-by-`k` arrangement. Because
each sample is `N`-dimensional, the output shape is ``(m,n,k,N)``.
If no shape is specified, a single (`N`-D) sample is returned.
check_valid : { 'warn', 'raise', 'ignore' }, optional
Behavior when the covariance matrix is not positive semidefinite.
tol : float, optional
Tolerance when checking the singular values in covariance matrix.
cov is cast to double before the check.
Returns
-------
out : ndarray
The drawn samples, of shape *size*, if that was provided. If not,
the shape is ``(N,)``.
In other words, each entry ``out[i,j,...,:]`` is an N-dimensional
value drawn from the distribution.
See Also
--------
random.Generator.multivariate_normal: which should be used for new code.
Notes
-----
The mean is a coordinate in N-dimensional space, which represents the
location where samples are most likely to be generated. This is
analogous to the peak of the bell curve for the one-dimensional or
univariate normal distribution.
Covariance indicates the level to which two variables vary together.
From the multivariate normal distribution, we draw N-dimensional
samples, :math:`X = [x_1, x_2, ... x_N]`. The covariance matrix
element :math:`C_{ij}` is the covariance of :math:`x_i` and :math:`x_j`.
The element :math:`C_{ii}` is the variance of :math:`x_i` (i.e. its
"spread").
Instead of specifying the full covariance matrix, popular
approximations include:
- Spherical covariance (`cov` is a multiple of the identity matrix)
- Diagonal covariance (`cov` has non-negative elements, and only on
the diagonal)
This geometrical property can be seen in two dimensions by plotting
generated data-points:
>>> mean = [0, 0]
>>> cov = [[1, 0], [0, 100]] # diagonal covariance
Diagonal covariance means that points are oriented along x or y-axis:
>>> import matplotlib.pyplot as plt
>>> x, y = np.random.multivariate_normal(mean, cov, 5000).T
>>> plt.plot(x, y, 'x')
>>> plt.axis('equal')
>>> plt.show()
Note that the covariance matrix must be positive semidefinite (a.k.a.
nonnegative-definite). Otherwise, the behavior of this method is
undefined and backwards compatibility is not guaranteed.
References
----------
.. [1] Papoulis, A., "Probability, Random Variables, and Stochastic
Processes," 3rd ed., New York: McGraw-Hill, 1991.
.. [2] Duda, R. O., Hart, P. E., and Stork, D. G., "Pattern
Classification," 2nd ed., New York: Wiley, 2001.
Examples
--------
>>> mean = (1, 2)
>>> cov = [[1, 0], [0, 1]]
>>> x = np.random.multivariate_normal(mean, cov, (3, 3))
>>> x.shape
(3, 3, 2)
Here we generate 800 samples from the bivariate normal distribution
with mean [0, 0] and covariance matrix [[6, -3], [-3, 3.5]]. The
expected variances of the first and second components of the sample
are 6 and 3.5, respectively, and the expected correlation
coefficient is -3/sqrt(6*3.5) ≈ -0.65465.
>>> cov = np.array([[6, -3], [-3, 3.5]])
>>> pts = np.random.multivariate_normal([0, 0], cov, size=800)
Check that the mean, covariance, and correlation coefficient of the
sample are close to the expected values:
>>> pts.mean(axis=0)
array([ 0.0326911 , -0.01280782]) # may vary
>>> np.cov(pts.T)
array([[ 5.96202397, -2.85602287],
[-2.85602287, 3.47613949]]) # may vary
>>> np.corrcoef(pts.T)[0, 1]
-0.6273591314603949 # may vary
We can visualize this data with a scatter plot. The orientation
of the point cloud illustrates the negative correlation of the
components of this sample.
>>> import matplotlib.pyplot as plt
>>> plt.plot(pts[:, 0], pts[:, 1], '.', alpha=0.5)
>>> plt.axis('equal')
>>> plt.grid()
>>> plt.show()
negative_binomial(...) method of numpy.random.mtrand.RandomState instance
negative_binomial(n, p, size=None)
Draw samples from a negative binomial distribution.
Samples are drawn from a negative binomial distribution with specified
parameters, `n` successes and `p` probability of success where `n`
is > 0 and `p` is in the interval [0, 1].
.. note::
New code should use the
`~numpy.random.Generator.negative_binomial`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
n : float or array_like of floats
Parameter of the distribution, > 0.
p : float or array_like of floats
Parameter of the distribution, >= 0 and <=1.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``n`` and ``p`` are both scalars.
Otherwise, ``np.broadcast(n, p).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized negative binomial distribution,
where each sample is equal to N, the number of failures that
occurred before a total of n successes was reached.
See Also
--------
random.Generator.negative_binomial: which should be used for new code.
Notes
-----
The probability mass function of the negative binomial distribution is
.. math:: P(N;n,p) = \frac{\Gamma(N+n)}{N!\Gamma(n)}p^{n}(1-p)^{N},
where :math:`n` is the number of successes, :math:`p` is the
probability of success, :math:`N+n` is the number of trials, and
:math:`\Gamma` is the gamma function. When :math:`n` is an integer,
:math:`\frac{\Gamma(N+n)}{N!\Gamma(n)} = \binom{N+n-1}{N}`, which is
the more common form of this term in the pmf. The negative
binomial distribution gives the probability of N failures given n
successes, with a success on the last trial.
If one throws a die repeatedly until the third time a "1" appears,
then the probability distribution of the number of non-"1"s that
appear before the third "1" is a negative binomial distribution.
References
----------
.. [1] Weisstein, Eric W. "Negative Binomial Distribution." From
MathWorld--A Wolfram Web Resource.
http://mathworld.wolfram.com/NegativeBinomialDistribution.html
.. [2] Wikipedia, "Negative binomial distribution",
https://en.wikipedia.org/wiki/Negative_binomial_distribution
Examples
--------
Draw samples from the distribution:
A real world example. A company drills wild-cat oil
exploration wells, each with an estimated probability of
success of 0.1. What is the probability of having one success
for each successive well, that is what is the probability of a
single success after drilling 5 wells, after 6 wells, etc.?
>>> s = np.random.negative_binomial(1, 0.1, 100000)
>>> for i in range(1, 11): # doctest: +SKIP
... probability = sum(s<i) / 100000.
... print(i, "wells drilled, probability of one success =", probability)
noncentral_chisquare(...) method of numpy.random.mtrand.RandomState instance
noncentral_chisquare(df, nonc, size=None)
Draw samples from a noncentral chi-square distribution.
The noncentral :math:`\chi^2` distribution is a generalization of
the :math:`\chi^2` distribution.
.. note::
New code should use the
`~numpy.random.Generator.noncentral_chisquare`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
df : float or array_like of floats
Degrees of freedom, must be > 0.
.. versionchanged:: 1.10.0
Earlier NumPy versions required dfnum > 1.
nonc : float or array_like of floats
Non-centrality, must be non-negative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``df`` and ``nonc`` are both scalars.
Otherwise, ``np.broadcast(df, nonc).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized noncentral chi-square distribution.
See Also
--------
random.Generator.noncentral_chisquare: which should be used for new code.
Notes
-----
The probability density function for the noncentral Chi-square
distribution is
.. math:: P(x;df,nonc) = \sum^{\infty}_{i=0}
\frac{e^{-nonc/2}(nonc/2)^{i}}{i!}
P_{Y_{df+2i}}(x),
where :math:`Y_{q}` is the Chi-square with q degrees of freedom.
References
----------
.. [1] Wikipedia, "Noncentral chi-squared distribution"
https://en.wikipedia.org/wiki/Noncentral_chi-squared_distribution
Examples
--------
Draw values from the distribution and plot the histogram
>>> import matplotlib.pyplot as plt
>>> values = plt.hist(np.random.noncentral_chisquare(3, 20, 100000),
... bins=200, density=True)
>>> plt.show()
Draw values from a noncentral chisquare with very small noncentrality,
and compare to a chisquare.
>>> plt.figure()
>>> values = plt.hist(np.random.noncentral_chisquare(3, .0000001, 100000),
... bins=np.arange(0., 25, .1), density=True)
>>> values2 = plt.hist(np.random.chisquare(3, 100000),
... bins=np.arange(0., 25, .1), density=True)
>>> plt.plot(values[1][0:-1], values[0]-values2[0], 'ob')
>>> plt.show()
Demonstrate how large values of non-centrality lead to a more symmetric
distribution.
>>> plt.figure()
>>> values = plt.hist(np.random.noncentral_chisquare(3, 20, 100000),
... bins=200, density=True)
>>> plt.show()
noncentral_f(...) method of numpy.random.mtrand.RandomState instance
noncentral_f(dfnum, dfden, nonc, size=None)
Draw samples from the noncentral F distribution.
Samples are drawn from an F distribution with specified parameters,
`dfnum` (degrees of freedom in numerator) and `dfden` (degrees of
freedom in denominator), where both parameters > 1.
