3D Visualization#
You can create a 3D axis by projection='3d'
option.
import numpy as np
import matplotlib.pyplot as plt
The magic command %matplotlib notebook
allows you to interactively change the viewpoint by clicking and dragging.
But for this static book, we use regular inline mode.
#%matplotlib notebook
%matplotlib inline
Lines and Points in 3D#
# spiral data
x = np.linspace(0, 20, 100)
y = x*np.sin(x)
z = x*np.cos(x)
# create a figure and 3D axes
fig = plt.figure()
ax = fig.add_subplot(projection='3d')
ax.plot(x, y, z)
[<mpl_toolkits.mplot3d.art3d.Line3D at 0x10d8d1640>]
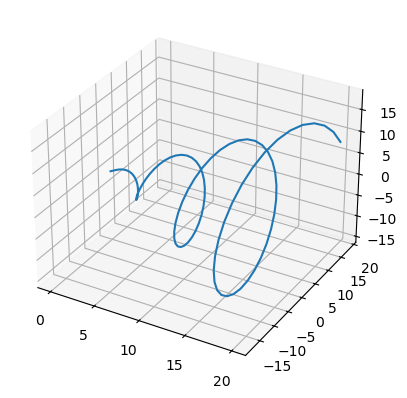
# You can make a figure and an axis in one line:
ax = plt.figure().add_subplot(projection='3d')
# scatter plot with x value mapped to color
ax.scatter(x, y, z, c=x)
<mpl_toolkits.mplot3d.art3d.Path3DCollection at 0x10d92fa40>
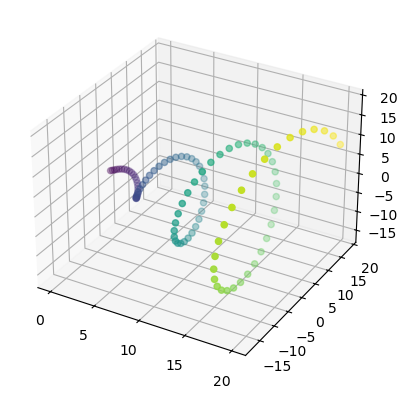
surface plot#
x = np.linspace(-5, 5, 25)
y = np.linspace(-5, 5, 25)
X, Y = np.meshgrid(x, y)
Z = X*Y
ax = plt.figure().add_subplot(projection='3d')
ax.plot_surface(X, Y, Z)
<mpl_toolkits.mplot3d.art3d.Poly3DCollection at 0x10d8069f0>
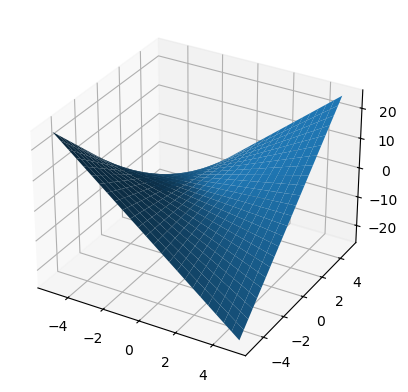
You can color the surface by the height.
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# map Z value to 'viridis' colormap
ax.plot_surface(X, Y, Z, cmap='viridis');
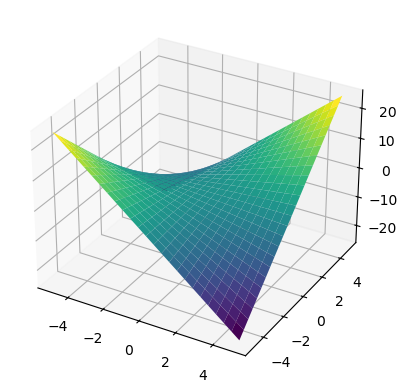
surface by wire frame#
# make a 3D axis in one line
ax = plt.figure().add_subplot(111, projection='3d')
# wireframe plot
ax.plot_wireframe(X, Y, Z)
<mpl_toolkits.mplot3d.art3d.Line3DCollection at 0x10dbaa4b0>
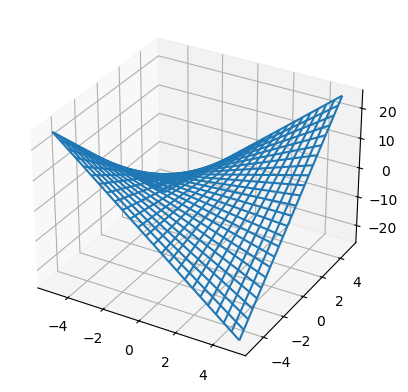
3D vector field by quiver( )
#
x = np.linspace(-5, 5, 10)
y = np.linspace(-5, 5, 10)
z = np.linspace(-5, 5, 10)
X, Y, Z = np.meshgrid(x, y, z)
#print(X)
# tornado
U = Y*Z # dx/dt
V = -X*Z # dy/dt
W = Z # dz/dt
ax = plt.figure().add_subplot(111, projection='3d')
ax.quiver(X, Y, Z, U, V, W, length=0.1)
<mpl_toolkits.mplot3d.art3d.Line3DCollection at 0x10dba95b0>
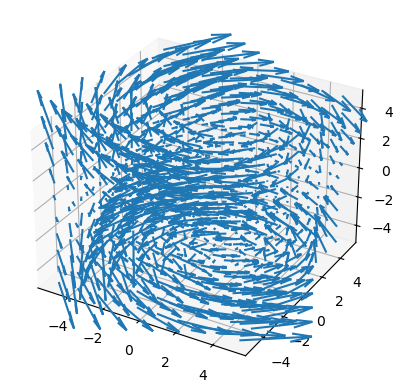
For more advanced 3D visualization, you may want to use a specialized library like mayavi
https://docs.enthought.com/mayavi/mayavi/