Collaborative Development: GitHub#
GitHub is currently the most popular cloud service for sharing software. It is free for open software.
This is a good platform for sharing programs, or in some cases text data and manuscripts, among collaborators. It is also helpful for a single-person project, for succession by a future member of your lab, for open access after publication, or for yourself after some time.
These are typical steps in contributing to a project in GitHub.
Join as a member of a repository.
Copy the existing files and see how they work.
Make a new branch and add or modify the codes.
After tesing locally, commit the new version.
Open a pull request for other members to test your revision.
Your pull request is merged into the master branch.
See “Hello World” in GitHub Guide for details (https://guides.github.com).
Cloning a repository#
If you just use a copy of a stable software, and not going to contribute your changes, just downloading a zip file is fine.
But if you would congribute to joint development, or catch up with updates, git clone
is the better way.
Cloning odes repository#
Let us try with a simple ODE simulator odes.py
on:
doya-oist/odes
To download a copy of the repository, run
git clone https://github.com/doya-oist/odes.git
This should create a folder odes
.
!git clone https://github.com/doya-oist/odes.git
fatal: destination path 'odes' already exists and is not an empty directory.
Move into the folder and test odesim.py
program.
%cd odes
/Users/doya/OIST Dropbox/kenji doya/Python/iSciComp/odes
%ls
LICENSE __pycache__/ odes.py vdp.py
README.md first.py second.py
!cat odes.py
# odes.py
# An Ordinary Differential Equation Simulator
# 2022 by Kenji Doya
import numpy as np
from scipy.integrate import odeint
import matplotlib.pyplot as plt
import importlib # import module from string
class odes():
"""An ODE Simulator"""
time = 0
trun = 10
dt = 0.1
def __init__(self, odename):
"""create a new ODE"""
print('Importing ODE:', odename)
self.ode = importlib.import_module( odename)
importlib.reload(self.ode) # for updated module
self.state = self.ode.initial_state
#self.reset()
plt.ion()
def reset(self):
"""reset the state"""
self.time = 0
self.state = self.ode.initial_state
plt.clf()
def simulate(self):
"""simulate the ODE"""
# +dt/2 to include time+trun
self.t = np.arange(self.time, self.time+self.trun+self.dt/2, self.dt)
self.y = odeint(self.ode.dynamics, self.state, self.t, args=(self.ode.parameters,))
# update the time and state
self.time = self.t[-1]
self.state = self.y[-1,:]
print("t=", self.time, "; state=", self.state)
def plot(self):
"""plot in time"""
plt.plot(self.t, self.y)
plt.xlabel('t'); plt.ylabel('y')
def run(self):
"""simulate the ODE"""
self.simulate()
self.plot()
From the console you can run interactively after reading the module as:
python -i odes.py
sim = odesim('first')
sim.run()
from odes import *
sim = odes('first')
Importing ODE: first
sim.run()
t= 10.0 ; state= [-0.36787947]
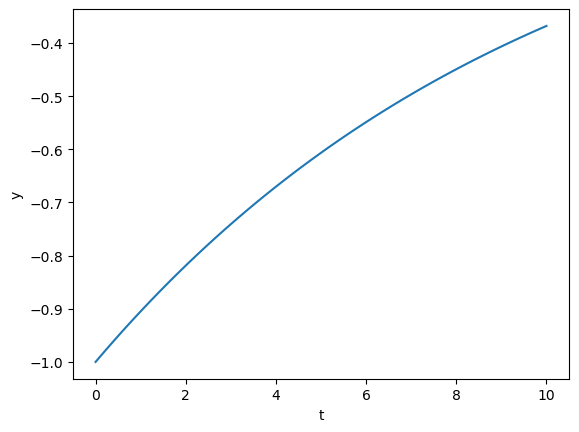
Your branch#
Now make your own branch, check it out, and add your own ODE module.
!git branch myname
!git checkout myname
fatal: a branch named 'myname' already exists
Already on 'myname'
Make a copy of a dynamics file first.py
or second.py
, implement your own ODE, and save with a new name, e.g. vdp.py
.
%%file vdp.py
# vdp.py
# van der Pol oscillator
# Dec. 2018 by Kenji Doya
import numpy as np
# Right-hand-side function of the ODE
def dynamics(y, t, mu=1.):
"""van der Pol oscillator:
d2y/dt2 = mu*(1 - y**2)*dy/dt - y"""
y1, y2 = y
return np.array([y2, mu*(1 - y1**2)*y2 - y1])
# Default parameters
parameters = 1.
# Default initial state
initial_state = [1, 0]
Overwriting vdp.py
%pwd
'/Users/doya/OIST Dropbox/kenji doya/Python/iSciComp/odes'
Run odes and confirm that your ODE runs appropriately.
sim = odes('vdp')
Importing ODE: vdp
sim.run()
t= 10.0 ; state= [-1.58203155 0.73418353]
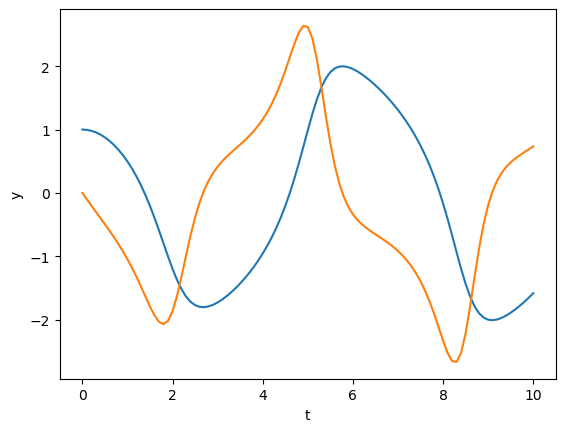
Then you can add and commit your change.
!git status
On branch myname
Untracked files:
(use "git add <file>..." to include in what will be committed)
__pycache__/
nothing added to commit but untracked files present (use "git add" to track)
!git add vdp.py
!git commit -m "adding my model vdp.py"
On branch myname
Untracked files:
(use "git add <file>..." to include in what will be committed)
__pycache__/
nothing added to commit but untracked files present (use "git add" to track)
!git log --graph --all
* commit c76d3ef44edd3033e1411f3f609747ddd2362eb1 (HEAD -> myname)
| Author: Kenji Doya <doya@oist.jp>
| Date: Mon Dec 16 14:54:04 2024 +0900
|
| adding my model vdp.py
|
* commit 670bad23cf98271d2017e8d9034ae3337bad3122 (origin/main, origin/HEAD, main)
| Author: Kenji Doya <doya@oist.jp>
| Date: Mon Dec 16 14:28:15 2024 +0900
|
| first set of files
|
* commit 0c1c8b1334ffe99caf36f502b5f400a1eeb84121
Author: Kenji Doya <doya@oist.jp>
Date: Mon Dec 16 12:42:22 2024 +0900
Initial commit
Now push your branch to GitHub repository by, e.g.
git push origin myname
!git push origin myname
Username for 'https://github.com':
^C
Check the status on GitHub: oist/ComputationalMethods2022
and make a pull request for the repository administrator to check your updates.
The administrator may reply back with a comment for revision or merge your change to the main branch.
Pulling updates#
While you are working on your local code, the codes on the origial repository may be updated. You may also want to check the branches other people have created.
You can use git pull
to reflect the changes in the GitHub to your local repository.
You can use git branch
to see what branches are there and git checkout
to try with the codes in other branches.
!git pull
!git branch
Optional) In addition to adding a new module, you are welcome to improve the main program odesim.py
itself. For example,
add other visualization like a phese plot.
fix any bugs or improve error handling.
add documentation.
…