`nonc` is the non-centrality parameter.
.. note::
New code should use the
`~numpy.random.Generator.noncentral_f`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
dfnum : float or array_like of floats
Numerator degrees of freedom, must be > 0.
.. versionchanged:: 1.14.0
Earlier NumPy versions required dfnum > 1.
dfden : float or array_like of floats
Denominator degrees of freedom, must be > 0.
nonc : float or array_like of floats
Non-centrality parameter, the sum of the squares of the numerator
means, must be >= 0.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``dfnum``, ``dfden``, and ``nonc``
are all scalars. Otherwise, ``np.broadcast(dfnum, dfden, nonc).size``
samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized noncentral Fisher distribution.
See Also
--------
random.Generator.noncentral_f: which should be used for new code.
Notes
-----
When calculating the power of an experiment (power = probability of
rejecting the null hypothesis when a specific alternative is true) the
non-central F statistic becomes important. When the null hypothesis is
true, the F statistic follows a central F distribution. When the null
hypothesis is not true, then it follows a non-central F statistic.
References
----------
.. [1] Weisstein, Eric W. "Noncentral F-Distribution."
From MathWorld--A Wolfram Web Resource.
http://mathworld.wolfram.com/NoncentralF-Distribution.html
.. [2] Wikipedia, "Noncentral F-distribution",
https://en.wikipedia.org/wiki/Noncentral_F-distribution
Examples
--------
In a study, testing for a specific alternative to the null hypothesis
requires use of the Noncentral F distribution. We need to calculate the
area in the tail of the distribution that exceeds the value of the F
distribution for the null hypothesis. We'll plot the two probability
distributions for comparison.
>>> dfnum = 3 # between group deg of freedom
>>> dfden = 20 # within groups degrees of freedom
>>> nonc = 3.0
>>> nc_vals = np.random.noncentral_f(dfnum, dfden, nonc, 1000000)
>>> NF = np.histogram(nc_vals, bins=50, density=True)
>>> c_vals = np.random.f(dfnum, dfden, 1000000)
>>> F = np.histogram(c_vals, bins=50, density=True)
>>> import matplotlib.pyplot as plt
>>> plt.plot(F[1][1:], F[0])
>>> plt.plot(NF[1][1:], NF[0])
>>> plt.show()
normal(...) method of numpy.random.mtrand.RandomState instance
normal(loc=0.0, scale=1.0, size=None)
Draw random samples from a normal (Gaussian) distribution.
The probability density function of the normal distribution, first
derived by De Moivre and 200 years later by both Gauss and Laplace
independently [2]_, is often called the bell curve because of
its characteristic shape (see the example below).
The normal distributions occurs often in nature. For example, it
describes the commonly occurring distribution of samples influenced
by a large number of tiny, random disturbances, each with its own
unique distribution [2]_.
.. note::
New code should use the `~numpy.random.Generator.normal`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
loc : float or array_like of floats
Mean ("centre") of the distribution.
scale : float or array_like of floats
Standard deviation (spread or "width") of the distribution. Must be
non-negative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``loc`` and ``scale`` are both scalars.
Otherwise, ``np.broadcast(loc, scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized normal distribution.
See Also
--------
scipy.stats.norm : probability density function, distribution or
cumulative density function, etc.
random.Generator.normal: which should be used for new code.
Notes
-----
The probability density for the Gaussian distribution is
.. math:: p(x) = \frac{1}{\sqrt{ 2 \pi \sigma^2 }}
e^{ - \frac{ (x - \mu)^2 } {2 \sigma^2} },
where :math:`\mu` is the mean and :math:`\sigma` the standard
deviation. The square of the standard deviation, :math:`\sigma^2`,
is called the variance.
The function has its peak at the mean, and its "spread" increases with
the standard deviation (the function reaches 0.607 times its maximum at
:math:`x + \sigma` and :math:`x - \sigma` [2]_). This implies that
normal is more likely to return samples lying close to the mean, rather
than those far away.
References
----------
.. [1] Wikipedia, "Normal distribution",
https://en.wikipedia.org/wiki/Normal_distribution
.. [2] P. R. Peebles Jr., "Central Limit Theorem" in "Probability,
Random Variables and Random Signal Principles", 4th ed., 2001,
pp. 51, 51, 125.
Examples
--------
Draw samples from the distribution:
>>> mu, sigma = 0, 0.1 # mean and standard deviation
>>> s = np.random.normal(mu, sigma, 1000)
Verify the mean and the variance:
>>> abs(mu - np.mean(s))
0.0 # may vary
>>> abs(sigma - np.std(s, ddof=1))
0.1 # may vary
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, 30, density=True)
>>> plt.plot(bins, 1/(sigma * np.sqrt(2 * np.pi)) *
... np.exp( - (bins - mu)**2 / (2 * sigma**2) ),
... linewidth=2, color='r')
>>> plt.show()
Two-by-four array of samples from the normal distribution with
mean 3 and standard deviation 2.5:
>>> np.random.normal(3, 2.5, size=(2, 4))
array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
[ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
pareto(...) method of numpy.random.mtrand.RandomState instance
pareto(a, size=None)
Draw samples from a Pareto II or Lomax distribution with
specified shape.
The Lomax or Pareto II distribution is a shifted Pareto
distribution. The classical Pareto distribution can be
obtained from the Lomax distribution by adding 1 and
multiplying by the scale parameter ``m`` (see Notes). The
smallest value of the Lomax distribution is zero while for the
classical Pareto distribution it is ``mu``, where the standard
Pareto distribution has location ``mu = 1``. Lomax can also
be considered as a simplified version of the Generalized
Pareto distribution (available in SciPy), with the scale set
to one and the location set to zero.
The Pareto distribution must be greater than zero, and is
unbounded above. It is also known as the "80-20 rule". In
this distribution, 80 percent of the weights are in the lowest
20 percent of the range, while the other 20 percent fill the
remaining 80 percent of the range.
.. note::
New code should use the `~numpy.random.Generator.pareto`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
a : float or array_like of floats
Shape of the distribution. Must be positive.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``a`` is a scalar. Otherwise,
``np.array(a).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Pareto distribution.
See Also
--------
scipy.stats.lomax : probability density function, distribution or
cumulative density function, etc.
scipy.stats.genpareto : probability density function, distribution or
cumulative density function, etc.
random.Generator.pareto: which should be used for new code.
Notes
-----
The probability density for the Pareto distribution is
.. math:: p(x) = \frac{am^a}{x^{a+1}}
where :math:`a` is the shape and :math:`m` the scale.
The Pareto distribution, named after the Italian economist
Vilfredo Pareto, is a power law probability distribution
useful in many real world problems. Outside the field of
economics it is generally referred to as the Bradford
distribution. Pareto developed the distribution to describe
the distribution of wealth in an economy. It has also found
use in insurance, web page access statistics, oil field sizes,
and many other problems, including the download frequency for
projects in Sourceforge [1]_. It is one of the so-called
"fat-tailed" distributions.
References
----------
.. [1] Francis Hunt and Paul Johnson, On the Pareto Distribution of
Sourceforge projects.
.. [2] Pareto, V. (1896). Course of Political Economy. Lausanne.
.. [3] Reiss, R.D., Thomas, M.(2001), Statistical Analysis of Extreme
Values, Birkhauser Verlag, Basel, pp 23-30.
.. [4] Wikipedia, "Pareto distribution",
https://en.wikipedia.org/wiki/Pareto_distribution
Examples
--------
Draw samples from the distribution:
>>> a, m = 3., 2. # shape and mode
>>> s = (np.random.pareto(a, 1000) + 1) * m
Display the histogram of the samples, along with the probability
density function:
>>> import matplotlib.pyplot as plt
>>> count, bins, _ = plt.hist(s, 100, density=True)
>>> fit = a*m**a / bins**(a+1)
>>> plt.plot(bins, max(count)*fit/max(fit), linewidth=2, color='r')
>>> plt.show()
permutation(...) method of numpy.random.mtrand.RandomState instance
permutation(x)
Randomly permute a sequence, or return a permuted range.
If `x` is a multi-dimensional array, it is only shuffled along its
first index.
.. note::
New code should use the
`~numpy.random.Generator.permutation`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
x : int or array_like
If `x` is an integer, randomly permute ``np.arange(x)``.
If `x` is an array, make a copy and shuffle the elements
randomly.
Returns
-------
out : ndarray
Permuted sequence or array range.
See Also
--------
random.Generator.permutation: which should be used for new code.
Examples
--------
>>> np.random.permutation(10)
array([1, 7, 4, 3, 0, 9, 2, 5, 8, 6]) # random
>>> np.random.permutation([1, 4, 9, 12, 15])
array([15, 1, 9, 4, 12]) # random
>>> arr = np.arange(9).reshape((3, 3))
>>> np.random.permutation(arr)
array([[6, 7, 8], # random
[0, 1, 2],
[3, 4, 5]])
poisson(...) method of numpy.random.mtrand.RandomState instance
poisson(lam=1.0, size=None)
Draw samples from a Poisson distribution.
The Poisson distribution is the limit of the binomial distribution
for large N.
.. note::
New code should use the `~numpy.random.Generator.poisson`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
lam : float or array_like of floats
Expected number of events occurring in a fixed-time interval,
must be >= 0. A sequence must be broadcastable over the requested
size.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``lam`` is a scalar. Otherwise,
``np.array(lam).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Poisson distribution.
See Also
--------
random.Generator.poisson: which should be used for new code.
Notes
-----
The Poisson distribution
.. math:: f(k; \lambda)=\frac{\lambda^k e^{-\lambda}}{k!}
For events with an expected separation :math:`\lambda` the Poisson
distribution :math:`f(k; \lambda)` describes the probability of
:math:`k` events occurring within the observed
interval :math:`\lambda`.
Because the output is limited to the range of the C int64 type, a
ValueError is raised when `lam` is within 10 sigma of the maximum
representable value.
References
----------
.. [1] Weisstein, Eric W. "Poisson Distribution."
From MathWorld--A Wolfram Web Resource.
http://mathworld.wolfram.com/PoissonDistribution.html
.. [2] Wikipedia, "Poisson distribution",
https://en.wikipedia.org/wiki/Poisson_distribution
Examples
--------
Draw samples from the distribution:
>>> import numpy as np
>>> s = np.random.poisson(5, 10000)
Display histogram of the sample:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, 14, density=True)
>>> plt.show()
Draw each 100 values for lambda 100 and 500:
>>> s = np.random.poisson(lam=(100., 500.), size=(100, 2))
power(...) method of numpy.random.mtrand.RandomState instance
power(a, size=None)
Draws samples in [0, 1] from a power distribution with positive
exponent a - 1.
Also known as the power function distribution.
.. note::
New code should use the `~numpy.random.Generator.power`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
a : float or array_like of floats
Parameter of the distribution. Must be non-negative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``a`` is a scalar. Otherwise,
``np.array(a).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized power distribution.
Raises
------
ValueError
If a <= 0.
See Also
--------
random.Generator.power: which should be used for new code.
Notes
-----
The probability density function is
.. math:: P(x; a) = ax^{a-1}, 0 \le x \le 1, a>0.
The power function distribution is just the inverse of the Pareto
distribution. It may also be seen as a special case of the Beta
distribution.
It is used, for example, in modeling the over-reporting of insurance
claims.
References
----------
.. [1] Christian Kleiber, Samuel Kotz, "Statistical size distributions
in economics and actuarial sciences", Wiley, 2003.
.. [2] Heckert, N. A. and Filliben, James J. "NIST Handbook 148:
Dataplot Reference Manual, Volume 2: Let Subcommands and Library
Functions", National Institute of Standards and Technology
Handbook Series, June 2003.
https://www.itl.nist.gov/div898/software/dataplot/refman2/auxillar/powpdf.pdf
Examples
--------
Draw samples from the distribution:
>>> a = 5. # shape
>>> samples = 1000
>>> s = np.random.power(a, samples)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, bins=30)
>>> x = np.linspace(0, 1, 100)
>>> y = a*x**(a-1.)
>>> normed_y = samples*np.diff(bins)[0]*y
>>> plt.plot(x, normed_y)
>>> plt.show()
Compare the power function distribution to the inverse of the Pareto.
>>> from scipy import stats # doctest: +SKIP
>>> rvs = np.random.power(5, 1000000)
>>> rvsp = np.random.pareto(5, 1000000)
>>> xx = np.linspace(0,1,100)
>>> powpdf = stats.powerlaw.pdf(xx,5) # doctest: +SKIP
>>> plt.figure()
>>> plt.hist(rvs, bins=50, density=True)
>>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
>>> plt.title('np.random.power(5)')
>>> plt.figure()
>>> plt.hist(1./(1.+rvsp), bins=50, density=True)
>>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
>>> plt.title('inverse of 1 + np.random.pareto(5)')
>>> plt.figure()
>>> plt.hist(1./(1.+rvsp), bins=50, density=True)
>>> plt.plot(xx,powpdf,'r-') # doctest: +SKIP
>>> plt.title('inverse of stats.pareto(5)')
rand(...) method of numpy.random.mtrand.RandomState instance
rand(d0, d1, ..., dn)
Random values in a given shape.
.. note::
This is a convenience function for users porting code from Matlab,
and wraps `random_sample`. That function takes a
tuple to specify the size of the output, which is consistent with
other NumPy functions like `numpy.zeros` and `numpy.ones`.
Create an array of the given shape and populate it with
random samples from a uniform distribution
over ``[0, 1)``.
Parameters
----------
d0, d1, ..., dn : int, optional
The dimensions of the returned array, must be non-negative.
If no argument is given a single Python float is returned.
Returns
-------
out : ndarray, shape ``(d0, d1, ..., dn)``
Random values.
See Also
--------
random
Examples
--------
>>> np.random.rand(3,2)
array([[ 0.14022471, 0.96360618], #random
[ 0.37601032, 0.25528411], #random
[ 0.49313049, 0.94909878]]) #random
randint(...) method of numpy.random.mtrand.RandomState instance
randint(low, high=None, size=None, dtype=int)
Return random integers from `low` (inclusive) to `high` (exclusive).
Return random integers from the "discrete uniform" distribution of
the specified dtype in the "half-open" interval [`low`, `high`). If
`high` is None (the default), then results are from [0, `low`).
.. note::
New code should use the `~numpy.random.Generator.integers`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
low : int or array-like of ints
Lowest (signed) integers to be drawn from the distribution (unless
``high=None``, in which case this parameter is one above the
*highest* such integer).
high : int or array-like of ints, optional
If provided, one above the largest (signed) integer to be drawn
from the distribution (see above for behavior if ``high=None``).
If array-like, must contain integer values
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
dtype : dtype, optional
Desired dtype of the result. Byteorder must be native.
The default value is int.
.. versionadded:: 1.11.0
Returns
-------
out : int or ndarray of ints
`size`-shaped array of random integers from the appropriate
distribution, or a single such random int if `size` not provided.
See Also
--------
random_integers : similar to `randint`, only for the closed
interval [`low`, `high`], and 1 is the lowest value if `high` is
omitted.
random.Generator.integers: which should be used for new code.
Examples
--------
>>> np.random.randint(2, size=10)
array([1, 0, 0, 0, 1, 1, 0, 0, 1, 0]) # random
>>> np.random.randint(1, size=10)
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0])
Generate a 2 x 4 array of ints between 0 and 4, inclusive:
>>> np.random.randint(5, size=(2, 4))
array([[4, 0, 2, 1], # random
[3, 2, 2, 0]])
Generate a 1 x 3 array with 3 different upper bounds
>>> np.random.randint(1, [3, 5, 10])
array([2, 2, 9]) # random
Generate a 1 by 3 array with 3 different lower bounds
>>> np.random.randint([1, 5, 7], 10)
array([9, 8, 7]) # random
Generate a 2 by 4 array using broadcasting with dtype of uint8
>>> np.random.randint([1, 3, 5, 7], [[10], [20]], dtype=np.uint8)
array([[ 8, 6, 9, 7], # random
[ 1, 16, 9, 12]], dtype=uint8)
randn(...) method of numpy.random.mtrand.RandomState instance
randn(d0, d1, ..., dn)
Return a sample (or samples) from the "standard normal" distribution.
.. note::
This is a convenience function for users porting code from Matlab,
and wraps `standard_normal`. That function takes a
tuple to specify the size of the output, which is consistent with
other NumPy functions like `numpy.zeros` and `numpy.ones`.
.. note::
New code should use the
`~numpy.random.Generator.standard_normal`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
If positive int_like arguments are provided, `randn` generates an array
of shape ``(d0, d1, ..., dn)``, filled
with random floats sampled from a univariate "normal" (Gaussian)
distribution of mean 0 and variance 1. A single float randomly sampled
from the distribution is returned if no argument is provided.
Parameters
----------
d0, d1, ..., dn : int, optional
The dimensions of the returned array, must be non-negative.
If no argument is given a single Python float is returned.
Returns
-------
Z : ndarray or float
A ``(d0, d1, ..., dn)``-shaped array of floating-point samples from
the standard normal distribution, or a single such float if
no parameters were supplied.
See Also
--------
standard_normal : Similar, but takes a tuple as its argument.
normal : Also accepts mu and sigma arguments.
random.Generator.standard_normal: which should be used for new code.
Notes
-----
For random samples from the normal distribution with mean ``mu`` and
standard deviation ``sigma``, use::
sigma * np.random.randn(...) + mu
Examples
--------
>>> np.random.randn()
2.1923875335537315 # random
Two-by-four array of samples from the normal distribution with
mean 3 and standard deviation 2.5:
>>> 3 + 2.5 * np.random.randn(2, 4)
array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
[ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
random(...) method of numpy.random.mtrand.RandomState instance
random(size=None)
Return random floats in the half-open interval [0.0, 1.0). Alias for
`random_sample` to ease forward-porting to the new random API.
random_integers(...) method of numpy.random.mtrand.RandomState instance
random_integers(low, high=None, size=None)
Random integers of type `np.int_` between `low` and `high`, inclusive.
Return random integers of type `np.int_` from the "discrete uniform"
distribution in the closed interval [`low`, `high`]. If `high` is
None (the default), then results are from [1, `low`]. The `np.int_`
type translates to the C long integer type and its precision
is platform dependent.
This function has been deprecated. Use randint instead.
.. deprecated:: 1.11.0
Parameters
----------
low : int
Lowest (signed) integer to be drawn from the distribution (unless
``high=None``, in which case this parameter is the *highest* such
integer).
high : int, optional
If provided, the largest (signed) integer to be drawn from the
distribution (see above for behavior if ``high=None``).
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
Returns
-------
out : int or ndarray of ints
`size`-shaped array of random integers from the appropriate
distribution, or a single such random int if `size` not provided.
See Also
--------
randint : Similar to `random_integers`, only for the half-open
interval [`low`, `high`), and 0 is the lowest value if `high` is
omitted.
Notes
-----
To sample from N evenly spaced floating-point numbers between a and b,
use::
a + (b - a) * (np.random.random_integers(N) - 1) / (N - 1.)
Examples
--------
>>> np.random.random_integers(5)
4 # random
>>> type(np.random.random_integers(5))
<class 'numpy.int64'>
>>> np.random.random_integers(5, size=(3,2))
array([[5, 4], # random
[3, 3],
[4, 5]])
Choose five random numbers from the set of five evenly-spaced
numbers between 0 and 2.5, inclusive (*i.e.*, from the set
:math:`{0, 5/8, 10/8, 15/8, 20/8}`):
>>> 2.5 * (np.random.random_integers(5, size=(5,)) - 1) / 4.
array([ 0.625, 1.25 , 0.625, 0.625, 2.5 ]) # random
Roll two six sided dice 1000 times and sum the results:
>>> d1 = np.random.random_integers(1, 6, 1000)
>>> d2 = np.random.random_integers(1, 6, 1000)
>>> dsums = d1 + d2
Display results as a histogram:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(dsums, 11, density=True)
>>> plt.show()
random_sample(...) method of numpy.random.mtrand.RandomState instance
random_sample(size=None)
Return random floats in the half-open interval [0.0, 1.0).
Results are from the "continuous uniform" distribution over the
stated interval. To sample :math:`Unif[a, b), b > a` multiply
the output of `random_sample` by `(b-a)` and add `a`::
(b - a) * random_sample() + a
.. note::
New code should use the `~numpy.random.Generator.random`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
Returns
-------
out : float or ndarray of floats
Array of random floats of shape `size` (unless ``size=None``, in which
case a single float is returned).
See Also
--------
random.Generator.random: which should be used for new code.
Examples
--------
>>> np.random.random_sample()
0.47108547995356098 # random
>>> type(np.random.random_sample())
<class 'float'>
>>> np.random.random_sample((5,))
array([ 0.30220482, 0.86820401, 0.1654503 , 0.11659149, 0.54323428]) # random
Three-by-two array of random numbers from [-5, 0):
>>> 5 * np.random.random_sample((3, 2)) - 5
array([[-3.99149989, -0.52338984], # random
[-2.99091858, -0.79479508],
[-1.23204345, -1.75224494]])
ranf(...)
This is an alias of `random_sample`. See `random_sample` for the complete
documentation.
rayleigh(...) method of numpy.random.mtrand.RandomState instance
rayleigh(scale=1.0, size=None)
Draw samples from a Rayleigh distribution.
The :math:`\chi` and Weibull distributions are generalizations of the
Rayleigh.
.. note::
New code should use the `~numpy.random.Generator.rayleigh`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
scale : float or array_like of floats, optional
Scale, also equals the mode. Must be non-negative. Default is 1.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``scale`` is a scalar. Otherwise,
``np.array(scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Rayleigh distribution.
See Also
--------
random.Generator.rayleigh: which should be used for new code.
Notes
-----
The probability density function for the Rayleigh distribution is
.. math:: P(x;scale) = \frac{x}{scale^2}e^{\frac{-x^2}{2 \cdotp scale^2}}
The Rayleigh distribution would arise, for example, if the East
and North components of the wind velocity had identical zero-mean
Gaussian distributions. Then the wind speed would have a Rayleigh
distribution.
References
----------
.. [1] Brighton Webs Ltd., "Rayleigh Distribution,"
https://web.archive.org/web/20090514091424/http://brighton-webs.co.uk:80/distributions/rayleigh.asp
.. [2] Wikipedia, "Rayleigh distribution"
https://en.wikipedia.org/wiki/Rayleigh_distribution
Examples
--------
Draw values from the distribution and plot the histogram
>>> from matplotlib.pyplot import hist
>>> values = hist(np.random.rayleigh(3, 100000), bins=200, density=True)
Wave heights tend to follow a Rayleigh distribution. If the mean wave
height is 1 meter, what fraction of waves are likely to be larger than 3
meters?
>>> meanvalue = 1
>>> modevalue = np.sqrt(2 / np.pi) * meanvalue
>>> s = np.random.rayleigh(modevalue, 1000000)
The percentage of waves larger than 3 meters is:
>>> 100.*sum(s>3)/1000000.
0.087300000000000003 # random
sample(...)
This is an alias of `random_sample`. See `random_sample` for the complete
documentation.
seed(...)
seed(seed=None)
Reseed the singleton RandomState instance.
Notes
-----
This is a convenience, legacy function that exists to support
older code that uses the singleton RandomState. Best practice
is to use a dedicated ``Generator`` instance rather than
the random variate generation methods exposed directly in
the random module.
See Also
--------
numpy.random.Generator
set_state(...) method of numpy.random.mtrand.RandomState instance
set_state(state)
Set the internal state of the generator from a tuple.
For use if one has reason to manually (re-)set the internal state of
the bit generator used by the RandomState instance. By default,
RandomState uses the "Mersenne Twister"[1]_ pseudo-random number
generating algorithm.
Parameters
----------
state : {tuple(str, ndarray of 624 uints, int, int, float), dict}
The `state` tuple has the following items:
1. the string 'MT19937', specifying the Mersenne Twister algorithm.
2. a 1-D array of 624 unsigned integers ``keys``.
3. an integer ``pos``.
4. an integer ``has_gauss``.
5. a float ``cached_gaussian``.
If state is a dictionary, it is directly set using the BitGenerators
`state` property.
Returns
-------
out : None
Returns 'None' on success.
See Also
--------
get_state
Notes
-----
`set_state` and `get_state` are not needed to work with any of the
random distributions in NumPy. If the internal state is manually altered,
the user should know exactly what he/she is doing.
For backwards compatibility, the form (str, array of 624 uints, int) is
also accepted although it is missing some information about the cached
Gaussian value: ``state = ('MT19937', keys, pos)``.
References
----------
.. [1] M. Matsumoto and T. Nishimura, "Mersenne Twister: A
623-dimensionally equidistributed uniform pseudorandom number
generator," *ACM Trans. on Modeling and Computer Simulation*,
Vol. 8, No. 1, pp. 3-30, Jan. 1998.
shuffle(...) method of numpy.random.mtrand.RandomState instance
shuffle(x)
Modify a sequence in-place by shuffling its contents.
This function only shuffles the array along the first axis of a
multi-dimensional array. The order of sub-arrays is changed but
their contents remains the same.
.. note::
New code should use the `~numpy.random.Generator.shuffle`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
x : ndarray or MutableSequence
The array, list or mutable sequence to be shuffled.
Returns
-------
None
See Also
--------
random.Generator.shuffle: which should be used for new code.
Examples
--------
>>> arr = np.arange(10)
>>> np.random.shuffle(arr)
>>> arr
[1 7 5 2 9 4 3 6 0 8] # random
Multi-dimensional arrays are only shuffled along the first axis:
>>> arr = np.arange(9).reshape((3, 3))
>>> np.random.shuffle(arr)
>>> arr
array([[3, 4, 5], # random
[6, 7, 8],
[0, 1, 2]])
standard_cauchy(...) method of numpy.random.mtrand.RandomState instance
standard_cauchy(size=None)
Draw samples from a standard Cauchy distribution with mode = 0.
Also known as the Lorentz distribution.
.. note::
New code should use the
`~numpy.random.Generator.standard_cauchy`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
Returns
-------
samples : ndarray or scalar
The drawn samples.
See Also
--------
random.Generator.standard_cauchy: which should be used for new code.
Notes
-----
The probability density function for the full Cauchy distribution is
.. math:: P(x; x_0, \gamma) = \frac{1}{\pi \gamma \bigl[ 1+
(\frac{x-x_0}{\gamma})^2 \bigr] }
and the Standard Cauchy distribution just sets :math:`x_0=0` and
:math:`\gamma=1`
The Cauchy distribution arises in the solution to the driven harmonic
oscillator problem, and also describes spectral line broadening. It
also describes the distribution of values at which a line tilted at
a random angle will cut the x axis.
When studying hypothesis tests that assume normality, seeing how the
tests perform on data from a Cauchy distribution is a good indicator of
their sensitivity to a heavy-tailed distribution, since the Cauchy looks
very much like a Gaussian distribution, but with heavier tails.
References
----------
.. [1] NIST/SEMATECH e-Handbook of Statistical Methods, "Cauchy
Distribution",
https://www.itl.nist.gov/div898/handbook/eda/section3/eda3663.htm
.. [2] Weisstein, Eric W. "Cauchy Distribution." From MathWorld--A
Wolfram Web Resource.
http://mathworld.wolfram.com/CauchyDistribution.html
.. [3] Wikipedia, "Cauchy distribution"
https://en.wikipedia.org/wiki/Cauchy_distribution
Examples
--------
Draw samples and plot the distribution:
>>> import matplotlib.pyplot as plt
>>> s = np.random.standard_cauchy(1000000)
>>> s = s[(s>-25) & (s<25)] # truncate distribution so it plots well
>>> plt.hist(s, bins=100)
>>> plt.show()
standard_exponential(...) method of numpy.random.mtrand.RandomState instance
standard_exponential(size=None)
Draw samples from the standard exponential distribution.
`standard_exponential` is identical to the exponential distribution
with a scale parameter of 1.
.. note::
New code should use the
`~numpy.random.Generator.standard_exponential`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
Returns
-------
out : float or ndarray
Drawn samples.
See Also
--------
random.Generator.standard_exponential: which should be used for new code.
Examples
--------
Output a 3x8000 array:
>>> n = np.random.standard_exponential((3, 8000))
standard_gamma(...) method of numpy.random.mtrand.RandomState instance
standard_gamma(shape, size=None)
Draw samples from a standard Gamma distribution.
Samples are drawn from a Gamma distribution with specified parameters,
shape (sometimes designated "k") and scale=1.
.. note::
New code should use the
`~numpy.random.Generator.standard_gamma`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
shape : float or array_like of floats
Parameter, must be non-negative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``shape`` is a scalar. Otherwise,
``np.array(shape).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized standard gamma distribution.
See Also
--------
scipy.stats.gamma : probability density function, distribution or
cumulative density function, etc.
random.Generator.standard_gamma: which should be used for new code.
Notes
-----
The probability density for the Gamma distribution is
.. math:: p(x) = x^{k-1}\frac{e^{-x/\theta}}{\theta^k\Gamma(k)},
where :math:`k` is the shape and :math:`\theta` the scale,
and :math:`\Gamma` is the Gamma function.
The Gamma distribution is often used to model the times to failure of
electronic components, and arises naturally in processes for which the
waiting times between Poisson distributed events are relevant.
References
----------
.. [1] Weisstein, Eric W. "Gamma Distribution." From MathWorld--A
Wolfram Web Resource.
http://mathworld.wolfram.com/GammaDistribution.html
.. [2] Wikipedia, "Gamma distribution",
https://en.wikipedia.org/wiki/Gamma_distribution
Examples
--------
Draw samples from the distribution:
>>> shape, scale = 2., 1. # mean and width
>>> s = np.random.standard_gamma(shape, 1000000)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> import scipy.special as sps # doctest: +SKIP
>>> count, bins, ignored = plt.hist(s, 50, density=True)
>>> y = bins**(shape-1) * ((np.exp(-bins/scale))/ # doctest: +SKIP
... (sps.gamma(shape) * scale**shape))
>>> plt.plot(bins, y, linewidth=2, color='r') # doctest: +SKIP
>>> plt.show()
standard_normal(...) method of numpy.random.mtrand.RandomState instance
standard_normal(size=None)
Draw samples from a standard Normal distribution (mean=0, stdev=1).
.. note::
New code should use the
`~numpy.random.Generator.standard_normal`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. Default is None, in which case a
single value is returned.
Returns
-------
out : float or ndarray
A floating-point array of shape ``size`` of drawn samples, or a
single sample if ``size`` was not specified.
See Also
--------
normal :
Equivalent function with additional ``loc`` and ``scale`` arguments
for setting the mean and standard deviation.
random.Generator.standard_normal: which should be used for new code.
Notes
-----
For random samples from the normal distribution with mean ``mu`` and
standard deviation ``sigma``, use one of::
mu + sigma * np.random.standard_normal(size=...)
np.random.normal(mu, sigma, size=...)
Examples
--------
>>> np.random.standard_normal()
2.1923875335537315 #random
>>> s = np.random.standard_normal(8000)
>>> s
array([ 0.6888893 , 0.78096262, -0.89086505, ..., 0.49876311, # random
-0.38672696, -0.4685006 ]) # random
>>> s.shape
(8000,)
>>> s = np.random.standard_normal(size=(3, 4, 2))
>>> s.shape
(3, 4, 2)
Two-by-four array of samples from the normal distribution with
mean 3 and standard deviation 2.5:
>>> 3 + 2.5 * np.random.standard_normal(size=(2, 4))
array([[-4.49401501, 4.00950034, -1.81814867, 7.29718677], # random
[ 0.39924804, 4.68456316, 4.99394529, 4.84057254]]) # random
standard_t(...) method of numpy.random.mtrand.RandomState instance
standard_t(df, size=None)
Draw samples from a standard Student's t distribution with `df` degrees
of freedom.
A special case of the hyperbolic distribution. As `df` gets
large, the result resembles that of the standard normal
distribution (`standard_normal`).
.. note::
New code should use the `~numpy.random.Generator.standard_t`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
df : float or array_like of floats
Degrees of freedom, must be > 0.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``df`` is a scalar. Otherwise,
``np.array(df).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized standard Student's t distribution.
See Also
--------
random.Generator.standard_t: which should be used for new code.
Notes
-----
The probability density function for the t distribution is
.. math:: P(x, df) = \frac{\Gamma(\frac{df+1}{2})}{\sqrt{\pi df}
\Gamma(\frac{df}{2})}\Bigl( 1+\frac{x^2}{df} \Bigr)^{-(df+1)/2}
The t test is based on an assumption that the data come from a
Normal distribution. The t test provides a way to test whether
the sample mean (that is the mean calculated from the data) is
a good estimate of the true mean.
The derivation of the t-distribution was first published in
1908 by William Gosset while working for the Guinness Brewery
in Dublin. Due to proprietary issues, he had to publish under
a pseudonym, and so he used the name Student.
References
----------
.. [1] Dalgaard, Peter, "Introductory Statistics With R",
Springer, 2002.
.. [2] Wikipedia, "Student's t-distribution"
https://en.wikipedia.org/wiki/Student's_t-distribution
Examples
--------
From Dalgaard page 83 [1]_, suppose the daily energy intake for 11
women in kilojoules (kJ) is:
>>> intake = np.array([5260., 5470, 5640, 6180, 6390, 6515, 6805, 7515, \
... 7515, 8230, 8770])
Does their energy intake deviate systematically from the recommended
value of 7725 kJ? Our null hypothesis will be the absence of deviation,
and the alternate hypothesis will be the presence of an effect that could be
either positive or negative, hence making our test 2-tailed.
Because we are estimating the mean and we have N=11 values in our sample,
we have N-1=10 degrees of freedom. We set our significance level to 95% and
compute the t statistic using the empirical mean and empirical standard
deviation of our intake. We use a ddof of 1 to base the computation of our
empirical standard deviation on an unbiased estimate of the variance (note:
the final estimate is not unbiased due to the concave nature of the square
root).
>>> np.mean(intake)
6753.636363636364
>>> intake.std(ddof=1)
1142.1232221373727
>>> t = (np.mean(intake)-7725)/(intake.std(ddof=1)/np.sqrt(len(intake)))
>>> t
-2.8207540608310198
We draw 1000000 samples from Student's t distribution with the adequate
degrees of freedom.
>>> import matplotlib.pyplot as plt
>>> s = np.random.standard_t(10, size=1000000)
>>> h = plt.hist(s, bins=100, density=True)
Does our t statistic land in one of the two critical regions found at
both tails of the distribution?
>>> np.sum(np.abs(t) < np.abs(s)) / float(len(s))
0.018318 #random < 0.05, statistic is in critical region
The probability value for this 2-tailed test is about 1.83%, which is
lower than the 5% pre-determined significance threshold.
Therefore, the probability of observing values as extreme as our intake
conditionally on the null hypothesis being true is too low, and we reject
the null hypothesis of no deviation.
triangular(...) method of numpy.random.mtrand.RandomState instance
triangular(left, mode, right, size=None)
Draw samples from the triangular distribution over the
interval ``[left, right]``.
The triangular distribution is a continuous probability
distribution with lower limit left, peak at mode, and upper
limit right. Unlike the other distributions, these parameters
directly define the shape of the pdf.
.. note::
New code should use the `~numpy.random.Generator.triangular`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
left : float or array_like of floats
Lower limit.
mode : float or array_like of floats
The value where the peak of the distribution occurs.
The value must fulfill the condition ``left <= mode <= right``.
right : float or array_like of floats
Upper limit, must be larger than `left`.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``left``, ``mode``, and ``right``
are all scalars. Otherwise, ``np.broadcast(left, mode, right).size``
samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized triangular distribution.
See Also
--------
random.Generator.triangular: which should be used for new code.
Notes
-----
The probability density function for the triangular distribution is
.. math:: P(x;l, m, r) = \begin{cases}
\frac{2(x-l)}{(r-l)(m-l)}& \text{for $l \leq x \leq m$},\\
\frac{2(r-x)}{(r-l)(r-m)}& \text{for $m \leq x \leq r$},\\
0& \text{otherwise}.
\end{cases}
The triangular distribution is often used in ill-defined
problems where the underlying distribution is not known, but
some knowledge of the limits and mode exists. Often it is used
in simulations.
References
----------
.. [1] Wikipedia, "Triangular distribution"
https://en.wikipedia.org/wiki/Triangular_distribution
Examples
--------
Draw values from the distribution and plot the histogram:
>>> import matplotlib.pyplot as plt
>>> h = plt.hist(np.random.triangular(-3, 0, 8, 100000), bins=200,
... density=True)
>>> plt.show()
uniform(...) method of numpy.random.mtrand.RandomState instance
uniform(low=0.0, high=1.0, size=None)
Draw samples from a uniform distribution.
Samples are uniformly distributed over the half-open interval
``[low, high)`` (includes low, but excludes high). In other words,
any value within the given interval is equally likely to be drawn
by `uniform`.
.. note::
New code should use the `~numpy.random.Generator.uniform`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
low : float or array_like of floats, optional
Lower boundary of the output interval. All values generated will be
greater than or equal to low. The default value is 0.
high : float or array_like of floats
Upper boundary of the output interval. All values generated will be
less than or equal to high. The high limit may be included in the
returned array of floats due to floating-point rounding in the
equation ``low + (high-low) * random_sample()``. The default value
is 1.0.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``low`` and ``high`` are both scalars.
Otherwise, ``np.broadcast(low, high).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized uniform distribution.
See Also
--------
randint : Discrete uniform distribution, yielding integers.
random_integers : Discrete uniform distribution over the closed
interval ``[low, high]``.
random_sample : Floats uniformly distributed over ``[0, 1)``.
random : Alias for `random_sample`.
rand : Convenience function that accepts dimensions as input, e.g.,
``rand(2,2)`` would generate a 2-by-2 array of floats,
uniformly distributed over ``[0, 1)``.
random.Generator.uniform: which should be used for new code.
Notes
-----
The probability density function of the uniform distribution is
.. math:: p(x) = \frac{1}{b - a}
anywhere within the interval ``[a, b)``, and zero elsewhere.
When ``high`` == ``low``, values of ``low`` will be returned.
If ``high`` < ``low``, the results are officially undefined
and may eventually raise an error, i.e. do not rely on this
function to behave when passed arguments satisfying that
inequality condition. The ``high`` limit may be included in the
returned array of floats due to floating-point rounding in the
equation ``low + (high-low) * random_sample()``. For example:
>>> x = np.float32(5*0.99999999)
>>> x
5.0
Examples
--------
Draw samples from the distribution:
>>> s = np.random.uniform(-1,0,1000)
All values are within the given interval:
>>> np.all(s >= -1)
True
>>> np.all(s < 0)
True
Display the histogram of the samples, along with the
probability density function:
>>> import matplotlib.pyplot as plt
>>> count, bins, ignored = plt.hist(s, 15, density=True)
>>> plt.plot(bins, np.ones_like(bins), linewidth=2, color='r')
>>> plt.show()
vonmises(...) method of numpy.random.mtrand.RandomState instance
vonmises(mu, kappa, size=None)
Draw samples from a von Mises distribution.
Samples are drawn from a von Mises distribution with specified mode
(mu) and dispersion (kappa), on the interval [-pi, pi].
The von Mises distribution (also known as the circular normal
distribution) is a continuous probability distribution on the unit
circle. It may be thought of as the circular analogue of the normal
distribution.
.. note::
New code should use the `~numpy.random.Generator.vonmises`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
mu : float or array_like of floats
Mode ("center") of the distribution.
kappa : float or array_like of floats
Dispersion of the distribution, has to be >=0.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``mu`` and ``kappa`` are both scalars.
Otherwise, ``np.broadcast(mu, kappa).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized von Mises distribution.
See Also
--------
scipy.stats.vonmises : probability density function, distribution, or
cumulative density function, etc.
random.Generator.vonmises: which should be used for new code.
Notes
-----
The probability density for the von Mises distribution is
.. math:: p(x) = \frac{e^{\kappa cos(x-\mu)}}{2\pi I_0(\kappa)},
where :math:`\mu` is the mode and :math:`\kappa` the dispersion,
and :math:`I_0(\kappa)` is the modified Bessel function of order 0.
The von Mises is named for Richard Edler von Mises, who was born in
Austria-Hungary, in what is now the Ukraine. He fled to the United
States in 1939 and became a professor at Harvard. He worked in
probability theory, aerodynamics, fluid mechanics, and philosophy of
science.
References
----------
.. [1] Abramowitz, M. and Stegun, I. A. (Eds.). "Handbook of
Mathematical Functions with Formulas, Graphs, and Mathematical
Tables, 9th printing," New York: Dover, 1972.
.. [2] von Mises, R., "Mathematical Theory of Probability
and Statistics", New York: Academic Press, 1964.
Examples
--------
Draw samples from the distribution:
>>> mu, kappa = 0.0, 4.0 # mean and dispersion
>>> s = np.random.vonmises(mu, kappa, 1000)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> from scipy.special import i0 # doctest: +SKIP
>>> plt.hist(s, 50, density=True)
>>> x = np.linspace(-np.pi, np.pi, num=51)
>>> y = np.exp(kappa*np.cos(x-mu))/(2*np.pi*i0(kappa)) # doctest: +SKIP
>>> plt.plot(x, y, linewidth=2, color='r') # doctest: +SKIP
>>> plt.show()
wald(...) method of numpy.random.mtrand.RandomState instance
wald(mean, scale, size=None)
Draw samples from a Wald, or inverse Gaussian, distribution.
As the scale approaches infinity, the distribution becomes more like a
Gaussian. Some references claim that the Wald is an inverse Gaussian
with mean equal to 1, but this is by no means universal.
The inverse Gaussian distribution was first studied in relationship to
Brownian motion. In 1956 M.C.K. Tweedie used the name inverse Gaussian
because there is an inverse relationship between the time to cover a
unit distance and distance covered in unit time.
.. note::
New code should use the `~numpy.random.Generator.wald`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
mean : float or array_like of floats
Distribution mean, must be > 0.
scale : float or array_like of floats
Scale parameter, must be > 0.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``mean`` and ``scale`` are both scalars.
Otherwise, ``np.broadcast(mean, scale).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Wald distribution.
See Also
--------
random.Generator.wald: which should be used for new code.
Notes
-----
The probability density function for the Wald distribution is
.. math:: P(x;mean,scale) = \sqrt{\frac{scale}{2\pi x^3}}e^
\frac{-scale(x-mean)^2}{2\cdotp mean^2x}
As noted above the inverse Gaussian distribution first arise
from attempts to model Brownian motion. It is also a
competitor to the Weibull for use in reliability modeling and
modeling stock returns and interest rate processes.
References
----------
.. [1] Brighton Webs Ltd., Wald Distribution,
https://web.archive.org/web/20090423014010/http://www.brighton-webs.co.uk:80/distributions/wald.asp
.. [2] Chhikara, Raj S., and Folks, J. Leroy, "The Inverse Gaussian
Distribution: Theory : Methodology, and Applications", CRC Press,
1988.
.. [3] Wikipedia, "Inverse Gaussian distribution"
https://en.wikipedia.org/wiki/Inverse_Gaussian_distribution
Examples
--------
Draw values from the distribution and plot the histogram:
>>> import matplotlib.pyplot as plt
>>> h = plt.hist(np.random.wald(3, 2, 100000), bins=200, density=True)
>>> plt.show()
weibull(...) method of numpy.random.mtrand.RandomState instance
weibull(a, size=None)
Draw samples from a Weibull distribution.
Draw samples from a 1-parameter Weibull distribution with the given
shape parameter `a`.
.. math:: X = (-ln(U))^{1/a}
Here, U is drawn from the uniform distribution over (0,1].
The more common 2-parameter Weibull, including a scale parameter
:math:`\lambda` is just :math:`X = \lambda(-ln(U))^{1/a}`.
.. note::
New code should use the `~numpy.random.Generator.weibull`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
a : float or array_like of floats
Shape parameter of the distribution. Must be nonnegative.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``a`` is a scalar. Otherwise,
``np.array(a).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Weibull distribution.
See Also
--------
scipy.stats.weibull_max
scipy.stats.weibull_min
scipy.stats.genextreme
gumbel
random.Generator.weibull: which should be used for new code.
Notes
-----
The Weibull (or Type III asymptotic extreme value distribution
for smallest values, SEV Type III, or Rosin-Rammler
distribution) is one of a class of Generalized Extreme Value
(GEV) distributions used in modeling extreme value problems.
This class includes the Gumbel and Frechet distributions.
The probability density for the Weibull distribution is
.. math:: p(x) = \frac{a}
{\lambda}(\frac{x}{\lambda})^{a-1}e^{-(x/\lambda)^a},
where :math:`a` is the shape and :math:`\lambda` the scale.
The function has its peak (the mode) at
:math:`\lambda(\frac{a-1}{a})^{1/a}`.
When ``a = 1``, the Weibull distribution reduces to the exponential
distribution.
References
----------
.. [1] Waloddi Weibull, Royal Technical University, Stockholm,
1939 "A Statistical Theory Of The Strength Of Materials",
Ingeniorsvetenskapsakademiens Handlingar Nr 151, 1939,
Generalstabens Litografiska Anstalts Forlag, Stockholm.
.. [2] Waloddi Weibull, "A Statistical Distribution Function of
Wide Applicability", Journal Of Applied Mechanics ASME Paper
1951.
.. [3] Wikipedia, "Weibull distribution",
https://en.wikipedia.org/wiki/Weibull_distribution
Examples
--------
Draw samples from the distribution:
>>> a = 5. # shape
>>> s = np.random.weibull(a, 1000)
Display the histogram of the samples, along with
the probability density function:
>>> import matplotlib.pyplot as plt
>>> x = np.arange(1,100.)/50.
>>> def weib(x,n,a):
... return (a / n) * (x / n)**(a - 1) * np.exp(-(x / n)**a)
>>> count, bins, ignored = plt.hist(np.random.weibull(5.,1000))
>>> x = np.arange(1,100.)/50.
>>> scale = count.max()/weib(x, 1., 5.).max()
>>> plt.plot(x, weib(x, 1., 5.)*scale)
>>> plt.show()
zipf(...) method of numpy.random.mtrand.RandomState instance
zipf(a, size=None)
Draw samples from a Zipf distribution.
Samples are drawn from a Zipf distribution with specified parameter
`a` > 1.
The Zipf distribution (also known as the zeta distribution) is a
discrete probability distribution that satisfies Zipf's law: the
frequency of an item is inversely proportional to its rank in a
frequency table.
.. note::
New code should use the `~numpy.random.Generator.zipf`
method of a `~numpy.random.Generator` instance instead;
please see the :ref:`random-quick-start`.
Parameters
----------
a : float or array_like of floats
Distribution parameter. Must be greater than 1.
size : int or tuple of ints, optional
Output shape. If the given shape is, e.g., ``(m, n, k)``, then
``m * n * k`` samples are drawn. If size is ``None`` (default),
a single value is returned if ``a`` is a scalar. Otherwise,
``np.array(a).size`` samples are drawn.
Returns
-------
out : ndarray or scalar
Drawn samples from the parameterized Zipf distribution.
See Also
--------
scipy.stats.zipf : probability density function, distribution, or
cumulative density function, etc.
random.Generator.zipf: which should be used for new code.
Notes
-----
The probability density for the Zipf distribution is
.. math:: p(k) = \frac{k^{-a}}{\zeta(a)},
for integers :math:`k \geq 1`, where :math:`\zeta` is the Riemann Zeta
function.
It is named for the American linguist George Kingsley Zipf, who noted
that the frequency of any word in a sample of a language is inversely
proportional to its rank in the frequency table.
References
----------
.. [1] Zipf, G. K., "Selected Studies of the Principle of Relative
Frequency in Language," Cambridge, MA: Harvard Univ. Press,
1932.
Examples
--------
Draw samples from the distribution:
>>> a = 4.0
>>> n = 20000
>>> s = np.random.zipf(a, n)
Display the histogram of the samples, along with
the expected histogram based on the probability
density function:
>>> import matplotlib.pyplot as plt
>>> from scipy.special import zeta # doctest: +SKIP
`bincount` provides a fast histogram for small integers.
>>> count = np.bincount(s)
>>> k = np.arange(1, s.max() + 1)
>>> plt.bar(k, count[1:], alpha=0.5, label='sample count')
>>> plt.plot(k, n*(k**-a)/zeta(a), 'k.-', alpha=0.5,
... label='expected count') # doctest: +SKIP
>>> plt.semilogy()
>>> plt.grid(alpha=0.4)
>>> plt.legend()
>>> plt.title(f'Zipf sample, a={a}, size={n}')
>>> plt.show()
DATA
__all__ = ['beta', 'binomial', 'bytes', 'chisquare', 'choice', 'dirich...
FILE
/opt/anaconda3/lib/python3.12/site-packages/numpy/random/__init__.py
Rejection Sampling#
What can we do when transformations from uniform distribution is not available?
Let us find a proposal distribution \(q(x)\) for which samples are easily generated and covers the target distribution as \(p(x)\le cq(x)\) with a scaling constant \(c>0\).
Then take a sample from \(q(x)\) and accept it with probability \(\frac{p(x)}{cq(x)}\).
Gamma distribution#
Gamma distribution is an extension of exponential distribution defined as $\( p(x; k, \theta) = \frac{1}{\Gamma(k)\theta^k}x^{k-1}e^{-\frac{x}{\theta}} \)\( with the shape parameter \)k>0\( and the scaling parameter \)\theta$.
\(\Gamma(k)\) is the gamma function, which is a generalization of factorial, \(\Gamma(k)=(k-1)!\) for an integer \(k\).
Let us generate samples from gamma distribution with integer \(k\) and \(\theta=1\).
def p_gamma(x, k=1):
"""gamma distribution with integer k"""
return x**(k-1)*np.exp(-x)/np.prod(range(1,k))
x = np.linspace(0, 10, 100)
for k in range(1, 6):
plt.plot(x, p_gamma(x, k), label='k={}'.format(k))
plt.xlabel('x'); plt.ylabel('p(x)'); plt.legend();
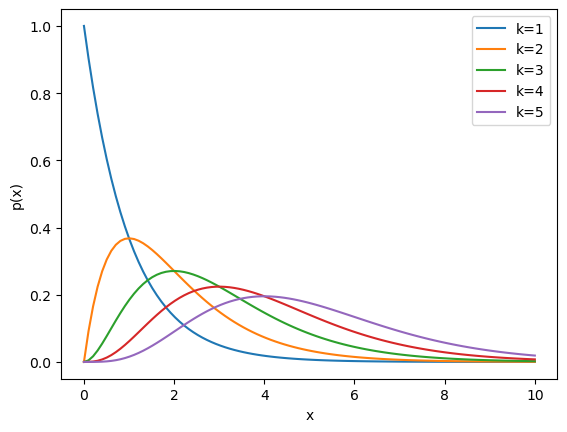
Consider the exponential distribution \(q(x;\mu)=\frac{1}{\mu}e^{-\frac{x}{\mu}}\) as the proposal distribution.
For
$\( \frac{p(x;k)}{q(x;\mu)} = \frac{\mu}{(k-1)!}x^{k-1}e^{-(1-\frac{1}{\mu})x}, \)\(
we set
\)\( \frac{d}{dx}\frac{p(x;k)}{q(x;\mu)} = 0 \)\(
and have
\)\( \left((k-1)x^{k-2}+\frac{1-\mu}{\mu}x^{k-1}\right)e^{\frac{1-\mu}{\mu}x} = 0, \)\(
\)\( x = \frac{\mu(k-1)}{\mu-1}, \)\(
where \)\frac{p(x;k)}{q(x;\mu)}\( takes the maximum
\)\( \frac{\mu^k}{(k-1)!}\left(\frac{k-1}{\mu-1}\right)^{k-1}e^{1-k}. \)\(
By taking \)\mu=k\(,
\)\( c=\frac{k^k}{(k-1)!}e^{1-k} \)\(
satisfies \)p(x)\le cq(x)$
k = 3
c = (k**k)/np.prod(range(1,k))*np.exp(1-k)
#print(c)
x = np.linspace(0, 10, 100)
plt.plot(x, p_gamma(x, k))
plt.plot(x, c*p_exp(x, k))
plt.xlabel('x'); plt.ylabel('p, q');
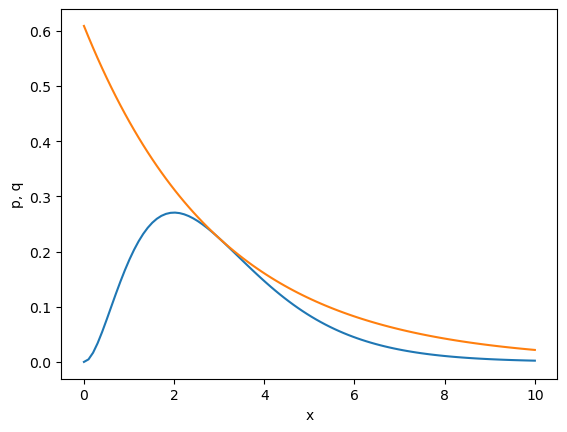
def x_gamma(n=10, k=1):
"""sample from gamma distribution by rejection sampling"""
c = (k**k)/np.prod(range(1,k))*np.exp(1-k)
xe = x_exp(n, k)
paccept = p_gamma(xe, k)/(c*p_exp(xe, k))
accept = np.random.random(n)<paccept
xg = xe[accept] # rejection sampling
#print("c =", c, "; acceptance rate =", len(xg)/n)
return xg
k = 3
xs = x_gamma(1000, k)
print('accept:', len(xs))
plt.hist(xs, bins=20, density=True)
plt.plot(x, p_gamma(x, k))
plt.xlabel('x'); plt.ylabel('p');
accept: 521
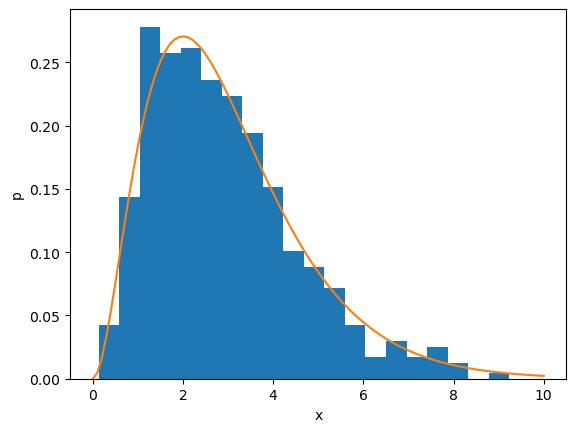
Importance Sampling#
One probelm of rejection sampling is that we may end up rejecting many of the samples when we cannot find a good proposal distribution.
When the aim is not just to take samples from \(p(x)\) but to take the expectation of a function \(h(x)\) with respect to the distribution \(p(x)\) $\( E_p[h(x)] = \int h(x)p(x)dx, \)$ we can use the ratio between the target and proposal distributions to better utilize the samples.
Mean and variance#
Let us consider taking the mean $\( \mu = E_p[x] = \int xp(x)dx \)\( and the variance \)\( \sigma^2 = E_p[(x-\mu)^2] = \int (x-\mu)^2p(x)dx \)\( for the gamma distribution, which are known to be \)k\theta\( and \)k\theta^2$, respectively.
def mv_exp(n=1, mu=1):
"""mean and variance of exponential distribution"""
x = x_exp(n, mu)
mean = np.mean(x)
var = np.var(x)
return (mean, var)
mv_exp(1000, 3)
(2.9947309041359773, 8.864967781536892)
def mv_gamma(n=1, k=1):
"""mean and variance of gamma distribution by rejection sampling"""
x = x_gamma(n, k) # by rejection sampling
mean = np.mean(x)
var = np.var(x)
return (mean, var)
mv_gamma(100, 3)
(3.024007319940854, 2.2140024594809584)
def mv_gamma_is(n=1, k=1):
"""mean and variance of gamma distribution by importance sampling"""
x = x_exp(n, k) # sample by exponential distribution
importance = p_gamma(x, k)/p_exp(x, k)
mean = np.dot(importance, x)/n
var = np.dot(importance, (x-mean)**2)/(n - 1)
return (mean, var)
mv_gamma_is(1000, 3)
(3.091573581019066, 2.9307231307804145)
Compare the variability of the estimate of the mean.
m = 100 # number of runs
n = 100 # number of samples
k = 3
means = np.zeros((m, 2))
for i in range(m):
means[i,0], var = mv_gamma(n, k)
means[i,1], var = mv_gamma_is(n, k)
print("RS: ", np.mean(means[:,0]), np.var(means[:,0]))
print("IS: ", np.mean(means[:,1]), np.var(means[:,1]))
RS: 2.9631283091320664 0.03914153346330196
IS: 2.9946973798115923 0.06491384051700812
Markov Chain Monte Carlo (MCMC)#
What if we don’t know the right proposal distribution? We can take a nearby point of a previous sample as a candidate sample and keep it if the candidate’s probability is high. The method is callel Markov Chain Monte Carlo (MCMC) method and used practically for sampling from unknow distributions in high dimensional space.
Metropolis Sampling#
A simple example of MCMC is Metropolis sampling, which requires only unnormalized propability \(\tilde{p}(x)\propto p(x)\) of samples for relative comparison.
A new candidate \(x^*\) is generated by a symmetric proposal distribution \(q(x^*|x_k)=q(x_k|x^*)\), such as a gaussian distribution, and acctepted with the probability of $\( p_\mbox{acc} = \min(1, \frac{p(x^*)}{p(x_k)}) \)$
def metropolis(p, x0, sig=0.1, m=1000):
"""metropolis: Metropolis sampling
p:unnormalized probability, x0:initial point,
sif:sd of proposal distribution, m:number of sampling"""
n = len(x0) # dimension
p0 = p(x0)
x = []
for i in range(m):
x1 = x0 + sig*np.random.randn(n)
p1 = p(x1)
pacc = min(1, p1/p0)
if np.random.rand()<pacc:
x.append(x1)
x0 = x1
p0 = p1
return np.array(x)
def croissant(x):
"""croissant-like distribution in 2D"""
return np.exp(-x[0]**2 - (x[1]-x[0]**2)**2)
r = 4 # plot rage
x = np.linspace(-r, r)
X, Y = np.meshgrid(x, x)
Z = croissant(np.array([X,Y]))
plt.contour(X, Y, Z);
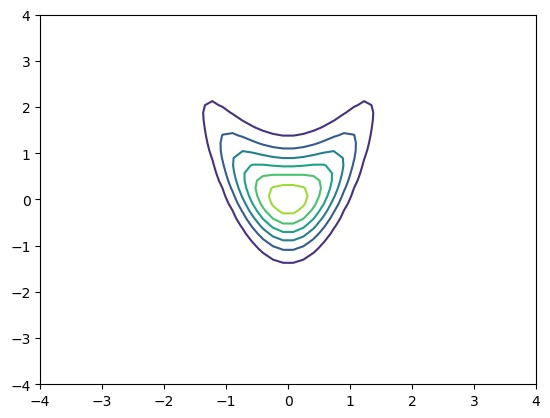
x = metropolis(croissant, [0,0])
s = len(x); print(s)
plt.contour(X, Y, Z)
plt.scatter(x[:,0], x[:,1], c=np.arange(s), marker='.');
941
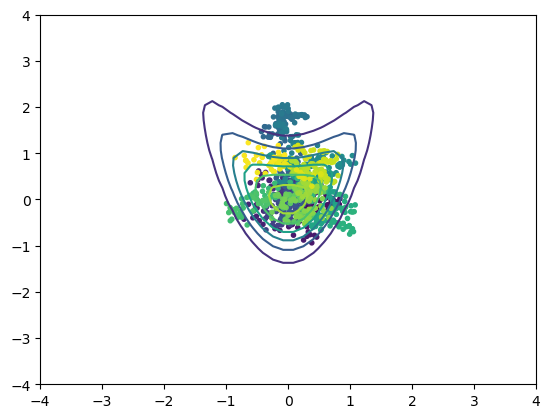
x = metropolis(croissant, [2,0], sig=0.1, m=1000)
s = len(x); print(s)
plt.contour(X, Y, Z)
plt.scatter(x[:,0], x[:,1], c=np.arange(s), marker='.');
931
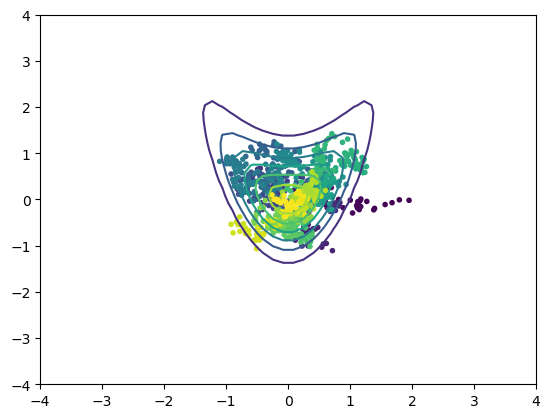